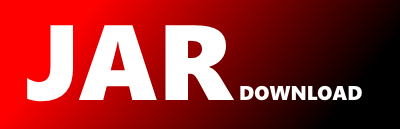
com.google.code.plsqlmaven.xdb.XdbMojo.groovy Maven / Gradle / Ivy
The newest version!
package com.google.code.plsqlmaven.xdb;
/*
* Copyright 2001-2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.codehaus.groovy.maven.mojo.GroovyMojo
import groovy.sql.Sql
import com.google.code.plsqlmaven.shared.XdbUtils
/**
* Abstract class for XDB related Mojos
*
*/
public abstract class XdbMojo
extends GroovyMojo
{
/**
* Database username.
* @since 1.0
* @parameter expression="${username}"
*/
protected String username;
/**
* Database password.
* @since 1.0
* @parameter expression="${password}"
*/
protected String password;
/**
* Database URL.
* @parameter expression="${url}"
* @since 1.0
*/
protected String url;
/**
* @parameter expression="${project}"
* @required
* @readonly
*/
protected org.apache.maven.project.MavenProject project
/**
* The base path from witch the export should start
* @since 1.0
* @parameter expression="${basePath}"
* @required
*/
protected String basePath;
/**
* A list of comma separated file extensions to
* enable html entity translation
* @since 1.0
* @parameter expression="${translateEntities}"
*/
protected String translateEntities;
/**
* Database connection helper
*/
protected Sql sql
protected XdbUtils xdbUtils;
public void disconnectFromDatabase()
{
if (sql)
sql.close();
}
public boolean connectToDatabase()
{
if (url)
{
log.debug( "connecting to " + url )
sql = Sql.newInstance(url, username, password, "oracle.jdbc.driver.OracleDriver")
xdbUtils= new XdbUtils(ant,log,sql)
}
else
sql= null;
return (sql!=null);
}
/**
* Source directory for XDB files src/main/xdb
*/
public String getXdbSourceDirectory()
{
return project.basedir.absolutePath+File.separator+"src"+File.separator+"main"+File.separator+"xdb"+File.separator;
}
public String[] getTranslateEntitiesExtensions()
{
if (translateEntities)
return translateEntities.split(',')
else
return []
}
protected boolean translateEntitiesEnabled(path)
{
for (ext in translateEntitiesExtensions)
if (path.endsWith(ext))
return true;
return false;
}
protected removeHtmlEntities(text)
{
def retval= text;
entities.each
{ retval= retval.replaceAll(it.key,it.value); }
return retval;
}
protected recreateHtmlEntities(text)
{
def retval= text;
entities.each
{ retval= retval.replaceAll(it.value,it.key); }
return retval;
}
protected entities= [
' ': ' ',
'¡': '¡',
'¢': '¢',
'£': '£',
'¤': '¤',
'¥': '¥',
'¦': '¦',
'§': '§',
'¨': '¨',
'©': '©',
