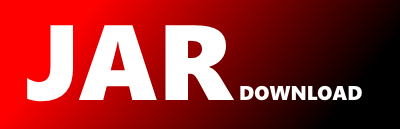
protoj.lang.StandardProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
/**
* These are all the properties that are added to the {@link PropertyStore} on
* behalf of the StandardProject.
*
* @author Ashley Williams
*
*/
public final class StandardProperties {
/**
* See {@link #getLevel()}.
*/
private PropertyInfo level;
/**
* See {@link #getqQuiet()}.
*/
private PropertyInfo quiet;
/**
* See {@link #getJunitMemory()}.
*/
private PropertyInfo junitMemory;
/**
* See {@link #getJunitDebug()}.
*/
private PropertyInfo junitDebug;
/**
* See {@link #getJunitDebugSuspend()}.
*/
private PropertyInfo junitDebugSuspend;
/**
* See {@link #getJunitDebugPort()}.
*/
private PropertyInfo junitDebugPort;
/**
* See {@link #getMainMemory()}.
*/
private PropertyInfo mainMemory;
/**
* See {@link #getMainDebug()}.
*/
private PropertyInfo mainDebug;
/**
* See {@link #getMainDebugSuspend()}.
*/
private PropertyInfo mainDebugSuspend;
/**
* See {@link #getMainDebugPort()}.
*/
private PropertyInfo mainDebugPort;
/**
* See {@link #getGcskip()}.
*/
private PropertyInfo gcskip;
/**
* See {@link #getScpskip()}.
*/
private PropertyInfo scpskip;
/**
* See {@link #getStatusWindow()}.
*/
private PropertyInfo statusWindow;
/**
* See {@link #getCompileSource()}.
*/
private PropertyInfo compileSource;
/**
* See {@link #getCompileTarget()}.
*/
private PropertyInfo compileTarget;
/**
* See {@link #getCompileBootclasspath()}.
*/
private PropertyInfo compileBootclasspath;
/**
* See {@link #getCompileExtdirs()}.
*/
private PropertyInfo compileExtdirs;
/**
* See {@link #getCompileMemory()}.
*/
private PropertyInfo compileMemory;
/**
* See {@link #getProfileHome()}.
*/
private PropertyInfo profileHome;
/**
* See {@link #getProfileUrl()}.
*/
private PropertyInfo profileUrl;
/**
* See {@link #getParent()}.
*/
private final StandardProject parent;
/**
* Create an instance with the owning parent project.
*
* @param parent
*/
public StandardProperties(StandardProject parent) {
this.parent = parent;
this.level = parent
.getPropertyStore()
.addInfo(
"protoj.level",
"INFO",
"INFO",
"use this to configure application logging by setting to one of the log4j logging level constants");
this.junitMemory = parent.getPropertyStore().addInfo(
"protoj.junit.memory", "", "",
"Specify the amount of memory for the junit vm, eg \"4m\"");
this.junitDebug = parent.getPropertyStore().addInfo(
"protoj.junit.debug", Boolean.FALSE.toString(),
Boolean.TRUE.toString(),
"Set to true in order to debug the junit vm");
this.junitDebugSuspend = parent
.getPropertyStore()
.addInfo("protoj.junit.debug.suspend",
Boolean.FALSE.toString(), Boolean.TRUE.toString(),
"Set to true to suspend the junit vm whilst waiting for a debugger connection");
this.junitDebugPort = parent
.getPropertyStore()
.addInfo("protoj.junit.debug.port", "11111", "11111",
"set to a valid port number to be used when debugging the junit vm");
this.mainMemory = parent.getPropertyStore().addInfo(
"protoj.main.memory", "", "",
"Specify the amount of memory for the main vm, eg \"4m\"");
this.mainDebug = parent.getPropertyStore().addInfo("protoj.main.debug",
Boolean.FALSE.toString(), Boolean.TRUE.toString(),
"Set to true in order to debug the main vm");
this.mainDebugSuspend = parent
.getPropertyStore()
.addInfo("protoj.main.debug.suspend", Boolean.FALSE.toString(),
Boolean.TRUE.toString(),
"Set to true to suspend the main vm whilst waiting for a debugger connection");
this.mainDebugPort = parent
.getPropertyStore()
.addInfo("protoj.main.debug.port", "11111", "11111",
"set to a valid port number to be used when debugging the main vm");
this.quiet = parent.getPropertyStore().addInfo("protoj.quiet",
Boolean.FALSE.toString(), Boolean.TRUE.toString(),
"set to true to stop most output to the console");
this.gcskip = parent.getPropertyStore().addInfo("protoj.gcskip",
"true", "true", getGcskipDescription());
this.scpskip = parent.getPropertyStore().addInfo("protoj.scpskip",
