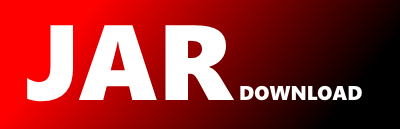
com.google.common.base.Suppliers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-collect Show documentation
Show all versions of google-collect Show documentation
Google Collections Library is a suite of new collections and collection-related goodness for Java 5.0
The newest version!
/*
* Copyright (C) 2007 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.common.base;
import java.io.Serializable;
/**
* Useful {@code Supplier}s
*
* @author Laurence Gonsalves
* @author Harry Heymann
*/
public final class Suppliers {
private Suppliers() {}
/**
* Returns a new {@code Supplier} which is the composition of the {@code
* Function function} and the {@code Supplier first}. In other words, this
* new {@code Supplier}'s value will be computed by retrieving the value from
* {@code first}, and then applying {@code function} to that value. Note that
* the resulting {@code Supplier} will not force {@code first} (or
* invoke {@code function}) until it is, itself, forced.
*/
public static Supplier compose(final Function function,
final Supplier extends K> first) {
return new SupplierComposition(function, first);
}
private static class SupplierComposition
implements SerializableSupplier {
private final Function function;
private final Supplier extends K> first;
public SupplierComposition(Function function,
Supplier extends K> first) {
this.function = function;
this.first = first;
}
public V get() {
return function.apply(first.get());
}
private static final long serialVersionUID = 239402700273621706L;
}
/**
* Returns a {@code Supplier} which delegates to the given {@code Supplier}
* on the first call to get(), records the value returned, and returns this
* recorded value on all subsequent calls to get(). See:
* memoization
*
* The returned {@code Supplier} will throw {@code CyclicDependencyException}
* if the call to get() tries to get its own value.
*
* The returned supplier is not MT-safe.
*/
public static Supplier memoize(final Supplier delegate) {
return new MemoizingSupplier(delegate);
}
private static class MemoizingSupplier
implements SerializableSupplier {
private final Supplier delegate;
private MemoizationState state = MemoizationState.NOT_YET;
private T value;
public MemoizingSupplier(Supplier delegate) {
this.delegate = delegate;
}
public T get() {
switch (state) {
case NOT_YET:
state = MemoizationState.COMPUTING;
try {
value = delegate.get();
} finally {
state = MemoizationState.NOT_YET;
}
state = MemoizationState.DONE;
break;
case COMPUTING:
throw new CyclicDependencyException();
}
return value;
}
private static final long serialVersionUID = 1138306392412025275L;
}
private enum MemoizationState { NOT_YET, COMPUTING, DONE }
/**
* Exception thrown when a memoizing {@code Supplier} tries to get its
* own value.
*/
public static class CyclicDependencyException extends RuntimeException {
private static final long serialVersionUID = -1;
public CyclicDependencyException() {
super("Cycle detected when forcing a memoizing supplier.");
}
}
/**
* Returns a {@code Supplier} that always supplies {@code instance}.
*/
public static Supplier ofInstance(@Nullable final T instance) {
return new SupplierOfInstance(instance);
}
private static class SupplierOfInstance
implements SerializableSupplier {
private final T instance;
public SupplierOfInstance(T instance) {
this.instance = instance;
}
public T get() {
return instance;
}
private static final long serialVersionUID = 1052627637788228454L;
}
private interface SerializableSupplier
extends Supplier, Serializable {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy