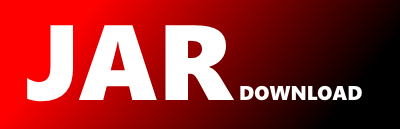
com.google.code.liquidform.HavingClause Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of liquidform.jpa Show documentation
Show all versions of liquidform.jpa Show documentation
Provides a Java Domain Specific Language for building type-safe and refactoring proof JPA queries.
The newest version!
/*
* Copyright (C) 2008-2009 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.code.liquidform;
import java.util.Date;
import com.google.code.liquidform.conditions.ConditionalExpression;
/**
* Represents the optional HAVING part of the query. An instance of
* this may be added to the query using the
* {@link GroupByClause#having(ConditionalExpression)} syntax, or one of its
* shortcut methods.
*
* @param
* the type that was selected by the {@link SelectClause SELECT
* construct}
*/
public class HavingClause extends AbstractClause implements SubQuery {
private final ConditionalExpression conditionalExpression;
/* default */HavingClause(GroupByClause previousClause,
ConditionalExpression conditionalExpression) {
super(previousClause);
this.conditionalExpression = conditionalExpression;
}
@Override
StringBuilder addTo(StringBuilder buffer) {
buffer.append(" having ").append(
conditionalExpression.getPreferredStringRepresentation());
return buffer;
}
// ============================ O R D E R B Y ==========================
public OrderByClause orderBy(Number o) {
return this.orderBy(o, SortDirection.ASC);
}
public OrderByClause orderBy(Number o, SortDirection dir) {
return new OrderByClause(this, o, dir);
}
public OrderByClause orderBy(Date o) {
return this.orderBy(o, SortDirection.ASC);
}
public OrderByClause orderBy(Date o, SortDirection dir) {
return new OrderByClause(this, o, dir);
}
public OrderByClause orderBy(String o) {
return this.orderBy(o, SortDirection.ASC);
}
public OrderByClause orderBy(String o, SortDirection dir) {
return new OrderByClause(this, o, dir);
}
public OrderByClause orderBy(Character o) {
return this.orderBy(o, SortDirection.ASC);
}
public OrderByClause orderBy(Character o, SortDirection dir) {
return new OrderByClause(this, o, dir);
}
// TODO : legal or not?
// public OrderByClause orderBy(Enum o) {
// return this.orderBy(o, SortDirection.ASC);
// }
//
// public OrderByClause orderBy(Enum o, SortDirection dir) {
// return new OrderByClause(this, o, dir);
// }
}