"true", "true", getScpskipDescription());
this.statusWindow = parent.getPropertyStore().addInfo(
"protoj.statusWindow", "false", "true",
getStatusWindowDescription());
this.compileSource = parent.getPropertyStore().addInfo(
"protoj.compile.source",
"the compiler source option, does not default to any value");
this.compileTarget = parent.getPropertyStore().addInfo(
"protoj.compile.target",
"the compiler target option, does not default to any value");
this.compileBootclasspath = parent
.getPropertyStore()
.addInfo("protoj.compile.bootclasspath",
"the compiler bootclasspath option, does not default to any value");
this.compileExtdirs = parent.getPropertyStore().addInfo(
"protoj.compile.extdirs",
"the compiler extdirs option, does not default to any value");
this.compileMemory = parent
.getPropertyStore()
.addInfo("protoj.compile.memory",
"the compiler maximum memory option, does not default to any value");
this.profileHome = parent
.getPropertyStore()
.addInfo("protoj.profile.home",
"the directory that contains the profiles available for configuration");
this.profileUrl = parent
.getPropertyStore()
.addInfo("protoj.profile.url",
"the remote url that contains the profiles available for configuration");
}
/**
* The owning parent of this class.
*
* @return
*/
public StandardProject getParent() {
return parent;
}
private String getStatusWindowDescription() {
StringBuilder builder = new StringBuilder();
builder
.append("Specify whether or not a window gets displayed indicating project");
builder
.append("\nsuccess (green) or failure (red). The application does not terminate");
builder.append("\nunless the window is closed.");
builder.append("\nThe two possible values are:");
builder
.append("\n\n 1. true: the windows gets diplayed, provided there is a gui system available.");
builder.append("\n\n 2. false: no window gets displayed at all.");
return builder.toString();
}
private String getScpskipDescription() {
StringBuilder builder = new StringBuilder();
builder
.append("Specify whether or not the project tar file gets remotely copied during scp.");
builder.append("\nThe two possible values are:");
builder
.append("\n\n 1. true: the tar file doesn't get copied and an informative message is logged.");
builder.append("\n\n 2. false: the tar file gets copied normally.");
return builder.toString();
}
/**
* Factoring out large description into its own method.
*
* @return
*/
private String getGcskipDescription() {
StringBuilder builder = new StringBuilder();
builder
.append("Specify whether or not the project tar file gets uploaded to google code.");
builder.append("\nThe two possible values are:");
builder
.append("\n\n 1. true: the tar file doesn't get posted and an informative message is logged.");
builder.append("\n\n 2. false: the tar file gets posted normally.");
return builder.toString();
}
/**
* One of the logging levels constant values as declared in the log4j Level
* class.
*
* @return
*/
public PropertyInfo getLevel() {
return level;
}
/**
* Overrides the amount of memory used by the main vm.
*
* @return
*/
public PropertyInfo getMainMemory() {
return mainMemory;
}
/**
* Overrides the amount of memory used by the specified command vm.
*
* @param name
* @return
*/
public PropertyInfo getCommandMemory(String name) {
String key = "protoj.memory." + name;
return parent.getPropertyStore().getLazyInfo(key,
"Specify the amount of memory for the command vm, eg \"4m\"",
"", "");
}
/**
* Specifies whether or not to debug for a given command.
*
* @param name
* @return
*/
public PropertyInfo getCommandDebug(String name) {
String key = "protoj.debug." + name;
StringBuilder builder = new StringBuilder();
builder
.append("Set to true in order to debug a vm for the specified command vm");
builder.append("\nFor example -D" + key);
return parent.getPropertyStore().getLazyInfo(key, builder.toString(),
Boolean.FALSE.toString(), Boolean.TRUE.toString());
}
/**
* Specifies whether or not to suspend a vm for the given command name
* during debugging.
*
* @param name
* @return
*/
public PropertyInfo getCommandDebugSuspend(String name) {
String key = "protoj.debug.suspend." + name;
StringBuilder builder = new StringBuilder();
builder
.append("Set to true in order to suspend a vm when starting a debug session for");
builder.append(" the specified command. " + "\nFor example -D" + key);
return parent.getPropertyStore().getLazyInfo(key, builder.toString(),
Boolean.FALSE.toString(), Boolean.TRUE.toString());
}
/**
* Specifies the remote debugging port to use when debugging for the given
* command.
*
* @param name
* @return
*/
public PropertyInfo getCommandDebugPort(String name) {
String key = "protoj.debug.port." + name;
StringBuilder builder = new StringBuilder();
builder
.append("Set to the port number to use when starting a debug session for");
builder.append(" the specified command. " + "\nFor example -D" + key
+ "=11111");
return parent.getPropertyStore().getLazyInfo(key, builder.toString(),
"11111", "11111");
}
/**
* Set to true or false to turn the console appender on or off.
*
* @return
*/
public PropertyInfo getQuiet() {
return quiet;
}
/**
* Overrides the amount of memory used by the junit vm.
*
* @return
*/
public PropertyInfo getJunitMemory() {
return junitMemory;
}
/**
* Set to true or false to to turn debugging on or off for the junit vm.
*
* @return
*/
public PropertyInfo getJunitDebug() {
return junitDebug;
}
/**
* Set to true or false to switch suspend on or off for the junit vm
* debugging session.
*
* @return
*/
public PropertyInfo getJunitDebugSuspend() {
return junitDebugSuspend;
}
/**
* Set to a valid port number that the junit vm debugger should listen for
* connections on.
*
* @return
*/
public PropertyInfo getJunitDebugPort() {
return junitDebugPort;
}
/**
* Set to true or false to to turn debugging on or off for the main vm.
*
* @return
*/
public PropertyInfo getMainDebugProperty() {
return mainDebug;
}
/**
* Set to true or false to switch suspend on or off for the main vm
* debugging session.
*
* @return
*/
public PropertyInfo getMainDebugSuspend() {
return mainDebugSuspend;
}
/**
* Set to a valid port number that the main vm debugger should listen for
* connections on.
*
* @return
*/
public PropertyInfo getMainDebugPort() {
return mainDebugPort;
}
/**
* Whether or not the upload to google code should occur.
*
* @return
*/
public PropertyInfo getGcskip() {
return gcskip;
}
/**
* Whether or not the remote copy via scp should occur.
*
* @return
*/
public PropertyInfo getScpskip() {
return scpskip;
}
/**
* Whether or not to display the colored window at the end of the build.
*
* @return
*/
public PropertyInfo getStatusWindow() {
return statusWindow;
}
/**
* The javac source option.
*
* @return
*/
public PropertyInfo getCompileSource() {
return compileSource;
}
/**
* The javac target option for cross-compilation.
*
* @return
*/
public PropertyInfo getCompileTarget() {
return compileTarget;
}
/**
* The javac bootstrap option for cross-compilation.
*
* @return
*/
public PropertyInfo getCompileBootclasspath() {
return compileBootclasspath;
}
/**
* The javac extdirs option for cross-compilation.
*
* @return
*/
public PropertyInfo getCompileExtdirs() {
return compileExtdirs;
}
/**
* The javac maximum memory option.
*
* @return
*/
public PropertyInfo getCompileMemory() {
return compileMemory;
}
/**
* The directory containing all the profiles that can be used for
* configuring application instances. Used in conjunction with
* {@link DirconfFeature}.
*
* @return
*/
public PropertyInfo getProfileHome() {
return profileHome;
}
/**
* The remote url containing all the profiles that can be used for
* configuring application instances. Used in conjuntion with
* {@link ScpconfFeature}.
*
* @return
*/
public PropertyInfo getProfileUrl() {
return profileUrl;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy