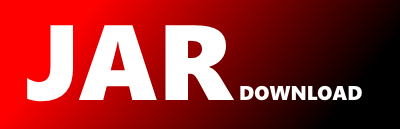
protoj.lang.RetrieveFeature Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import org.apache.ivy.ant.IvyRetrieve;
import org.apache.maven.artifact.ant.DependenciesTask;
import org.apache.maven.artifact.ant.Pom;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.Copy;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.Mapper;
import org.apache.tools.ant.types.Reference;
import org.apache.tools.ant.types.Mapper.MapperType;
import protoj.util.AntTarget;
import protoj.util.ArgRunnable;
/**
* Retrieves dependency artifacts and places them in the project lib directory.
* By default ivy is used to perform the download and requires an ivy xml file
* to be placed on the classpath that describes the project dependencies.
*
* Alternatively maven can be used to perform the download, which requires a
* maven pom file to be placed on the classpath that describes the project
* dependencies.
*
* When choosing between ivy and maven, be aware that transitive dependency
* downloading can be turned off with ivy but not with maven.
*
* @author Ashley Williams
*
*/
public final class RetrieveFeature {
/**
* See {@link #getParent()}.
*/
private final StandardProject parent;
/**
* See {@link #getDependencies()}.
*/
private DependenciesTask dependencies;
/**
* See {@link #getCopyClasses()}.
*/
private Copy copyClasses;
/**
* See {@link #getCopyJavadocs()}.
*/
private Copy copyJavadocs;
/**
* See {@link #getCopySources()}.
*/
private Copy copySources;
/**
* The instance used for additional feature configuration.
*/
private ArgRunnable config;
/**
* See {@link #getIvyRetrieve()}.
*/
private IvyRetrieve ivyRetrieve;
/**
* See {@link #getIvyFile()}.
*/
private File ivyFile;
/**
* See {@link #getMavenFile()}.
*/
private File mavenFile;
/**
* See {@link #getWorkingDir()}.
*/
private File workingDir;
/**
* The resource path to the ivy xml file.
*/
private String ivyConfig;
/**
* The resource path to the maven pom file.
*/
private String mavenConfig;
/**
* Creates with the owning parent.
*
* @param parent
*/
public RetrieveFeature(StandardProject parent) {
this.parent = parent;
}
/**
* Configures with the classpath location of the maven config file.
*
* @param mavenConfig
* the resource path of the maven config file, for example
* "/acme/pom.xml"
*/
public void initMaven(String mavenConfig) {
this.mavenConfig = mavenConfig;
}
/**
* Configures with the classpath location of the ivy configuration file.
*
* @param ivyConfig
* the resource path of the ivy config file, for example
* "/acme/ivy.xml"
*/
public void initIvy(String ivyConfig) {
this.ivyConfig = ivyConfig;
}
/**
* Add a configuration instance to this feature.
*
* @param config
* the instance that gets called back when
* {@link #mavenRetrieve()} is invoked so that further
* configuration of this feature may be applied.
*/
public void initConfig(ArgRunnable config) {
this.config = config;
}
/**
* Calls both the {@link #ivyRetrieve()} and {@link #mavenRetrieve()}
* methods in succession.
*/
public void retrieveAll() {
ivyRetrieve();
mavenRetrieve();
}
/**
* Downloads the dependencies described in the project ivy.xml file under
* the conf directory to the lib directory. Just before execution of the ant
* task, any configuration provided via a call to
* {@link #initConfig(ArgRunnable)} will be called back so that further
* configuration of this feature may be applied.
*
* Hint: use this ivy based method when you don't want transitive
* dependencies since there doesn't seem to be a way to disable this when
* using maven.
*/
public void ivyRetrieve() {
if (ivyConfig != null) {
AntTarget target = new AntTarget("ivy-feature");
target.getProject().setBaseDir(parent.getLayout().getRootDir());
target.initLogging(Project.MSG_INFO);
ivyRetrieve = new IvyRetrieve();
target.addTask(ivyRetrieve);
ivyRetrieve.setTaskName("ivyRetrieve");
ivyRetrieve.setFile(getIvyFile());
if (config != null) {
config.run(this);
}
target.execute();
}
}
/**
* Downloads the dependencies described in the project pom.xml file under
* the conf directory to the lib directory. Just before execution of the ant
* task, any configuration provided via a call to
* {@link #initConfig(ArgRunnable)} will be called back so that further
* configuration of this feature may be applied.
*/
public void mavenRetrieve() {
if (mavenConfig != null) {
AntTarget target = new AntTarget("maven-feature");
target.initLogging(Project.MSG_INFO);
dependencies = createDependencies(target);
String classesDependency = "classes.fileset";
dependencies.setFilesetId(classesDependency);
copyClasses = addDependency(target, classesDependency);
String javadocsDependency = "javadocs.fileset";
dependencies.setJavadocFilesetId(javadocsDependency);
copyJavadocs = addDependency(target, javadocsDependency);
String sourcesDependency = "sources.fileset";
dependencies.setSourcesFilesetId(sourcesDependency);
copySources = addDependency(target, sourcesDependency);
if (config != null) {
config.run(this);
}
target.execute();
}
}
/**
* Sets up the basic maven dependencies task.
*
* @param target
* @return
*/
private DependenciesTask createDependencies(AntTarget target) {
// point to the project pom.xml file in the conf directory
Pom pom = new Pom();
pom.setTaskName("maven-feature-pom");
target.addTask(pom);
pom.setId("protoj.project");
pom.setFile(getMavenFile());
// assign the pom to a new dependencies task
DependenciesTask dependencies = new DependenciesTask();
dependencies.setTaskName("maven-feature-dependencies");
target.addTask(dependencies);
dependencies.setPomRefId("protoj.project");
return dependencies;
}
/**
* Adds an ant task to the specified target that copies the project pom.xml
* dependencies into the lib directory. Whether or not the javadocs, sources
* or classes dependencies are being configured is determined by the value
* of the dependency parameter.
*
* @param target
* @param dependency
* @return
*/
private Copy addDependency(AntTarget target, String dependency) {
ProjectLayout layout = parent.getLayout();
Copy copy = new Copy();
target.addTask(copy);
copy.setTaskName("maven-feature-copy-artifact");
FileSet fileSet = new FileSet();
copy.addFileset(fileSet);
Reference dependencyRef = new Reference(target.getProject(), dependency);
fileSet.setRefid(dependencyRef);
copy.setTodir(layout.getLibDir());
copy.setOverwrite(true);
Mapper flattenMapper = copy.createMapper();
MapperType flattenType = new MapperType();
flattenType.setValue("flatten");
flattenMapper.setType(flattenType);
return copy;
}
/**
* The parent of this feature.
*
* @return
*/
public StandardProject getParent() {
return parent;
}
/**
* The ivy ant task used to update the repository with the project
* dependencies.
*
* @return
*/
public IvyRetrieve getIvyRetrieve() {
return ivyRetrieve;
}
/**
* The maven ant task used to update the repository with the project
* dependencies.
*
* @return
*/
public DependenciesTask getDependencies() {
return dependencies;
}
/**
* The ant task used to copy the repository classes jar files to the lib
* directory.
*
* @return
*/
public Copy getCopyClasses() {
return copyClasses;
}
/**
* The ant task used to copy the repository javadocs jar files to the lib
* directory.
*
* @return
*/
public Copy getCopyJavadocs() {
return copyJavadocs;
}
/**
* The ant task used to copy the repository sources jar files to the lib
* directory.
*
* @return
*/
public Copy getCopySources() {
return copySources;
}
/**
* The ivy file used to define project dependencies.
*
* @return
*/
public File getIvyFile() {
if (ivyFile == null) {
ResourceFeature feature = parent.getResourceFeature();
File confDir = parent.getLayout().getConfDir();
ivyFile = feature.filterResourceToDir(ivyConfig, confDir);
}
return ivyFile;
}
/**
* The maven pom file used to define project dependencies.
*
* @return
*/
public File getMavenFile() {
if (mavenFile == null) {
ResourceFeature feature = parent.getResourceFeature();
File confDir = parent.getLayout().getConfDir();
mavenFile = feature.filterResourceToDir(mavenConfig, confDir);
}
return mavenFile;
}
/**
* The directory where the ivy and maven files are extracted to.
*
* @return
*/
public File getWorkingDir() {
if (workingDir == null) {
workingDir = new File(parent.getLayout().getTargetDir(), "retrieve");
workingDir.mkdirs();
}
return workingDir;
}
}