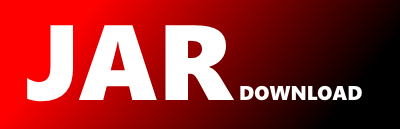
com.google.code.proto.model.ExampleMessageProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpb-stream-reader-writer Show documentation
Show all versions of gpb-stream-reader-writer Show documentation
A package to read and write Google Protocol Buffer messages to and from Input and Output Streams
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: examplemessage.proto
package com.google.code.proto.model;
public final class ExampleMessageProto {
private ExampleMessageProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface ExampleMsgOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
boolean hasName();
String getName();
// required string value = 2;
boolean hasValue();
String getValue();
// required int32 code = 3;
boolean hasCode();
int getCode();
}
public static final class ExampleMsg extends
com.google.protobuf.GeneratedMessage
implements ExampleMsgOrBuilder {
// Use ExampleMsg.newBuilder() to construct.
private ExampleMsg(Builder builder) {
super(builder);
}
private ExampleMsg(boolean noInit) {}
private static final ExampleMsg defaultInstance;
public static ExampleMsg getDefaultInstance() {
return defaultInstance;
}
public ExampleMsg getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMsg_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMsg_fieldAccessorTable;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private Object name_;
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getName() {
Object ref = name_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
name_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getNameBytes() {
Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private Object value_;
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getValue() {
Object ref = value_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
value_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getValueBytes() {
Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 code = 3;
public static final int CODE_FIELD_NUMBER = 3;
private int code_;
public boolean hasCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getCode() {
return code_;
}
private void initFields() {
name_ = "";
value_ = "";
code_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCode()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, code_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, code_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
@java.lang.Override
protected Object writeReplace() throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMsg parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.code.proto.model.ExampleMessageProto.ExampleMsg prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMsg_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMsg_fieldAccessorTable;
}
// Construct using com.google.code.proto.model.ExampleMessageProto.ExampleMsg.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
code_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.code.proto.model.ExampleMessageProto.ExampleMsg.getDescriptor();
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg getDefaultInstanceForType() {
return com.google.code.proto.model.ExampleMessageProto.ExampleMsg.getDefaultInstance();
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg build() {
com.google.code.proto.model.ExampleMessageProto.ExampleMsg result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.google.code.proto.model.ExampleMessageProto.ExampleMsg buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.google.code.proto.model.ExampleMessageProto.ExampleMsg result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg buildPartial() {
com.google.code.proto.model.ExampleMessageProto.ExampleMsg result = new com.google.code.proto.model.ExampleMessageProto.ExampleMsg(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.code_ = code_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.code.proto.model.ExampleMessageProto.ExampleMsg) {
return mergeFrom((com.google.code.proto.model.ExampleMessageProto.ExampleMsg)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.code.proto.model.ExampleMessageProto.ExampleMsg other) {
if (other == com.google.code.proto.model.ExampleMessageProto.ExampleMsg.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasCode()) {
setCode(other.getCode());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
if (!hasCode()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
code_ = input.readInt32();
break;
}
}
}
}
private int bitField0_;
// required string name = 1;
private Object name_ = "";
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getName() {
Object ref = name_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
name_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setName(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
void setName(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
}
// required string value = 2;
private Object value_ = "";
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getValue() {
Object ref = value_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
value_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setValue(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
void setValue(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
}
// required int32 code = 3;
private int code_ ;
public boolean hasCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getCode() {
return code_;
}
public Builder setCode(int value) {
bitField0_ |= 0x00000004;
code_ = value;
onChanged();
return this;
}
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000004);
code_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:com.google.code.proto.model.ExampleMsg)
}
static {
defaultInstance = new ExampleMsg(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.google.code.proto.model.ExampleMsg)
}
public interface ExampleMessagesOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .com.google.code.proto.model.ExampleMsg msg = 1;
java.util.List
getMsgList();
com.google.code.proto.model.ExampleMessageProto.ExampleMsg getMsg(int index);
int getMsgCount();
java.util.List extends com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder>
getMsgOrBuilderList();
com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder getMsgOrBuilder(
int index);
}
public static final class ExampleMessages extends
com.google.protobuf.GeneratedMessage
implements ExampleMessagesOrBuilder {
// Use ExampleMessages.newBuilder() to construct.
private ExampleMessages(Builder builder) {
super(builder);
}
private ExampleMessages(boolean noInit) {}
private static final ExampleMessages defaultInstance;
public static ExampleMessages getDefaultInstance() {
return defaultInstance;
}
public ExampleMessages getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMessages_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMessages_fieldAccessorTable;
}
// repeated .com.google.code.proto.model.ExampleMsg msg = 1;
public static final int MSG_FIELD_NUMBER = 1;
private java.util.List msg_;
public java.util.List getMsgList() {
return msg_;
}
public java.util.List extends com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder>
getMsgOrBuilderList() {
return msg_;
}
public int getMsgCount() {
return msg_.size();
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg getMsg(int index) {
return msg_.get(index);
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder getMsgOrBuilder(
int index) {
return msg_.get(index);
}
private void initFields() {
msg_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getMsgCount(); i++) {
if (!getMsg(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < msg_.size(); i++) {
output.writeMessage(1, msg_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < msg_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, msg_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
@java.lang.Override
protected Object writeReplace() throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.google.code.proto.model.ExampleMessageProto.ExampleMessages parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.google.code.proto.model.ExampleMessageProto.ExampleMessages prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.google.code.proto.model.ExampleMessageProto.ExampleMessagesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMessages_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.code.proto.model.ExampleMessageProto.internal_static_com_google_code_proto_model_ExampleMessages_fieldAccessorTable;
}
// Construct using com.google.code.proto.model.ExampleMessageProto.ExampleMessages.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getMsgFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (msgBuilder_ == null) {
msg_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
msgBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.code.proto.model.ExampleMessageProto.ExampleMessages.getDescriptor();
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMessages getDefaultInstanceForType() {
return com.google.code.proto.model.ExampleMessageProto.ExampleMessages.getDefaultInstance();
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMessages build() {
com.google.code.proto.model.ExampleMessageProto.ExampleMessages result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.google.code.proto.model.ExampleMessageProto.ExampleMessages buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.google.code.proto.model.ExampleMessageProto.ExampleMessages result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMessages buildPartial() {
com.google.code.proto.model.ExampleMessageProto.ExampleMessages result = new com.google.code.proto.model.ExampleMessageProto.ExampleMessages(this);
int from_bitField0_ = bitField0_;
if (msgBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
msg_ = java.util.Collections.unmodifiableList(msg_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.msg_ = msg_;
} else {
result.msg_ = msgBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.code.proto.model.ExampleMessageProto.ExampleMessages) {
return mergeFrom((com.google.code.proto.model.ExampleMessageProto.ExampleMessages)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.code.proto.model.ExampleMessageProto.ExampleMessages other) {
if (other == com.google.code.proto.model.ExampleMessageProto.ExampleMessages.getDefaultInstance()) return this;
if (msgBuilder_ == null) {
if (!other.msg_.isEmpty()) {
if (msg_.isEmpty()) {
msg_ = other.msg_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMsgIsMutable();
msg_.addAll(other.msg_);
}
onChanged();
}
} else {
if (!other.msg_.isEmpty()) {
if (msgBuilder_.isEmpty()) {
msgBuilder_.dispose();
msgBuilder_ = null;
msg_ = other.msg_;
bitField0_ = (bitField0_ & ~0x00000001);
msgBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getMsgFieldBuilder() : null;
} else {
msgBuilder_.addAllMessages(other.msg_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getMsgCount(); i++) {
if (!getMsg(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder subBuilder = com.google.code.proto.model.ExampleMessageProto.ExampleMsg.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addMsg(subBuilder.buildPartial());
break;
}
}
}
}
private int bitField0_;
// repeated .com.google.code.proto.model.ExampleMsg msg = 1;
private java.util.List msg_ =
java.util.Collections.emptyList();
private void ensureMsgIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
msg_ = new java.util.ArrayList(msg_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.code.proto.model.ExampleMessageProto.ExampleMsg, com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder, com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder> msgBuilder_;
public java.util.List getMsgList() {
if (msgBuilder_ == null) {
return java.util.Collections.unmodifiableList(msg_);
} else {
return msgBuilder_.getMessageList();
}
}
public int getMsgCount() {
if (msgBuilder_ == null) {
return msg_.size();
} else {
return msgBuilder_.getCount();
}
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg getMsg(int index) {
if (msgBuilder_ == null) {
return msg_.get(index);
} else {
return msgBuilder_.getMessage(index);
}
}
public Builder setMsg(
int index, com.google.code.proto.model.ExampleMessageProto.ExampleMsg value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMsgIsMutable();
msg_.set(index, value);
onChanged();
} else {
msgBuilder_.setMessage(index, value);
}
return this;
}
public Builder setMsg(
int index, com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder builderForValue) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.set(index, builderForValue.build());
onChanged();
} else {
msgBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
public Builder addMsg(com.google.code.proto.model.ExampleMessageProto.ExampleMsg value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMsgIsMutable();
msg_.add(value);
onChanged();
} else {
msgBuilder_.addMessage(value);
}
return this;
}
public Builder addMsg(
int index, com.google.code.proto.model.ExampleMessageProto.ExampleMsg value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMsgIsMutable();
msg_.add(index, value);
onChanged();
} else {
msgBuilder_.addMessage(index, value);
}
return this;
}
public Builder addMsg(
com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder builderForValue) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.add(builderForValue.build());
onChanged();
} else {
msgBuilder_.addMessage(builderForValue.build());
}
return this;
}
public Builder addMsg(
int index, com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder builderForValue) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.add(index, builderForValue.build());
onChanged();
} else {
msgBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
public Builder addAllMsg(
java.lang.Iterable extends com.google.code.proto.model.ExampleMessageProto.ExampleMsg> values) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
super.addAll(values, msg_);
onChanged();
} else {
msgBuilder_.addAllMessages(values);
}
return this;
}
public Builder clearMsg() {
if (msgBuilder_ == null) {
msg_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
msgBuilder_.clear();
}
return this;
}
public Builder removeMsg(int index) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.remove(index);
onChanged();
} else {
msgBuilder_.remove(index);
}
return this;
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder getMsgBuilder(
int index) {
return getMsgFieldBuilder().getBuilder(index);
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder getMsgOrBuilder(
int index) {
if (msgBuilder_ == null) {
return msg_.get(index); } else {
return msgBuilder_.getMessageOrBuilder(index);
}
}
public java.util.List extends com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder>
getMsgOrBuilderList() {
if (msgBuilder_ != null) {
return msgBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(msg_);
}
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder addMsgBuilder() {
return getMsgFieldBuilder().addBuilder(
com.google.code.proto.model.ExampleMessageProto.ExampleMsg.getDefaultInstance());
}
public com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder addMsgBuilder(
int index) {
return getMsgFieldBuilder().addBuilder(
index, com.google.code.proto.model.ExampleMessageProto.ExampleMsg.getDefaultInstance());
}
public java.util.List
getMsgBuilderList() {
return getMsgFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.code.proto.model.ExampleMessageProto.ExampleMsg, com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder, com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder>
getMsgFieldBuilder() {
if (msgBuilder_ == null) {
msgBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.code.proto.model.ExampleMessageProto.ExampleMsg, com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder, com.google.code.proto.model.ExampleMessageProto.ExampleMsgOrBuilder>(
msg_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
msg_ = null;
}
return msgBuilder_;
}
// @@protoc_insertion_point(builder_scope:com.google.code.proto.model.ExampleMessages)
}
static {
defaultInstance = new ExampleMessages(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:com.google.code.proto.model.ExampleMessages)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_google_code_proto_model_ExampleMsg_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_google_code_proto_model_ExampleMsg_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_com_google_code_proto_model_ExampleMessages_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_com_google_code_proto_model_ExampleMessages_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024examplemessage.proto\022\033com.google.code." +
"proto.model\"7\n\nExampleMsg\022\014\n\004name\030\001 \002(\t\022" +
"\r\n\005value\030\002 \002(\t\022\014\n\004code\030\003 \002(\005\"G\n\017ExampleM" +
"essages\0224\n\003msg\030\001 \003(\0132\'.com.google.code.p" +
"roto.model.ExampleMsgB2\n\033com.google.code" +
".proto.modelB\023ExampleMessageProto"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_com_google_code_proto_model_ExampleMsg_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_google_code_proto_model_ExampleMsg_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_google_code_proto_model_ExampleMsg_descriptor,
new java.lang.String[] { "Name", "Value", "Code", },
com.google.code.proto.model.ExampleMessageProto.ExampleMsg.class,
com.google.code.proto.model.ExampleMessageProto.ExampleMsg.Builder.class);
internal_static_com_google_code_proto_model_ExampleMessages_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_com_google_code_proto_model_ExampleMessages_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_com_google_code_proto_model_ExampleMessages_descriptor,
new java.lang.String[] { "Msg", },
com.google.code.proto.model.ExampleMessageProto.ExampleMessages.class,
com.google.code.proto.model.ExampleMessageProto.ExampleMessages.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy