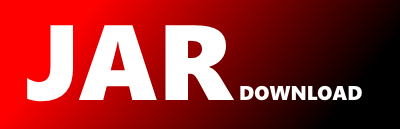
protoj.lang.IvyFeature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import org.apache.ivy.ant.IvyFindRevision;
import org.apache.ivy.ant.IvyListModules;
import org.apache.ivy.ant.IvyMakePom;
import org.apache.ivy.ant.IvyMakePom.Mapping;
import org.apache.tools.ant.Project;
import protoj.util.AntTarget;
/**
* All ProtoJ ivy functionality.
*
* @author Ashley Williams
*
*/
public final class IvyFeature {
private String organization;
private String module;
private String revision;
private String revisionResult;
private String branch;
private String settingsRef;
private String matcher;
private String value;
/**
* See {@link #getIvyFile()}.
*/
private File ivyFile;
/**
* See {@link #getPomFile()}.
*/
private File pomFile;
public IvyFeature(StandardProject parent) {
this.ivyFile = new File(parent.getLayout().getConfDir(), "ivy.xml");
this.pomFile = new File(parent.getLayout().getTargetDir(), "pom.xml");
}
/**
* Pass-thru method to the ivy listmodules ant task. Consult the
* documentation on the ivy website. TODO I haven't been able to get
* ivy.listmodules to produce any results so can't continue with this just
* yet.
*
* @param organization
* @param module
* @param branch
* @param revision
* @param matcher
* @param value
* @param settingsRef
*/
public void listmodules(String organization, String module, String branch,
String revision, String matcher, String value, String settingsRef) {
this.organization = organization;
this.module = module;
this.branch = branch;
this.revision = revision;
this.matcher = matcher;
this.value = value;
this.settingsRef = settingsRef;
AntTarget target = new AntTarget("ivy-feature");
target.initLogging(Project.MSG_INFO);
IvyListModules listmodules = new IvyListModules();
target.addTask(listmodules);
listmodules.setTaskName("findrevision");
if (organization != null) {
listmodules.setOrganisation(organization);
}
if (module != null) {
listmodules.setModule(module);
}
if (branch != null) {
listmodules.setBranch(branch);
}
if (revision != null) {
listmodules.setRevision(revision);
}
if (matcher != null) {
listmodules.setMatcher(matcher);
}
if (value != null) {
listmodules.setValue(value);
}
// listmodules.setSettingsRef(settingsRef);
String string = "modules.[module]";
listmodules.setProperty(string);
// System.out.println(listmodules.getSettingsRef().getRefId());
target.execute();
System.out.println(target.getProject().getProperty(string));
}
/**
* Pass-thru method to the ivy findrevision ant task. Consult the
* documentation on the ivy website.
*
* @param organization
* @param module
* @param branch
* @param revision
* @param settingsRef
*/
public void findrevision(String organization, String module, String branch,
String revision, String settingsRef) {
this.organization = organization;
this.module = module;
this.branch = branch;
this.revision = revision;
this.settingsRef = settingsRef;
AntTarget target = new AntTarget("ivy-feature");
target.initLogging(Project.MSG_INFO);
IvyFindRevision findrevision = new IvyFindRevision();
target.addTask(findrevision);
findrevision.setTaskName("findrevision");
if (organization != null) {
findrevision.setOrganisation(organization);
}
if (module != null) {
findrevision.setModule(module);
}
if (branch != null) {
findrevision.setBranch(branch);
}
if (revision != null) {
findrevision.setRevision(revision);
}
// findrevision.setSettingsRef(settingsRef);
target.execute();
revisionResult = target.getProject().getProperty(
findrevision.getProperty());
}
/**
* Decorates the ivy makepom ant task. The ivy.xml file under the conf
* directory is used as the source and a pom.xml file is created in the
* target directory. Ivy dependencies will map to either the maven compile
* scope or option scope depending on whether or not they are associated
* with an ivy config element called "compile".
*
* @param settingsRef
*/
public void makepom(String settingsRef) {
AntTarget target = new AntTarget("ivy-feature");
target.initLogging(Project.MSG_INFO);
IvyMakePom makepom = new IvyMakePom();
target.addTask(makepom);
makepom.setTaskName("makepom");
makepom.setIvyFile(ivyFile);
makepom.setPomFile(pomFile);
Mapping mapping = makepom.createMapping();
mapping.setConf("compile");
mapping.setScope("compile");
target.execute();
}
public String getOrganization() {
return organization;
}
public String getModule() {
return module;
}
public String getRevision() {
return revision;
}
public String getBranch() {
return branch;
}
public String getSettingsRef() {
return settingsRef;
}
public String getMatcher() {
return matcher;
}
public String getValue() {
return value;
}
/**
* Stores the result of the most recent invocation of
* {@link #findrevision(String, String, String, String, String)}. May be
* null if the search criteria resulted in no matching revision.
*
* @return
*/
public String getRevisionResult() {
return revisionResult;
}
/**
* The ivy file used to define project dependencies.
*
* @return
*/
public File getIvyFile() {
return ivyFile;
}
/**
* The pom file that gets generated from the ivy file after the call to
* {@link #makepom(String)}.
*
* @return
*/
public File getPomFile() {
return pomFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy