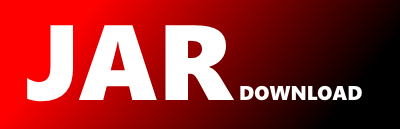
protoj.lang.ScpFeature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import org.apache.tools.ant.taskdefs.optional.ssh.Scp;
import protoj.lang.ClassesArchive.ClassesEntry;
import protoj.lang.internal.InformationException;
import protoj.util.ScpTask;
/**
* This feature is used to remotely copy any classes archive as configured in
* {@link ArchiveFeature#addClasses(String)}. Choose from
* {@link #copyClasses(String, String, String)} or
* {@link #copyClasses(String, String, String, File)} depending whether or not
* key based authentication is required.
*
* @author Ashley Williams
*
*/
public final class ScpFeature {
/**
* The ant scp implementation.
*/
private ScpTask scpTask;
/**
* See {@link #getProject()}.
*/
private final StandardProject parent;
/**
* See {@link #getToDir()}.
*/
private String toDir;
/**
* See {@link #getKeyFile()}.
*/
private File keyFile;
/**
* Creates an instance with the owning parent project.
*
* @param parent
*/
public ScpFeature(StandardProject parent) {
this.parent = parent;
}
/**
* Copies the archive file to the remote location using simple password
* authentication. The toDir
parameter takes the form
* @:
.
*
* @param archiveName
* the name of the classes archive that is to be copied, without
* the extension, that resides in the
* {@link ProjectLayout#getArchiveDir()} directory.
* @param toDir
* eg @:
* @param password
* the scp password, can be an empty string
*/
public void copyClasses(String archiveName, String toDir, String password) {
copyProjectArchive(archiveName, false, toDir, null, password);
}
/**
* Copies the project tar file to the remote location using key based
* authentication. The toDir
parameter takes the form
* @:
.
*
* @param archiveName
* the name of the classes archive that is to be copied, without
* the extension, that resides in the
* {@link ProjectLayout#getArchiveDir()} directory.
* @param toDir
* eg @:
* @param passphrase
* the scp passphrase, can be an empty string
* @param keyFile
* the location of the private key file
*/
public void copyClasses(String archiveName, String toDir,
String passphrase, File keyFile) {
copyProjectArchive(archiveName, true, toDir, keyFile, passphrase);
}
private void copyProjectArchive(String archiveName, boolean isKeyAuth,
String toDir, File keyFile, String passText) {
this.toDir = toDir;
this.keyFile = keyFile;
this.scpTask = createScpTask(archiveName);
if (isKeyAuth) {
this.scpTask.initKeyAuthentication(passText, keyFile
.getAbsolutePath());
} else {
this.scpTask.initPasswordAuthentication(passText);
}
this.scpTask.execute();
}
/**
* Creates the default scp task instance ready for further customization.
*
* @param archiveName
*
* @return
*/
private ScpTask createScpTask(String archiveName) {
ArchiveFeature feature = parent.getArchiveFeature();
ClassesEntry entry = feature.getClassesArchive().getEntry(archiveName);
// check that the archive is managed by the archive feature
if (entry == null) {
throw new InformationException("unrecognized archive name: "
+ archiveName);
}
File archive = entry.getArchiveEntry().getArtifact();
// check that the archive has been created
if (!archive.exists()) {
StringBuilder builder = new StringBuilder();
builder.append("can't find the archive at ");
builder.append(archive.getAbsolutePath());
builder.append("\nplease create it first");
throw new RuntimeException(builder.toString());
}
// should be ok to do the scp
ScpTask scp = new ScpTask();
scp.getTask().setRemoteTodir(toDir);
scp.getTask().setLocalFile(archive.getAbsolutePath());
scp.initLogging();
return scp;
}
/**
* The parent project of this feature.
*
* @return
*/
public StandardProject getProject() {
return parent;
}
/**
* The underlying scp ant object for fine grained customization.
*
* @return
*/
public Scp getAntScp() {
return scpTask.getTask();
}
/**
* The toDir of the remote machine.
*
* @return
*/
public String getToDir() {
return toDir;
}
/**
* The location of the private key when key based authentication is used.
*
* @return
*/
public File getKeyFile() {
return keyFile;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("host: ");
builder.append(scpTask.getTask().getHost());
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy