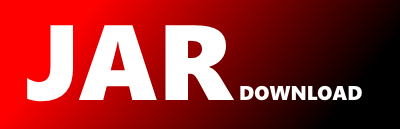
protoj.lang.PropertyInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
/**
* Represents a property in the {@link PropertyStore}. Where there is no fully
* defined property available in the PropertyStore each PropertyInfo instance
* contains defaults to be used instead.
*
* @author Ashley Williams
*
*/
public final class PropertyInfo {
/**
* see {@link #getKey()}()
*/
private String key;
/**
* see {@link #getEmptyDefault()}()
*/
private String emptyDefault;
/**
* see {@link #getMissingDefault()}()
*/
private String missingDefault;
/**
* See {@link #getDescription}.
*/
private String description;
/**
* The parent property store that this property belongs to.
*/
private final PropertyStore store;
/**
* See the corresponding property descriptions.
*
* @param store
* @param key
* @param missingDefault
* @param emptyDefault
* @param description
*/
public PropertyInfo(PropertyStore store, String key, String missingDefault,
String emptyDefault, String description) {
this.store = store;
this.key = key;
this.missingDefault = missingDefault;
this.emptyDefault = emptyDefault;
this.description = description;
}
/**
* The property key.
*
* @return
*/
public String getKey() {
return key;
}
/**
* Provides a value for the underlying key in all cases:
*
* - Where a value is present, that value is used. For example:
*
-Dmyproperty=myvalue
* - Where there is no property with the given
key
,
* missingDefault
is used.
* - Where there is a property with the given
key
but an
* empty value is provided, emptyDefault
is used. For example:
* -Dmyproperty
or -Dmyproperty=
*
*
* @return
*/
public String getValue() {
String value = store.getConfig().getString(key, missingDefault);
if (value.equals("")) {
value = emptyDefault;
}
return value;
}
/**
* Gets the value for the given info throwing an exception if the result is
* null. Convenience wrapper for {@link #getValue()}.
*
* @return
*/
public String getMandatoryValue() {
String value = getValue();
if (value.length() == 0) {
String message = "please provide a value for the property "
+ getKey();
throw new RuntimeException(message);
}
return value;
}
/**
* Conveniently typed method for converting {@link #getValue()}.
*
* @return
*/
public boolean getBooleanValue() {
String value = getValue();
return value != null ? Boolean.parseBoolean(value) : null;
}
/**
* Conveniently typed method for converting {@link #getValue()}.
*
* @return
*/
public int getIntegerValue() {
String value = getValue();
return value != null ? Integer.parseInt(value) : null;
}
/**
* The default value to use when the value of a property is empty. For
* example: -Dmyproperty
or -Dmyproperty=
*
* @return
*/
public String getEmptyDefault() {
return emptyDefault;
}
/**
* The default value to use when a property isn't specified at all.
*
* @return
*/
public String getMissingDefault() {
return missingDefault;
}
/**
* A description useful for help text.
*
* @return
*/
public String getDescription() {
return description;
}
/**
* Creates a command line equivalent of this property with the specified
* value. Convenient for writing test cases.
*
* @param value
* can be null for a property with no value
* @return
*/
public String createJvmArg(String value) {
StringBuilder builder = new StringBuilder();
builder.append("-D");
builder.append(getKey());
if (value != null) {
builder.append("=");
builder.append(value);
}
return builder.toString();
}
/**
* Builds a message suitable for displaying in response to a help command.
*
* @return
*/
public String getHelpText() {
StringBuilder builder = new StringBuilder();
builder.append("Name: ");
builder.append(getKey());
builder.append("\n\n");
builder.append(getDescription());
builder
.append("\n\nWhen the key is not specified the application assumes a value of '");
builder.append(getMissingDefault());
builder.append("'");
builder
.append("\nWhen the key is specified, but not assigned a value, the application assumes a value of '");
builder.append(getEmptyDefault());
builder.append("'");
builder.append("\n");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy