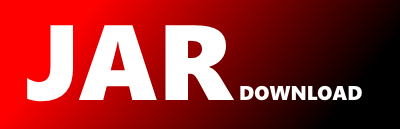
protoj.lang.ScriptSession Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import java.util.ArrayList;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Provides the ability to programmatically issue commands to a project script,
* which is useful in writing tests for example.
*
* Simply create an instance with the location of the project shell script and
* load the session up with commands to be executed by repeated calls to
* {@link #addCommand(String, ArgRunnable, String)}.
*
* The listener arguments also supplied get called back at the end of each
* command execution, providing an opportunity to test proper behavior with
* assertions. Where the same listener is added for different commands, they can
* be distinguished with a provided tag. Therefore the listener body can
* distinguish between the different commands that cause their invocation.
*
* Calling the {@link #execute()} method kicks this off.
*
* @author Ashley Williams
*
*/
public final class ScriptSession {
/**
* See {@link #getShellScript()}.
*/
private File shellScript;
/**
* See {@link #getCurrentExec()}.
*/
private CommandTask currentExec;
/**
* See {@link #getCurrentCommand()}.
*/
private String currentCommand;
/**
* See {@link #getCurrentTag()}.
*/
private String currentTag;
/**
* The names of the commands to be executed.
*/
private ArrayList commands = new ArrayList();
/**
* The tags corresponding to the commands to be executed.
*/
private ArrayList tags = new ArrayList();
/**
* The listeners that get triggered after each command execution.
*/
private ArrayList> listeners = new ArrayList>();
/**
* See {@link ScriptSession}.
*
* @param shellScript
*/
public ScriptSession(File shellScript) {
this.shellScript = shellScript;
}
/**
* Convenience call when not interested in receiving a call to a listener
* when the command has completed.
*
* @param command
*/
public void addCommand(String command) {
addCommand(command, null, null);
}
/**
* Queue the given command ready to be executed.
*
* @param command
* the command to be executed
* @param listener
* the listener to call when the command has completed, can be
* null
* @param tag
* the tag to be associated with the command when it executes,
* can be null
*/
public void addCommand(String command, ArgRunnable listener,
String tag) {
commands.add(command);
listeners.add(listener);
tags.add(tag);
}
/**
* This method executes all of the accumulated commands, calling back the
* listener after each one. All the properties are updated so that the
* listener is able to examine them as desired.
*/
public void execute() {
File scriptDir = shellScript.getParentFile();
String executable = shellScript.getCanonicalPath();
for (int i = 0; i < commands.size(); i++) {
currentTag = tags.get(i);
currentCommand = commands.get(i);
currentExec = new CommandTask(scriptDir, executable,
currentCommand, null);
currentExec.initSpawn(false);
currentExec.execute();
ArgRunnable currentListener = listeners.get(i);
if (currentListener != null) {
currentListener.run(this);
}
}
}
/**
* The script that the commands are sent to.
*
* @return
*/
public File getShellScript() {
return shellScript;
}
/**
* The command object resulting from the most recent command execution,
* containing useful information such as stdout.
*
* @return
*/
public CommandTask getCurrentExec() {
return currentExec;
}
/**
* The name of the most recently executed command.
*
* @return
*/
public String getCurrentCommand() {
return currentCommand;
}
/**
* The tag associated with the most recently executed command. Useful if
* there are added two commands of the same name that share the same
* listener, and so can be used to distinguish between them.
*
* @return
*/
public String getCurrentTag() {
return currentTag;
}
}