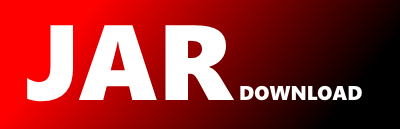
com.google.code.play.AbstractPlayDistMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of play-maven-plugin Show documentation
Show all versions of play-maven-plugin Show documentation
Maven Plugin for Play! Framework for integration of these two very different worlds
/*
* Copyright 2010-2014 Grzegorz Slowikowski
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.code.play;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.resolver.filter.AndArtifactFilter;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.shared.artifact.filter.PatternExcludesArtifactFilter;
import org.apache.maven.shared.artifact.filter.PatternIncludesArtifactFilter;
import org.apache.maven.shared.dependency.tree.DependencyTreeBuilderException;
import org.codehaus.plexus.archiver.manager.NoSuchArchiverException;
import org.codehaus.plexus.archiver.zip.ZipArchiver;
/**
* Base class for Play! distribution packaging mojos.
*
* @author Grzegorz Slowikowski
*/
public abstract class AbstractPlayDistMojo
extends AbstractArchivingMojo
{
/**
* Default Play! id (profile).
*
* @since 1.0.0
*/
@Parameter( property = "play.id", defaultValue = "" )
private String playId;
/**
* Distribution application resources include filter
*
* @since 1.0.0
*/
@Parameter( property = "play.distApplicationIncludes", defaultValue = "app/**,conf/**,public/**,tags/**,test/**" )
private String distApplicationIncludes;
/**
* Distribution application resources exclude filter.
*
* @since 1.0.0
*/
@Parameter( property = "play.distApplicationExcludes", defaultValue = "" )
private String distApplicationExcludes;
/**
* Distribution dependency include filter.
*
* @since 1.0.0
*/
@Parameter( property = "play.distDependencyIncludes", defaultValue = "" )
private String distDependencyIncludes;
/**
* Distribution dependency exclude filter.
*
* @since 1.0.0
*/
@Parameter( property = "play.distDependencyExcludes", defaultValue = "" )
private String distDependencyExcludes;
protected ZipArchiver prepareArchiver( ConfigurationParser configParser )
throws DependencyTreeBuilderException, IOException, MojoExecutionException, NoSuchArchiverException
{
ZipArchiver zipArchiver = getZipArchiver();
File baseDir = project.getBasedir();
Set providedModuleNames = getProvidedModuleNames( configParser, playId, false );
// APPLICATION
getLog().debug( "Dist includes: " + distApplicationIncludes );
getLog().debug( "Dist excludes: " + distApplicationExcludes );
String[] applicationIncludes = null;
if ( distApplicationIncludes != null )
{
applicationIncludes = distApplicationIncludes.split( "," );
}
// TODO-don't add "test/**" if profile is not test profile
String[] applicationExcludes = null;
if ( distApplicationExcludes != null )
{
applicationExcludes = distApplicationExcludes.split( "," );
}
zipArchiver.addDirectory( baseDir, "application/", applicationIncludes, applicationExcludes );
// preparation
Set> projectArtifacts = project.getArtifacts();
Set excludedArtifacts = new HashSet();
Artifact playSeleniumJunit4Artifact =
getDependencyArtifact( projectArtifacts, "com.google.code.maven-play-plugin", "play-selenium-junit4", "jar" );
if ( playSeleniumJunit4Artifact != null )
{
excludedArtifacts.addAll( getDependencyArtifacts( projectArtifacts, playSeleniumJunit4Artifact ) );
}
AndArtifactFilter dependencyFilter = new AndArtifactFilter();
if ( distDependencyIncludes != null && distDependencyIncludes.length() > 0 )
{
List incl = Arrays.asList( distDependencyIncludes.split( "," ) );
PatternIncludesArtifactFilter includeFilter =
new PatternIncludesArtifactFilter( incl, true/* actTransitively */ );
dependencyFilter.add( includeFilter );
}
if ( distDependencyExcludes != null && distDependencyExcludes.length() > 0 )
{
List excl = Arrays.asList( distDependencyExcludes.split( "," ) );
PatternExcludesArtifactFilter excludeFilter =
new PatternExcludesArtifactFilter( excl, true/* actTransitively */ );
dependencyFilter.add( excludeFilter );
}
Set filteredArtifacts = new HashSet(); // TODO-rename to filteredClassPathArtifacts
for ( Iterator> iter = projectArtifacts.iterator(); iter.hasNext(); )
{
Artifact artifact = (Artifact) iter.next();
if ( artifact.getArtifactHandler().isAddedToClasspath() && !excludedArtifacts.contains( artifact ) )
{
// TODO-add checkPotentialReactorProblem( artifact );
if ( dependencyFilter.include( artifact ) )
{
filteredArtifacts.add( artifact );
}
else
{
getLog().debug( artifact.toString() + " excluded" );
}
}
}
// framework
Artifact frameworkZipArtifact = findFrameworkArtifact( false );
// TODO-validate not null
File frameworkZipFile = frameworkZipArtifact.getFile();
zipArchiver.addArchivedFileSet( frameworkZipFile );
Artifact frameworkJarArtifact =
getDependencyArtifact( filteredArtifacts/* ?? */, frameworkZipArtifact.getGroupId(),
frameworkZipArtifact.getArtifactId(), "jar" );
// TODO-validate not null
File frameworkJarFile = frameworkJarArtifact.getFile();
String frameworkDestinationFileName = "framework/" + frameworkJarFile.getName();
String playVersion = frameworkJarArtifact.getBaseVersion();
if ( "1.2".compareTo( playVersion ) > 0 )
{
// Play 1.1.x
frameworkDestinationFileName = "framework/play.jar";
}
zipArchiver.addFile( frameworkJarFile, frameworkDestinationFileName );
filteredArtifacts.remove( frameworkJarArtifact );
Set dependencySubtree = getFrameworkDependencyArtifacts( filteredArtifacts, frameworkJarArtifact );
for ( Artifact classPathArtifact : dependencySubtree )
{
File jarFile = classPathArtifact.getFile();
String destinationFileName = "framework/lib/" + jarFile.getName();
zipArchiver.addFile( jarFile, destinationFileName );
filteredArtifacts.remove( classPathArtifact );
}
// modules/*/lib and application/modules/*/lib
Set notActiveProvidedModules = new HashSet();
Map moduleArtifacts = findAllModuleArtifacts( false );
for ( Map.Entry moduleArtifactEntry : moduleArtifacts.entrySet() )
{
String moduleName = moduleArtifactEntry.getKey();
Artifact moduleZipArtifact = moduleArtifactEntry.getValue();
File moduleZipFile = moduleZipArtifact.getFile();
if ( Artifact.SCOPE_PROVIDED.equals( moduleZipArtifact.getScope() ) )
{
if ( providedModuleNames.contains( moduleName ) )
{
String moduleSubDir =
String.format( "modules/%s-%s/", moduleName, moduleZipArtifact.getBaseVersion() );
if ( isFrameworkEmbeddedModule( moduleName ) )
{
moduleSubDir = String.format( "modules/%s/", moduleName );
}
zipArchiver.addArchivedFileSet( moduleZipFile, moduleSubDir );
dependencySubtree = getModuleDependencyArtifacts( filteredArtifacts, moduleZipArtifact );
for ( Artifact classPathArtifact : dependencySubtree )
{
File jarFile = classPathArtifact.getFile();
String destinationFileName = jarFile.getName();
// Scala module hack
if ( "scala".equals( moduleName ) )
{
destinationFileName = scalaHack( classPathArtifact );
}
String destinationPath = String.format( "%slib/%s", moduleSubDir, destinationFileName );
zipArchiver.addFile( jarFile, destinationPath );
filteredArtifacts.remove( classPathArtifact );
}
}
else
{
notActiveProvidedModules.add( moduleZipArtifact );
}
}
else
{
String moduleSubDir =
String.format( "application/modules/%s-%s/", moduleName, moduleZipArtifact.getBaseVersion() );
zipArchiver.addArchivedFileSet( moduleZipFile, moduleSubDir );
dependencySubtree = getModuleDependencyArtifacts( filteredArtifacts, moduleZipArtifact );
for ( Artifact classPathArtifact : dependencySubtree )
{
File jarFile = classPathArtifact.getFile();
String destinationFileName = jarFile.getName();
// Scala module hack
if ( "scala".equals( moduleName ) )
{
destinationFileName = scalaHack( classPathArtifact );
}
String destinationPath =
String.format( "application/modules/%s-%s/lib/%s", moduleName, moduleZipArtifact.getBaseVersion(),
destinationFileName );
zipArchiver.addFile( jarFile, destinationPath );
filteredArtifacts.remove( classPathArtifact );
}
}
}
for ( Artifact moduleZipArtifact : notActiveProvidedModules )
{
dependencySubtree = getModuleDependencyArtifacts( filteredArtifacts, moduleZipArtifact );
filteredArtifacts.removeAll( dependencySubtree );
}
// application/lib
for ( Iterator> iter = filteredArtifacts.iterator(); iter.hasNext(); )
{
Artifact artifact = (Artifact) iter.next();
File jarFile = artifact.getFile();
String destinationFileName = "application/lib/" + jarFile.getName();
zipArchiver.addFile( jarFile, destinationFileName );
}
checkArchiverForProblems( zipArchiver );
return zipArchiver;
}
private String scalaHack( Artifact dependencyArtifact ) throws IOException
{
String destinationFileName = dependencyArtifact.getFile().getName();
if ( "org.scala-lang".equals( dependencyArtifact.getGroupId() )
&& ( "scala-compiler".equals( dependencyArtifact.getArtifactId() ) || "scala-library".equals( dependencyArtifact.getArtifactId() ) )
&& "jar".equals( dependencyArtifact.getType() ) )
{
destinationFileName = dependencyArtifact.getArtifactId() + ".jar";
}
return destinationFileName;
}
protected ConfigurationParser getConfiguration() throws IOException
{
return getConfiguration( playId );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy