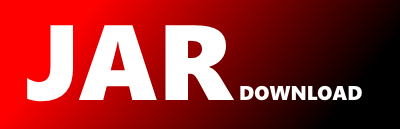
protoj.lang.DependencyStore Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
/**
* Holds the project maven and custom dependencies. If this class is used to
* store dependencies then maven and ivy xml files can include velocity markup
* in order to generate the dependency tags. That way copying and pasting
* dependency information between the java code and xml files is avoided.
*
* There are a number of dependencies out of the box that ProtoJ depends on. If
* you believe you don't use some of the use-cases implemented by one or more of
* the dependencies then remove them with a call to
* {@link #removeDependency(String)}. Also replace any if you have a conflicting
* version of the same library and would like to make a change.
*
* The {@link DependencyInfo} fields and getters are deliberately not javadoced
* as the comments would be repetitive.
*
* @author Ashley Williams
*
*/
public final class DependencyStore {
private HashMap dependencies = new HashMap();
private DependencyInfo beanUtils;
private DependencyInfo collections;
private DependencyInfo configuration;
private DependencyInfo io;
private DependencyInfo lang;
private DependencyInfo logging;
private DependencyInfo junit;
private DependencyInfo log4j;
private DependencyInfo jopt;
private DependencyInfo ant;
private DependencyInfo antLog4j;
private DependencyInfo antJunit;
private DependencyInfo antJsch;
private DependencyInfo antLauncher;
private DependencyInfo ivy;
private DependencyInfo mavenAntTasks;
private DependencyInfo velocity;
private DependencyInfo aspectjrt;
private DependencyInfo aspectjweaver;
private DependencyInfo aspectjtools;
private DependencyInfo googlecode;
private DependencyInfo jsch;
private DependencyInfo protoj;
private final StandardProject parent;
/**
* During the constructor call, the default ProtoJ dependencies are added.
*
* @param parent
*/
public DependencyStore(StandardProject parent) {
this.parent = parent;
beanUtils = addRepoDependency("commons-beanutils", "commons-beanutils",
"1.8.0");
collections = addRepoDependency("commons-collections",
"commons-collections", "3.2.1");
configuration = addRepoDependency("commons-configuration",
"commons-configuration", "1.6");
io = addRepoDependency("commons-io", "commons-io", "1.4");
lang = addRepoDependency("commons-lang", "commons-lang", "2.4");
logging = addRepoDependency("commons-logging", "commons-logging",
"1.1.1");
junit = addRepoDependency("junit", "junit", "4.5");
log4j = addRepoDependency("log4j", "log4j", "1.2.15");
jopt = addRepoDependency("net.sf.jopt-simple", "jopt-simple", "3.0");
ant = addRepoDependency("org.apache.ant", "ant", "1.7.1");
antLog4j = addRepoDependency("org.apache.ant", "ant-apache-log4j",
"1.7.1");
antJunit = addRepoDependency("org.apache.ant", "ant-junit", "1.7.1");
antJsch = addRepoDependency("org.apache.ant", "ant-jsch", "1.7.1");
antLauncher = addRepoDependency("org.apache.ant", "ant-launcher",
"1.7.1");
ivy = addRepoDependency("org.apache.ivy", "ivy", "2.0.0");
mavenAntTasks = addRepoDependency("org.apache.maven",
"maven-ant-tasks", "2.0.9");
velocity = addRepoDependency("org.apache.velocity", "velocity", "1.6.2");
protoj = addDependency("com.google.code.protoj", getProtojArtifactId(),
parent.getProtojVersion(), null, false);
aspectjrt = addCustomDependency("aspectjrt", "aspectjrt.jar");
aspectjweaver = addCustomDependency("aspectjweaver",
"aspectjweaver.jar");
aspectjtools = addCustomDependency("aspectjtools", "aspectjtools.jar");
googlecode = addCustomDependency("ant-googlecode",
"ant-googlecode-0.0.1.jar");
jsch = addCustomDependency("jsch", "jsch-0.1.41.jar");
}
/**
* Creates a DependencyInfo for a maven repo artifact and adds it to this
* store using the artifactId as a key. See
* {@link DependencyInfo#DependencyInfo(String, String, String)}.
