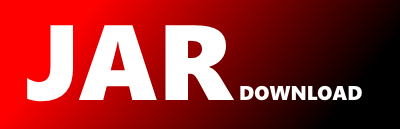
com.google.common.base.Predicates Maven / Gradle / Ivy
Show all versions of google-collect Show documentation
/*
* Copyright (C) 2007 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.common.base;
import static com.google.common.base.Preconditions.checkContentsNotNull;
import static com.google.common.base.Preconditions.checkNotNull;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Iterator;
/**
* Contains static methods for creating the standard set of {@code Predicate}
* objects.
*
* "Lispy, but good."
*
*
TODO: considering having these implement a {@code VisitablePredicate}
* interface which specifies an {@code accept(PredicateVisitor)} method.
*
* @author Kevin Bourrillion
*/
public final class Predicates {
private Predicates() {}
/**
* Returns a predicate that always evaluates to {@code true}.
*/
@SuppressWarnings("unchecked")
public static Predicate alwaysTrue() {
return (Predicate) AlwaysTruePredicate.INSTANCE;
}
/**
* Returns a predicate that always evaluates to {@code false}.
*/
@SuppressWarnings("unchecked")
public static Predicate alwaysFalse() {
return (Predicate) AlwaysFalsePredicate.INSTANCE;
}
/**
* Returns a predicate that evaluates to true if the object reference being
* tested is null.
*/
@SuppressWarnings("unchecked")
public static Predicate isNull() {
return (Predicate) IsNullPredicate.INSTANCE;
}
/**
* Returns a predicate that evaluates to true if the object reference being
* tested is not null.
*/
@SuppressWarnings("unchecked")
public static Predicate notNull() {
return (Predicate) NotNullPredicate.INSTANCE;
}
/**
* Returns a predicate that evaluates to true iff the given predicate
* evaluates to {@code false}.
*/
public static Predicate not(Predicate super T> predicate) {
return new NotPredicate(predicate);
}
/**
* Returns a predicate that evaluates to {@code true} iff each of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as the answer is
* determined. Does not defensively copy the iterable passed in, so future
* changes to it will alter the behavior of this predicate. If
* {@code components} is empty, the returned predicate will always evaluate to
* {@code true}.
*/
public static Predicate and(
Iterable extends Predicate super T>> components) {
return new AndPredicate(components);
}
/**
* Returns a predicate that evaluates to {@code true} iff each of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as the answer is
* determined. Does not defensively copy the array passed in, so future
* changes to it will alter the behavior of this predicate. If
* {@code components} is empty, the returned predicate will always evaluate to
* {@code true}.
*/
public static Predicate and(Predicate super T>... components) {
return and(Arrays.asList(components));
}
/**
* Returns a predicate that evaluates to {@code true} iff both of its
* components evaluate to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as the answer is
* determined.
*/
@SuppressWarnings("unchecked")
public static Predicate and(Predicate super T> first,
Predicate super T> second) {
return and(Arrays.> asList(first, second));
}
/**
* Returns a predicate that evaluates to {@code true} iff any one of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as the answer is
* determined. Does not defensively copy the iterable passed in, so future
* changes to it will alter the behavior of this predicate. If
* {@code components} is empty, the returned predicate will always evaluate to
* {@code false}.
*/
public static Predicate or(
Iterable extends Predicate super T>> components) {
return new OrPredicate(components);
}
/**
* Returns a predicate that evaluates to true iff any one of its components
* evaluates to true. The components are evaluated in order, and evaluation
* will be "short-circuited" as soon as the answer is determined. Does not
* defensively copy the array passed in, so future changes to it will alter
* the behavior of this predicate. If components is empty, the returned
* predicate will always evaluate to false.
*/
public static Predicate or(Predicate super T>... components) {
return or(Arrays.asList(components));
}
/**
* Returns a predicate that evaluates to {@code true} iff either of its
* components evaluates to {@code true}. The components are evaluated in
* order, and evaluation will be "short-circuited" as soon as the answer is
* determined.
*/
@SuppressWarnings("unchecked")
public static Predicate or(Predicate super T> first,
Predicate super T> second) {
return or(Arrays.> asList(first, second));
}
/**
* Returns a predicate that evaluates to {@code true} iff the object being
* tested {@code equals()} the given target or if both are null.
*
* TODO: Change signature to return Predicate<Object>
*/
public static Predicate isEqualTo(@Nullable T target) {
return (target == null)
? Predicates. isNull()
: new IsEqualToPredicate(target);
}
/**
* Returns a predicate that evaluates to {@code true} iff the object being
* tested refers to the same object as the given target.
*/
public static Predicate