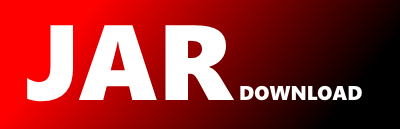
protoj.lang.ScpconfFeature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
package protoj.lang;
import java.io.File;
import org.apache.commons.io.FileUtils;
import protoj.util.ScpTask;
/**
* Equivalent to {@link DirconfFeature} except that the profile directories are
* located using the scp protocol. This means for example that all profiles can
* be stored and modified in a single directory on the network, preferably with
* a backup policy. This provides a quick and easy way of implementing
* persistent configuration.
*
* @author Ashley Williams
*
*/
public final class ScpconfFeature {
/**
* The ant scp implementation.
*/
private ScpTask scpTask;
/**
* Files are copied from the remote location to here.
*/
private File workingDir;
/**
* The implentation object that applies the profile.
*/
private DirconfFeature dirconf;
/**
* See {@link #ScpconfFeature(StandardProject, String)}.
*/
private final String parentUrl;
/**
* See {@link #ScpconfFeature(StandardProject, String)}.
*/
private final StandardProject parent;
/**
* This method must be called if configuration with properties files is to
* be supported from a remote directory. Broadly speaking, setting
* interpolated to true means that property placeholders of the form ${var}
* in config files will get replaced with their real values. This is useful
* if you use libraries that can't handle property placeholders at runtime.
*
* @param parent
* the owning instance
* @param parentUrl
* the url of the remote machine, the parent of the remote
* profile eg "[email protected]:~/allprofiles"
*/
public ScpconfFeature(StandardProject parent, String parentUrl) {
this.parent = parent;
this.parentUrl = parentUrl;
this.workingDir = new File(parent.getLayout().getTargetDir(),
"scpconfig");
this.dirconf = new DirconfFeature(parent, workingDir.getParentFile());
}
/**
* Configures the application with the config files copied from the remote
* profile directory. See {@link #transferProfile(String, File, String)} for
* a parameter description.
*
* @param name
* @param interpolate
* @param keyfile
* @param passtext
*/
public void configure(String name, boolean interpolate, File keyfile,
String passtext) {
transferProfile(name, keyfile, passtext);
dirconf.configure(name, interpolate);
}
/**
* Simple strategy that deletes the files mirroring the profile directory
* structure. See {@link #transferProfile(String, File, String)} for a
* parameter description.
*
* @param name
* @param keyfile
* @param passtext
*/
public void clean(String name, File keyfile, String passtext) {
transferProfile(name, keyfile, passtext);
dirconf.clean(name);
}
/**
* Configures the application with the config files copied from the remote
* profile directory.
*
* @param name
* the profile directory under the parentUrl eg testprofile
* @param interpolate
* true if ${var} placeholders should be replaced with their
* actual values
* @param keyfile
* the private key file, or null if simple password
* authentication is being used
* @param passtext
* the passphrase if key based authentication is being used, or
* password otherwise
*/
private void transferProfile(String name, File keyfile, String passtext) {
String profileUrl = parentUrl + "/" + name;
dirconf = new DirconfFeature(parent, workingDir.getParentFile());
FileUtils.deleteDirectory(workingDir);
workingDir.mkdirs();
scpTask = new ScpTask();
scpTask.initLogging();
scpTask.getTask().setRemoteFile(profileUrl + "/*");
scpTask.getTask().setLocalTodir(workingDir.getAbsolutePath());
if (keyfile != null) {
this.scpTask.initKeyAuthentication(passtext, keyfile
.getAbsolutePath());
} else {
scpTask.initPasswordAuthentication(passtext);
}
scpTask.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy