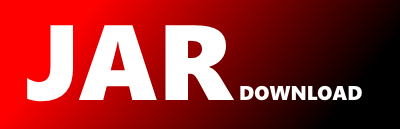
protoj.lang.PropertyStore Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import java.io.FilenameFilter;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import org.apache.commons.configuration.CompositeConfiguration;
import org.apache.commons.configuration.Configuration;
import org.apache.commons.configuration.PropertiesConfiguration;
import org.apache.commons.configuration.SystemConfiguration;
/**
* Responsible for managing the available infos. The property values can be
* obtained simply by querying the reference returned by {@link #getConfig()}.
*
* Alternatively consider calling {@link PropertyInfo#getValue()}, which
* provides for customized default behavior. These info objects can be added by
* calling {@link #addInfo(String, String, String, String)} and are used for
* example in ProtoJ help.
*
* @author Ashley Williams
*
*/
public final class PropertyStore {
/**
* See {@link #getCommands()}.
*/
private HashMap infos = new HashMap();
/**
* The directory where the infos files are stored.
*/
private File confDir;
/**
* See {@link #getConfig()}.
*/
private Configuration config;
/**
* Creates an instance with the owning project.
*
* @param parent
*/
public PropertyStore(StandardProject parent) {
this.confDir = parent.getLayout().getConfDir();
this.config = createConfig();
}
/**
* Creates a infos helper that is configured to look for property values
* first from any infos files immediately under the config directory and
* secondly from java.lang.System.
*
* @return
*/
@SuppressWarnings("unchecked")
private Configuration createConfig() {
final CompositeConfiguration config = new CompositeConfiguration();
confDir.listFiles(new FilenameFilter() {
public boolean accept(File dir, String name) {
if (name.endsWith(".properties")) {
File file = new File(dir, name);
config.addConfiguration(new PropertiesConfiguration(file));
}
return true;
}
});
config.addConfiguration(new SystemConfiguration());
return config.interpolatedConfiguration();
}
/**
* Creates a {@link PropertyInfo} instance with the given arguments and adds
* it to this store.
*
* @param key
* the key the property key
* @param missingDefault
* the non null value that will be returned when no property is
* found with the specified key
* @param emptyDefault
* the non null value that will be returned when a property is
* found with the specified key, but it has no value. For example
* "-Dmyprop=" or "-Dmyprop"
* @param description
* a description that will appear in the help for the property
* @return
*/
public PropertyInfo addInfo(String key, String missingDefault,
String emptyDefault, String description) {
PropertyInfo info = new PropertyInfo(this, key, missingDefault,
emptyDefault, description);
infos.put(key, info);
return info;
}
/**
* Convenience method that delegates to
* {@link #addInfo(String, String, String, String)} with empty default
* values.
*
* @param key
* @param description
* @return
*/
public PropertyInfo addInfo(String key, String description) {
return addInfo(key, "", "", description);
}
/**
* Read-only access to the added {@link PropertyInfo} instances.
*
* @return
*/
public Collection getInfos() {
return Collections.unmodifiableCollection(infos.values());
}
/**
* Looks up the {@link PropertyInfo} with the given key.
*
* @param key
* @return
*/
public PropertyInfo getInfo(String key) {
return infos.get(key);
}
/**
* If the {@link PropertyInfo} with the given key isn't found then it is
* added. Useful for properties whose keys aren't known until runtime - for
* an example see
* {@link StandardProperties#getCommandMemory(String)}. For a
* parameter description see
* {@link #addInfo(String, String, String, String)}.
*
* @param key
* @param description
* @param missingDefault
* @param emptyDefault
* @return
*/
public PropertyInfo getLazyInfo(String key, String description,
String missingDefault, String emptyDefault) {
PropertyInfo info = getInfo(key);
if (info == null) {
info = addInfo(key, missingDefault, emptyDefault, description);
}
return info;
}
/**
* The instance responsible for obtaining property values. See
* {@link #createConfig()}.
*
* @return
*/
public Configuration getConfig() {
return config;
}
}