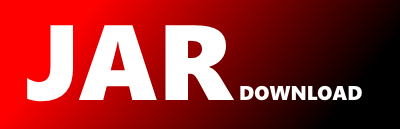
protoj.lang.JavadocArchive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import java.util.Collection;
import java.util.Set;
import java.util.TreeMap;
import org.apache.commons.io.FileUtils;
import org.apache.tools.ant.types.DirSet;
import protoj.lang.internal.InformationException;
import protoj.util.ArgRunnable;
import protoj.util.AssembleTask;
import protoj.util.JavadocTask;
/**
* Responsible for creating one or more archives from the parent javadoc files.
* Call {@link #addEntry(String, String, String, String, String)} to configure
* each additional archive with the specified name and
* {@link #createArchive(String)} to create one of the added archive. During
* creation, the config
argument specified in the constructor will
* be called back to give the caller a chance to provide further archive
* configuration.
*
* @author Ashley Williams
*
*/
public final class JavadocArchive {
/**
* Adds javadoc specific information to a wrapped {@link ArchiveEntry}
* instance.
*
* @author Ashley Williams
*
*/
public static final class JavadocEntry {
/**
* See {@link #getArchiveEntry()}.
*/
private ArchiveEntry archiveEntry;
/**
* See {@link #getMemory()}.
*/
private String memory;
/**
* See the {@link ArchiveEntry} accessors.
*
* @param parent
* @param name
* @param fileName
* @param manifest
* @param includes
* @param excludes
*/
public JavadocEntry(ArchiveFeature parent, String name,
String fileName, String manifest, String includes,
String excludes, String memory) {
this.archiveEntry = new ArchiveEntry(name, parent,
fileName, manifest, includes, excludes);
this.memory = memory;
}
/**
* Pass-thru method to {@link ArchiveEntry#initConfig(ArgRunnable)}.
*
* @param config
*/
public void initConfig(ArgRunnable config) {
archiveEntry.initConfig(config);
}
/**
* The maximum amount of memory that the javadoc vm should allocate.
*
* @return
*/
public String getMemory() {
return memory;
}
/**
* The wrapped helper.
*
* @return
*/
public ArchiveEntry getArchiveEntry() {
return archiveEntry;
}
}
/**
* The parent that owns this instance lifecycle.
*/
private final ArchiveFeature parent;
/**
* The configuration information for each added archive.
*/
private TreeMap entries = new TreeMap();
/**
* See {@link #getCurrentAssembleTask()}.
*/
private AssembleTask currentAssembleTask;
/**
* See {@link #getCurrentEntry()}.
*/
private ArchiveEntry currentEntry;
/**
* See {@link #getWorkingDir()}.
*/
private File workingDir;
/**
* See {@link #getJavadocTask()}.
*/
private JavadocTask javadocTask;
/**
* Creates with the parent feature.
*
* @param parent
*/
public JavadocArchive(ArchiveFeature parent) {
this.parent = parent;
this.workingDir = new File(parent.getProject().getLayout()
.getArchiveDir(), "javadoc-archive");
}
/**
* Use to enable creation of an additional archive.
*
* @param name
* the name of the jar file to be created, without the extension.
* @param manifest
* the name of the manifest to be used from the manifest
* directory, without the extension. Can be null if a default
* manifest is required.
* @param includes
* the resources that should be included in the archive, can be
* null to include all resources
* @param excludes
* the resources that should be excluded in the archive, can be
* null to exclude no resources
* @param memory
* the maximum amount of memory to be used by the javadoc vm, eg
* "16m"
*/
public void addEntry(String name, String manifest, String includes,
String excludes, String memory) {
if (memory == null) {
throw new InformationException(
"please specify a non null value for javadoc memory");
}
String fileName = String.format("%s-%s.jar", name, parent.getLayout()
.getJavadocPostfix());
JavadocEntry entry = new JavadocEntry(parent, name, fileName, manifest,
includes, excludes, memory);
entries.put(name, entry);
}
/**
* Delegates to {@link #createArchive(String)} for each archive that was
* added through a call to
* {@link #addEntry(String, String, String, String, String)}.
*/
public void createArchives() {
Set keys = entries.keySet();
for (String key : keys) {
createArchive(key);
}
}
/**
* Creates the jar for the given name under
* {@link ProjectLayout#getArchiveDir()}. The jar file will have a name of
* [name]-javadoc.jar.
*
* @param name
* the name as specified in the call to
* {@link #addEntry(String, String, String, String, String)} .
*/
public void createArchive(String name) {
ProjectLayout layout = parent.getProject().getLayout();
// creates javadocs in working directory
getWorkingDir().mkdirs();
JavadocEntry entry = entries.get(name);
String memory = entry.getMemory();
File srcDir = layout.getJavaDir();
javadocTask = new JavadocTask(srcDir, getWorkingDir());
javadocTask.initLogging();
javadocTask.getjavadoc().setMaxmemory(memory);
DirSet packageSet = javadocTask.getPackageSet();
packageSet.setDefaultexcludes(true);
ArgRunnable config = entry.getArchiveEntry()
.getConfig();
currentAssembleTask = entry.getArchiveEntry().createAssembleTask(
getWorkingDir());
if (config != null) {
config.run(this);
}
javadocTask.execute();
currentAssembleTask.execute();
FileUtils.deleteDirectory(getWorkingDir());
}
/**
* Convenient method that visits each entry in this archive.
*
* @param visitor
*/
public void visit(ArgRunnable visitor) {
Collection values = entries.values();
for (JavadocEntry entry : values) {
visitor.run(entry);
}
}
/**
* Accessor for the entry corresponding to the given name.
*
* @param name
* @return
*/
public JavadocEntry getEntry(String name) {
return entries.get(name);
}
/**
* The ant task responsible for creating the javadocs during the call to
* {@link #createArchive(String)}.
*
* @return
*/
public JavadocTask getJavadocTask() {
return javadocTask;
}
/**
* Used to create the javadoc files before archiving.
*
* @return
*/
public File getWorkingDir() {
return workingDir;
}
/**
* The ant task responsible for creating the jar during the call to
* {@link #createArchive(String)}.
*
* @return
*/
public AssembleTask getCurrentAssembleTask() {
return currentAssembleTask;
}
/**
* The instance used to hold information about the the archive being created
* during the call to {@link #createArchive(String)}.
*
* @return
*/
public ArchiveEntry getCurrentEntry() {
return currentEntry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy