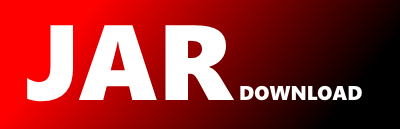
protoj.lang.DependencyInfo Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
/**
* Used to describe a dependency from a maven or ivy repository. Ideal for
* maintaining a list in your main project class and building up in your ivy or
* pom file using velocity.
*
* Can also be used to describe a dependency that isn't in the maven repository
* and is obtained by some other means. In this case just supply the name of the
* jar via the {@link #DependencyInfo(String, String)} constructor.
*
*
* @author Ashley Williams
*
*/
public final class DependencyInfo {
/**
* See {@link #getGroupId()}.
*/
private String groupId;
/**
* See {@link #getArtifactId()}.
*/
private String artifactId;
/**
* See {@link #getVersion()}.
*/
private String version;
/**
* See {@link #getJarName()}.
*/
private String jarName;
/**
* See {@link #isRepo()}.
*/
private boolean isRepo;
/**
* Convenience method for creating a dependency managed by a repository.
* Pass-thru to
* {@link #DependencyInfo(String, String, String, String, boolean)}.
*
* @param groupId
* @param artifactId
* @param version
*/
public DependencyInfo(String groupId, String artifactId, String version) {
this(groupId, artifactId, version, null, true);
}
/**
* Convenience method for creating a dependency managed in some way by the
* project. For example a dependency that is obtained from an internet
* download, rather than via a maven repository. Pass-thru to
* {@link #DependencyInfo(String, String, String, String, boolean)}.
*
* @param artifactId
* @param jarName
*/
public DependencyInfo(String artifactId, String jarName) {
this(null, artifactId, null, jarName, false);
}
/**
* Creates the information for maven repo or non maven repo managed an
* artifact.
*
* @param groupId
* the maven group id or the ivy org.
* @param artifactId
* the maven artifact id or the ivy name.
* @param version
* the maven version or the ivy revision.
* @param jarName
* the physical name of the jar including extension, mostly
* useful if the dependency isn't repository managed. Specify
* null for this to be calculated in the usual way from the
* artifact id and version.
* @param isRepo
* specify true if the dependency is repository managed, false
* otherwise
*/
public DependencyInfo(String groupId, String artifactId, String version,
String jarName, boolean isRepo) {
this.groupId = groupId;
this.artifactId = artifactId;
this.version = version;
this.jarName = jarName;
this.isRepo = isRepo;
}
/**
* See {@link #DependencyInfo(String, String, String)}.
*
* @return
*/
public String getGroupId() {
return groupId;
}
/**
* See {@link #DependencyInfo(String, String, String)}.
*
* @return
*/
public String getArtifactId() {
return artifactId;
}
/**
* See {@link #DependencyInfo(String, String, String)}.
*
* @return
*/
public String getVersion() {
return version;
}
/**
* A convenience method that returns the actual file name of the dependency.
* This is usually the concrete name of the jar file in the lib directory
* that can be different than the repository name, especially if the version
* number has been stripped off. If the jarName was not provided in the
* contructors then it defaults to the value returned by
* {@link #getVersionedJarName()}.
*
* @return
*/
public String getJarName() {
return jarName != null ? jarName : getVersionedJarName();
}
/**
* A convenience method that returns the file name of the dependency with
* the version.
*
* @return
*/
public String getVersionedJarName() {
return artifactId + "-" + version + ".jar";
}
/**
* A convenience method that returns the file name of the dependency without
* the version.
*
* @return
*/
public String getUnversionedJarName() {
return artifactId + ".jar";
}
/**
* True if this dependency describes an artifact in a repository, false if
* it is located by other means.
*
* @return
*/
public boolean isRepo() {
return isRepo;
}
/**
* Sometimes its necessary to update the information about where an artifact
* can be found. For example if it hasn't yet reached the repository at the
* time of release, but then can be found on a repository some time after.
* Or it could be that the artifact is pulled from the repository.
*
* @param isRepo
*/
public void setRepo(boolean isRepo) {
this.isRepo = isRepo;
}
}