'ª': 'ª',
'«': '«',
'¬': '¬',
'': '',
'®': '®',
'¯': '¯',
'°': '°',
'±': '±',
'²': '²',
'³': '³',
'´': '´',
'µ': 'µ',
'¶': '¶',
'·': '·',
'¸': '¸',
'¹': '¹',
'º': 'º',
'»': '»',
'¼': '¼',
'½': '½',
'¾': '¾',
'¿': '¿',
'À': 'À',
'Á': 'Á',
'Â': 'Â',
'Ã': 'Ã',
'Ä': 'Ä',
'Å': 'Å',
'Æ': 'Æ',
'Ç': 'Ç',
'È': 'È',
'É': 'É',
'Ê': 'Ê',
'Ë': 'Ë',
'Ì': 'Ì',
'Í': 'Í',
'Î': 'Î',
'Ï': 'Ï',
'Ð': 'Ð',
'Ñ': 'Ñ',
'Ò': 'Ò',
'Ó': 'Ó',
'Ô': 'Ô',
'Õ': 'Õ',
'Ö': 'Ö',
'×': '×',
'Ø': 'Ø',
'Ù': 'Ù',
'Ú': 'Ú',
'Û': 'Û',
'Ü': 'Ü',
'Ý': 'Ý',
'Þ': 'Þ',
'ß': 'ß',
'à': 'à',
'á': 'á',
'â': 'â',
'ã': 'ã',
'ä': 'ä',
'å': 'å',
'æ': 'æ',
'ç': 'ç',
'è': 'è',
'é': 'é',
'ê': 'ê',
'ë': 'ë',
'ì': 'ì',
'í': 'í',
'î': 'î',
'ï': 'ï',
'ð': 'ð',
'ñ': 'ñ',
'ò': 'ò',
'ó': 'ó',
'ô': 'ô',
'õ': 'õ',
'ö': 'ö',
'÷': '÷',
'ø': 'ø',
'ù': 'ù',
'ú': 'ú',
'û': 'û',
'ü': 'ü',
'ý': 'ý',
'þ': 'þ',
'ÿ': 'ÿ',
'ƒ': 'ƒ',
'Α': 'Α',
'Β': 'Β',
'Γ': 'Γ',
'Δ': 'Δ',
'Ε': 'Ε',
'Ζ': 'Ζ',
'Η': 'Η',
'Θ': 'Θ',
'Ι': 'Ι',
'Κ': 'Κ',
'Λ': 'Λ',
'Μ': 'Μ',
'Ν': 'Ν',
'Ξ': 'Ξ',
'Ο': 'Ο',
'Π': 'Π',
'Ρ': 'Ρ',
'Σ': 'Σ',
'Τ': 'Τ',
'Υ': 'Υ',
'Φ': 'Φ',
'Χ': 'Χ',
'Ψ': 'Ψ',
'Ω': 'Ω',
'α': 'α',
'β': 'β',
'γ': 'γ',
'δ': 'δ',
'ε': 'ε',
'ζ': 'ζ',
'η': 'η',
'θ': 'θ',
'ι': 'ι',
'κ': 'κ',
'λ': 'λ',
'μ': 'μ',
'ν': 'ν',
'ξ': 'ξ',
'ο': 'ο',
'π': 'π',
'ρ': 'ρ',
'ς': 'ς',
'σ': 'σ',
'τ': 'τ',
'υ': 'υ',
'φ': 'φ',
'χ': 'χ',
'ψ': 'ψ',
'ω': 'ω',
'ϑ': 'ϑ',
'ϒ': 'ϒ',
'ϖ': 'ϖ',
'•': '•',
'…': '…',
'′': '′',
'″': '″',
'‾': '‾',
'⁄': '⁄',
'℘': '℘',
'ℑ': 'ℑ',
'ℜ': 'ℜ',
'™': '™',
'ℵ': 'ℵ',
'←': '←',
'↑': '↑',
'→': '→',
'↓': '↓',
'↔': '↔',
'↵': '↵',
'⇐': '⇐',
'⇑': '⇑',
'⇒': '⇒',
'⇓': '⇓',
'⇔': '⇔',
'∀': '∀',
'∂': '∂',
'∃': '∃',
'∅': '∅',
'∇': '∇',
'∈': '∈',
'∉': '∉',
'∋': '∋',
'∏': '∏',
'∑': '∑',
'−': '−',
'∗': '∗',
'√': '√',
'∝': '∝',
'∞': '∞',
'∠': '∠',
'∧': '∧',
'∨': '∨',
'∩': '∩',
'∪': '∪',
'∫': '∫',
'∴': '∴',
'∼': '∼',
'≅': '≅',
'≈': '≈',
'≠': '≠',
'≡': '≡',
'≤': '≤',
'≥': '≥',
'⊂': '⊂',
'⊃': '⊃',
'⊄': '⊄',
'⊆': '⊆',
'⊇': '⊇',
'⊕': '⊕',
'⊗': '⊗',
'⊥': '⊥',
'⋅': '⋅',
'⌈': '⌈',
'⌉': '⌉',
'⌊': '⌊',
'⌋': '⌋',
'〈': '〈',
'〉': '〉',
'◊': '◊',
'♠': '♠',
'♣': '♣',
'♥': '♥',
'♦': '♦',
'"': '"',
'&': '&',
'<': '<',
'>': '>',
''': ''',
'Œ': 'Œ',
'œ': 'œ',
'Š': 'Š',
'š': 'š',
'Ÿ': 'Ÿ',
'ˆ': 'ˆ',
'˜': '˜',
' ': ' ',
' ': ' ',
' ': ' ',
'': '',
'': '',
'': '',
'': '',
'–': '–',
'—': '—',
'‘': '‘',
'’': '’',
'‚': '‚',
'“': '“',
'”': '”',
'„': '„',
'†': '†',
'‡': '‡',
'‰': '‰',
'‹': '‹',
'›': '›',
'€': '€' ];
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy