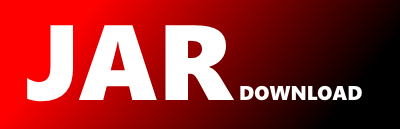
org.jscep.client.verification.CachingCertificateVerifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jscep Show documentation
Show all versions of jscep Show documentation
Java implementation of the Simple Certificate Enrollment Protocol
package org.jscep.client.verification;
import java.security.cert.Certificate;
import java.security.cert.X509Certificate;
import java.util.HashMap;
import java.util.Map;
/**
* This CertificateVerifier delegates verification to the provided
* CertificateVerifier and caches the answer.
*/
public final class CachingCertificateVerifier implements CertificateVerifier {
/**
* Previously received verification answers.
*/
private final Map verificationAnswers;
/**
* The verifier to delegate to.
*/
private final CertificateVerifier delegate;
/**
* Constructs a CachingCertificateVerifier which delegates to the
* specified CertificateVerifier.
*
* @param delegate
* the CertificateVerifier to delegate to.
*/
public CachingCertificateVerifier(final CertificateVerifier delegate) {
this.delegate = delegate;
this.verificationAnswers = new HashMap();
}
/**
* This implementation will forward the given certificate to the delegate
* provided in the constructor, and cache the delegate's response.
*
* On every subsequent invocation with the same certificate, the initial
* response from the delegate will be returned.
*
* @param cert
* the certificate to verify.
* @return the result of calling verify on the delegate
* CertificateVerifier.
*/
@Override
public synchronized boolean verify(final X509Certificate cert) {
if (verificationAnswers.containsKey(cert)) {
return verificationAnswers.get(cert);
} else {
boolean answer = delegate.verify(cert);
verificationAnswers.put(cert, answer);
return answer;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy