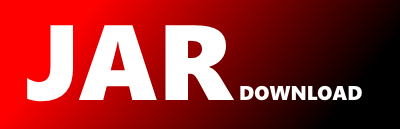
protoj.lang.ProjectArchive Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import org.apache.tools.ant.taskdefs.Tar;
import protoj.util.ArgRunnable;
import protoj.util.TarTask;
/**
* Provides support for creating a compressed tar of the entire project. If
* further configuration is required, such as adding or excluding directories,
* then supply an appropriate config argument to the constructor below. In the
* following example, an extra directory called "exampledir" gets included in
* the mytar tar.gz file:
*
*
* config = new ArgRunnable<ProjectArchive>() {
* public void configure(ProjectArchive feature) {
* feature.addFileSet("777", "777", "exampledir/**", null);
* }
* };
* tarFeature = new ProjectArchive(this, name, userName, group, config);
*
*
* Ant tasks can only be used once and cannot be reconfigured for a second time.
* And so the solution is to place the configuration (the api calls) in a
* runnable as in the above example so that it can be stored. The
* {@link #createArchive(boolean, boolean, boolean)} method then creates a brand
* new ant tar task every time, but passes it through the runnable that was
* supplied to the constructor so that it can be configured correctly.
*
* @author Ashley Williams
*
*/
public final class ProjectArchive {
/**
* See {@link #getParent()}.
*/
private ArchiveFeature parent;
/**
* See {@link #getUserName()}.
*/
private String userName;
/**
* See {@link #getGroup()}.
*/
private String group;
/**
* See {@link #isNoSrc()}.
*/
private boolean noSrc;
/**
* See {@link #isNoClasses()}.
*/
private boolean noClasses;
/**
* See {@link #isGlobalRwx()}.
*/
private boolean isGlobalRwx;
/**
* See {@link #getAntTar()}.
*/
private TarTask tarTask;
/**
* Responsible for additional tar configuration - see {@link ProjectArchive}
* .
*/
private ArgRunnable config;
/**
* See {@link #getPrefix()}.
*/
private final String prefix;
/**
* The portion of the tar file without any extention.
*/
private File tarStem;
/**
* A cache reference to this extensively used object.
*/
private ProjectLayout layout;
/**
* The individual property getter methods describe the parameters of the
* same name. See {@link ProjectArchive} for more explanation of the
* ArgRunnable
parameter.
*
* @param parent
* @param name
* @param prefix
* @param userName
* @param group
*/
public ProjectArchive(ArchiveFeature parent, String name, String prefix,
String userName, String group) {
this.parent = parent;
this.layout = parent.getProject().getLayout();
this.tarStem = new File(layout.getArchiveDir(), name);
this.prefix = prefix;
this.userName = userName;
this.group = group;
}
/**
* The specified config argument will be called back so that any last minute
* configuration can be applied.
*
* @param config
*/
public void initConfig(ArgRunnable config) {
this.config = config;
}
/**
* The parent of this feature.
*
* @return
*/
public ArchiveFeature getParent() {
return parent;
}
/**
* The username for the tar entries.
*
* @return
*/
public String getUserName() {
return userName;
}
/**
* The groupname for the tar entries.
*
* @return
*/
public String getGroup() {
return group;
}
/**
* The name to be used in the tar file before any prefix or suffix
* decoration or file extensions have been applied.
*
* @return
*/
public String getName() {
return tarStem.getName();
}
/**
* Often an application installation directory has additional business
* requirements such as the inclusion of a version number, customer product
* identifier and so on. The prefix enforces this since it is used as the
* prefix for every entry in the tar file.
*
* @return
*/
public String getPrefix() {
return prefix;
}
/**
* Whether or not to include the source directory in the tar.
*
* @return
*/
public boolean isNoSrc() {
return noSrc;
}
/**
* Whether or not to include the classes directory in the tar.
*
* @return
*/
public boolean isNoClasses() {
return noClasses;
}
/**
* If this is true then permissions are set to rwx on all files and
* directories in the tar, overriding any original configuration.
*
* @return
*/
public boolean isGlobalRwx() {
return isGlobalRwx;
}
/**
* The underlying ant object, which can be used for further customization.
*
* @return
*/
public Tar getAntTar() {
return tarTask.getTar();
}
/**
* Returns the full path name of the uncompressed tar file, eg
* /a/b/c/foo.tar.
