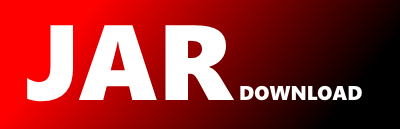
protoj.lang.ArchiveEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import java.io.FilenameFilter;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.apache.tools.ant.types.ZipFileSet;
import protoj.lang.internal.InformationException;
import protoj.util.ArgRunnable;
import protoj.util.AssembleTask;
/**
* Holds the information to be used when configuring an archive.
*
* @author Ashley Williams
*
*/
public final class ArchiveEntry {
/**
* See {@link #getManifest()}.
*/
private String manifest;
/**
* See {@link #getIncludes()}.
*/
private String includes;
/**
* See {@link #getExcludes()}.
*/
private String excludes;
/**
* See {@link #getConfig()}.
*/
private ArgRunnable config;
/**
* See {@link #getArtifact()}.
*/
private File artifact;
/**
* See {@link #getMergeArchives()}.
*/
private List mergeArchives;
/**
* See {@link #getParent()}.
*/
private ArchiveFeature parent;
/**
* See {@link #getName()}.
*/
private String name;
/**
* See the corresponding property accessors.
*
* @param name
* @param parent
* @param fileName
* @param manifest
* @param includes
* @param excludes
*/
public ArchiveEntry(String name, ArchiveFeature parent, String fileName,
String manifest, String includes, String excludes) {
this.name = name;
this.parent = parent;
this.mergeArchives = Collections.emptyList();
this.artifact = new File(parent.getProject().getLayout()
.getArchiveDir(), fileName);
this.manifest = manifest;
this.includes = includes;
this.excludes = excludes;
}
/**
* The specified config argument will be called back so that any last minute
* configuration can be applied.
*
* @param config
*/
public void initConfig(ArgRunnable config) {
this.config = config;
}
/**
* Any additional archives in the {@link ProjectLayout#getLibDir()}
* directory that should get merged during creation of the archive.
*
* @param archives
*/
public void initMergeArchives(String... archives) {
if (archives == null) {
mergeArchives = Collections.emptyList();
} else {
mergeArchives = Arrays.asList(archives);
}
}
/**
* Specifies that source archives in the {@link ProjectLayout#getLibDir()}
* that get past the specified filter should get merged during creation of
* the current archive.
*
* @param filter
*/
public void initMergeArchives(FilenameFilter filter) {
File libDir = parent.getProject().getLayout().getLibDir();
String[] archives = libDir.list(filter);
initMergeArchives(archives);
}
/**
* Returns a task that will create the archive in the
* {@link ProjectLayout#getArchiveDir()} directory. Any additional archives
* specified by {@link #getMergeArchives()} will also be merged into the
* final created jar file.
*
* @param assembleDir
* the base directory where files are to be included and excluded
* from
* @return
*/
public AssembleTask createAssembleTask(File assembleDir) {
ProjectLayout layout = parent.getProject().getLayout();
layout.getArchiveDir().mkdirs();
File manifestFile = getManifest() == null ? null : layout
.getManifest(getManifest());
AssembleTask assembleTask = new AssembleTask(getArtifact(),
assembleDir, manifestFile, getIncludes(), getExcludes());
assembleTask.initLogging();
List mergeArchives = getMergeArchives();
for (String archive : mergeArchives) {
File file = new File(layout.getLibDir(), archive);
if (!file.exists()) {
StringBuilder message = new StringBuilder();
message.append("merge failed: can't find file ");
message.append(file.getAbsolutePath());
message.append("\nwhile creating archive ");
message.append(getArtifact().getName());
throw new InformationException(message.toString());
}
ZipFileSet archiveSet = new ZipFileSet();
archiveSet.setSrc(file);
assembleTask.getJar().addFileset(archiveSet);
}
return assembleTask;
}
/**
* The archive file.
*
* @return
*/
public File getArtifact() {
return artifact;
}
/**
* The name of this archive entry without any file extention.
*
* @return
*/
public String getName() {
return name;
}
/**
* Used to specify any additional configuration.
*
* @return
*/
public ArgRunnable getConfig() {
return config;
}
/**
* The ant pattern used to specify the resources to be excluded from the
* archive.
*
* @return
*/
public String getExcludes() {
return excludes;
}
/**
* The ant pattern used to specify the resources to be included in the
* archive.
*
* @return
*/
public String getIncludes() {
return includes;
}
/**
* The name of the manifest file without the extension.
*
* @return
*/
public String getManifest() {
return manifest;
}
/**
* The parent parent.
*
* @return
*/
public ArchiveFeature getParent() {
return parent;
}
/**
* Those artifacts that are to be merged during artifact creation.
*
* @return
*/
public List getMergeArchives() {
return mergeArchives;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy