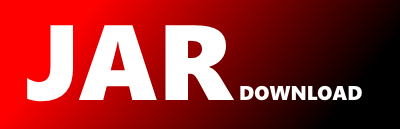
net.sourceforge.cobertura.javancss.Util Maven / Gradle / Ivy
Show all versions of cobertura Show documentation
/* ***** BEGIN LICENSE BLOCK *****
* Version: MPL 1.1/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is the reusable ccl java library
* (http://www.kclee.com/clemens/java/ccl/).
*
* The Initial Developer of the Original Code is
* Chr. Clemens Lee.
* Portions created by Chr. Clemens Lee are Copyright (C) 2002
* Chr. Clemens Lee. All Rights Reserved.
*
* Contributor(s): Chr. Clemens Lee
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or
* the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the MPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the MPL, the GPL or the LGPL.
*
* ***** END LICENSE BLOCK ***** */
package net.sourceforge.cobertura.javancss;
import java.util.Vector;
/**
* A general purpose class with a variety of support and convenience methods.
*
* There are different groups of methods in this class:
*
*
print methods - convenience methods for System.out.print etc. that additionally make sure output is gets flushed immediately.
*
string methods
*
string/vector converter methods
*
miscellaneous methods
*
*
* Some basic but none the less the most used methods by myself are:
* - {@link #isEmpty(java.lang.String) isEmpty}
* - {@link #stringToLines(java.lang.String) stringToLines}
* - {@link #sleep(int) sleep}
*
*
* @author
* Chr. Clemens Lee
* <
* [email protected]
* >
*/
public class Util
{
public static final Object CONSTANT_OBJECT = new Object();
/**
* This is an utility class, there is (should be) no need
* for an instance of this class.
*/
private Util()
{
super();
}
// -----------------------------------------------------
// debug methods and assertion stuff
// -----------------------------------------------------
/**
* panicIf <=> not assert. Throws ApplicationException if true.
* It's not necessary to catch this exception.
*/
static void panicIf(boolean bPanic_)
{
if (bPanic_)
{
throw (new RuntimeException());
}
}
/**
* panicIf <=> not assert. Throws ApplicationException if true.
* It's not necessary to catch this exception.
*
* @param sMessage_ The error message for the Exception.
*/
static void panicIf(boolean bPanic_, String sMessage_)
{
if (bPanic_)
{
throw (new RuntimeException(sMessage_));
}
}
/**
* Tests, if a given String equals null or "".
*/
public static boolean isEmpty(String sTest_)
{
if (sTest_ == null || sTest_.equals(""))
{
return true;
}
return false;
}
/**
* This function takes a String and separates it into different
* lines. The last line does not need to have a separator character.
*
* @param lines_ The number of lines that should be extracted.
* Zero if maximum number of lines is requested.
* @param cCutter_ The character that separates pString_ into
* different lines
*
* @return The single lines do not contain the cCutter_
* character at the end.
*/
private static Vector stringToLines(int lines_, String pString_, char cCutter_)
{
int maxLines = Integer.MAX_VALUE;
if (lines_ > 0)
{
maxLines = lines_;
}
Vector vRetVal = new Vector();
if (pString_ == null)
{
return vRetVal;
}
int startIndex = 0;
for (; maxLines > 0; maxLines--)
{
int endIndex = pString_.indexOf(cCutter_, startIndex);
if (endIndex == -1)
{
if (startIndex < pString_.length())
{
endIndex = pString_.length();
}
else
{
break;
}
}
String sLine = pString_.substring(startIndex, endIndex);
vRetVal.addElement(sLine);
startIndex = endIndex + 1;
}
return vRetVal;
}
/**
* This function takes a String and separates it into different
* lines. The last line does not need to have a separator character.
*
* @param cCutter_ The character that separates pString_ into
* different lines
*
* @return The single lines do not contain the cCutter_ character
* at the end.
*/
private static Vector stringToLines(String pString_, char cCutter_)
{
return stringToLines(0, pString_, cCutter_);
}
/**
* This function takes a String and separates it into different
* lines. The last line does not need to have a '\n'. The function
* can't handle dos carriage returns.
*
* @return The single lines do not contain the '\n' character
* at the end.
*/
public static Vector stringToLines(String pString_)
{
return stringToLines(pString_, '\n');
}
/**
* Current thread sleeps in seconds.
*/
private static void sleep(int seconds_)
{
try
{
Thread.sleep(seconds_ * 1000);
}
catch (Exception pException)
{
}
}
}