*
* @return
*/
public String getUncompressedPath() {
return tarStem.getAbsolutePath() + ".tar";
}
/**
* Returns the full path name of the compressed tar file, eg
* /a/b/c/foo.tar.gz.
*
* @return
*/
public String getArchivePath() {
return getUncompressedPath() + ".gz";
}
/**
* The file instance corresponding to {@link #getArchivePath()}.
*
* @return
*/
public File getArchiveFile() {
return new File(getArchivePath());
}
/**
* Adds the given fileset definition to the underlying tar ant task. The
* pattern entries should be specified relative to the parent root
* directory. Reminder: read=4, write=2, execute=1. Note that if
* {@link #isGlobalRwx()} is set to true then the fileMode and dirMode
* parameters are ignored in favor of the most relaxed rwx permissions.
*
* @param fileMode
* @param dirMode
* @param includes
* @param excludes
*/
public void addFileSet(String fileMode, String dirMode, String includes,
String excludes) {
if (isGlobalRwx()) {
fileMode = "777";
dirMode = "777";
}
File rootDir = layout.getRootDir();
tarTask.addFileSet(rootDir, getPrefix(), fileMode, dirMode, userName,
group, includes, excludes);
}
/**
* Creates a tar of the parent in the target directory. First a brand new
* ant tar task is created. Then it is configured with the defaults - see
* {@link #initTarTask()}. Finally it is configured by applying the
* {@link #config} strategy and then executed.
*
* @param noSrc
* true in order to exclude the src directory, false otherwise.
* @param noClasses
* true in order to exclude the classes directory, false
* otherwise.
* @param isGlobalRwx
* true to override preconfigured permissions with rwx, false to
* leave alone
*/
public void createArchive(boolean noSrc, boolean noClasses,
boolean isGlobalRwx) {
File archiveDir = layout.getArchiveDir();
archiveDir.mkdirs();
this.noSrc = noSrc;
this.noClasses = noClasses;
this.isGlobalRwx = isGlobalRwx;
this.tarTask = new TarTask(tarStem.getParent(), tarStem.getName());
initTarTask();
if (config != null) {
config.run(this);
}
this.tarTask.execute();
}
/**
* Provides the tar configuration that is always present, namely that the
* bin, classes, conf, docs, lib and log directories always get included in
* the tar file. Additionally the log file always gets excluded as do any
* javadoc and source jar files under the lib directory. The source
* directory will get included unless {@link #isNoSrc()} returns true. The
* classes directory will get included unless {@link #isNoClasses()} returns
* true.
*/
private void initTarTask() {
File logFile = layout.getLogFile();
tarTask.initLogging();
addFileSet("544", "755", layout.getBinDir().getName() + "/**", null);
addFileSet("444", "755", layout.getConfDir().getName() + "/**",
getConfExcludesPattern());
addFileSet("444", "755", layout.getDocsDir().getName() + "/**", null);
addFileSet("444", "755", layout.getLibDir().getName() + "/**",
getLibExcludesPattern());
String excludeLogFile = getLogExcludesPattern(logFile);
addFileSet("644", "755", layout.getLogDir().getName() + "/**",
excludeLogFile);
if (!noSrc) {
addFileSet("444", "755", layout.getSrcDir().getName() + "/**", null);
}
if (!noClasses) {
addFileSet("444", "755", layout.getClassesDir().getName() + "/**",
null);
}
}
/**
* The pattern used to filter out the protoj log file from the log
* directory.
*
* @return
*/
public String getLogExcludesPattern(File logFile) {
return logFile.getParentFile().getName() + "/" + logFile.getName();
}
/**
* The pattern used to filter out properties files from directly under the
* conf directory.
*
* @return
*/
public String getConfExcludesPattern() {
String confDirName = layout.getConfDir().getName();
return confDirName + "/*.properties";
}
/**
* The pattern used to filter out source and javadoc jar files from directly
* under the lib directory.
*
* @return
*/
public String getLibExcludesPattern() {
String libDirName = layout.getLibDir().getName();
return String
.format("%s/*%s.jar %s/*%s.jar %s/*%s.jar", libDirName, layout
.getJavadocPostfix(), libDirName, layout
.getSourcePostfix(), libDirName, layout.getSrcPostfix());
}
}