*
* @param groupId
* @param artifactId
* @param version
* @return
*/
public DependencyInfo addRepoDependency(String groupId, String artifactId,
String version) {
DependencyInfo info = new DependencyInfo(groupId, artifactId, version);
dependencies.put(artifactId, info);
return info;
}
/**
* Creates a DependencyInfo for a non-maven repo artifact and adds it to
* this store using the artifactId as a key. See
* {@link DependencyInfo#DependencyInfo(String, String)}.
*
* @param artifactId
* @param jarName
* @return
*/
public DependencyInfo addCustomDependency(String artifactId, String jarName) {
DependencyInfo info = new DependencyInfo(artifactId, jarName);
dependencies.put(artifactId, info);
return info;
}
/**
* Creates a DependencyInfo for a maven or non-maven repo artifact and adds
* it to this store using the artifactId as a key. See
* {@link DependencyInfo#DependencyInfo(String, String, String, String, boolean)}
*
* @param groupId
* @param artifactId
* @param version
* @param jarName
* @param isRepo
* @return
*/
public DependencyInfo addDependency(String groupId, String artifactId,
String version, String jarName, boolean isRepo) {
DependencyInfo info = new DependencyInfo(groupId, artifactId, version,
jarName, isRepo);
dependencies.put(artifactId, info);
return info;
}
/**
* Removes the dependency with the specified artifactId.
*
* @param artifactId
* @return
*/
public DependencyInfo removeDependency(String artifactId) {
return dependencies.remove(artifactId);
}
/**
* Removes the specified dependency.
*
* @param info
* @return
*/
public DependencyInfo removeDependency(DependencyInfo info) {
return removeDependency(info.getArtifactId());
}
/**
* Looks up the info with the specified artifactId.
*
* @param artifactId
* @return
*/
public DependencyInfo getDependency(String artifactId) {
return dependencies.get(artifactId);
}
/**
* Returns the sub-collection of dependencies that are managed by a maven
* repo. Useful for referring to in a maven or ivy xml file with a piece of
* velocity script in order to build up the dependency tags.
*
* @return
*/
public Collection getRepoDependencies() {
ArrayList resultInfos = new ArrayList();
Collection allInfos = dependencies.values();
for (DependencyInfo info : allInfos) {
if (info.isRepo()) {
resultInfos.add(info);
}
}
return resultInfos;
}
/**
* Returns the sub-collection of dependencies that are not managed by a
* maven repo.
*
* @return
*/
public Collection getCustomDependencies() {
ArrayList resultInfos = new ArrayList();
Collection allInfos = dependencies.values();
for (DependencyInfo info : allInfos) {
if (!info.isRepo()) {
resultInfos.add(info);
}
}
return resultInfos;
}
/**
* Gets all the maven and non-maven dependencies in this
*
* @return
*/
public Collection getDependencies() {
return dependencies.values();
}
public DependencyInfo getBeanUtils() {
return beanUtils;
}
public DependencyInfo getCollections() {
return collections;
}
public DependencyInfo getConfiguration() {
return configuration;
}
public DependencyInfo getIo() {
return io;
}
public DependencyInfo getLang() {
return lang;
}
public DependencyInfo getLogging() {
return logging;
}
public DependencyInfo getJunit() {
return junit;
}
public DependencyInfo getLog4j() {
return log4j;
}
public DependencyInfo getJopt() {
return jopt;
}
public DependencyInfo getAnt() {
return ant;
}
public DependencyInfo getAntLog4j() {
return antLog4j;
}
public DependencyInfo getAntJunit() {
return antJunit;
}
public DependencyInfo getAntJsch() {
return antJsch;
}
public DependencyInfo getAntLauncher() {
return antLauncher;
}
public DependencyInfo getIvy() {
return ivy;
}
public DependencyInfo getMavenAntTasks() {
return mavenAntTasks;
}
public DependencyInfo getVelocity() {
return velocity;
}
public DependencyInfo getAspectjrt() {
return aspectjrt;
}
public DependencyInfo getAspectjweaver() {
return aspectjweaver;
}
public DependencyInfo getAspectjtools() {
return aspectjtools;
}
public DependencyInfo getGooglecode() {
return googlecode;
}
public DependencyInfo getJsch() {
return jsch;
}
public DependencyInfo getProtoj() {
return protoj;
}
/**
* The protoj artifact id depends on the version of java running.
*
* @return
*/
public String getProtojArtifactId() {
String tag;
if (parent.isJdk6()) {
tag = "protoj-jdk6";
} else {
tag = "protoj-jdk5";
}
return tag;
}
}