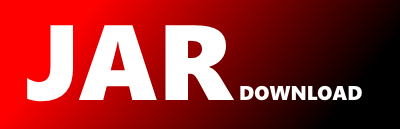
com.google.code.liquidform.internal.WeakIdentityHashMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of liquidform.jpa Show documentation
Show all versions of liquidform.jpa Show documentation
Provides a Java Domain Specific Language for building type-safe and refactoring proof JPA queries.
The newest version!
/*
* Copyright (C) 2008-2009 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.code.liquidform.internal;
import java.lang.ref.Reference;
import java.lang.ref.ReferenceQueue;
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.Map;
import java.util.WeakHashMap;
/**
* Quick and dirty mix of {@link WeakHashMap} and {@link IdentityHashMap}. This
* class does not implement {@link Map} per se but provides the methods
* we need.
*/
/* default */class WeakIdentityHashMap {
/**
* Considers that two objects are equal when they both reference the same
* (with == semantics) referent.
*/
private static class IdentityWeakReference extends WeakReference {
private final int hashCode;
IdentityWeakReference(T o) {
this(o, null);
}
IdentityWeakReference(T o, ReferenceQueue q) {
super(o, q);
this.hashCode = (o == null) ? 0 : System.identityHashCode(o);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof IdentityWeakReference)) {
return false;
}
IdentityWeakReference wr = (IdentityWeakReference) o;
T got = get();
return (got != null && got == wr.get());
}
@Override
public int hashCode() {
return hashCode;
}
}
private Map, V> map = new HashMap, V>();
private ReferenceQueue referenceQueue = new ReferenceQueue();
private void expunge() {
Reference extends K> ref;
while ((ref = referenceQueue.poll()) != null) {
map.remove(ref);
}
}
public V get(K key) {
expunge();
WeakReference keyref = makeReference(key);
return map.get(keyref);
}
private WeakReference makeReference(K referent) {
return new IdentityWeakReference(referent);
}
private WeakReference makeReference(K referent, ReferenceQueue q) {
return new IdentityWeakReference(referent, q);
}
public V put(K key, V value) {
expunge();
if (key == null) {
throw new NullPointerException("Null key");
}
WeakReference keyref = makeReference(key, referenceQueue);
return map.put(keyref, value);
}
public V remove(K key) {
expunge();
WeakReference keyref = makeReference(key);
return map.remove(keyref);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy