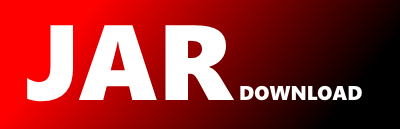
net.spy.memcached.internal.BulkGetFuture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spymemcached Show documentation
Show all versions of spymemcached Show documentation
A simple, asynchronous, single-threaded Memcached client written in java.
The newest version!
package net.spy.memcached.internal;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import net.spy.memcached.MemcachedConnection;
import net.spy.memcached.compat.log.LoggerFactory;
import net.spy.memcached.ops.Operation;
import net.spy.memcached.ops.OperationState;
/**
* Future for handling results from bulk gets.
*
* Not intended for general use.
*
* @param types of objects returned from the GET
*/
public class BulkGetFuture implements BulkFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy