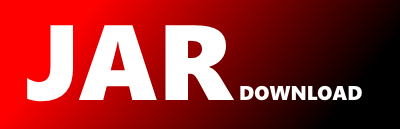
protoj.lang.UploadGoogleCodeFeature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang;
import java.io.File;
import net.bluecow.googlecode.ant.GoogleCodeUploadTask;
import org.apache.tools.ant.Project;
import protoj.lang.internal.InformationException;
import protoj.util.AntTarget;
/**
* This feature is used to upload project artifacts to the google code project
* website. It is an error to attempt the upload a non existent artifact
* resulting in application termination.
*
* The most generic way of uploading an artifact to google code is to use the
* {@link #uploadArtifact(File, String, String, String, String, String)} method
* which accepts any file. The following snippet shows how to upload a javadoc
* foo jar file:
*
*
* StandardProject project;
* JavadocArchive archive = project.getArchiveFeature().getJavadocArchive();
* UploadGoogleCodeFeature upload = project.getUploadGoogleCodeFeature();
*
* ArchiveEntry<JavadocArchive> entry = archive.getEntry("foo").getArchiveEntry();
* File artifact = entry.getArtifact();
* upload.uploadArtifact(artifact, "googleproj", "Featured", "release candidate",
* "user", "pass");
*
*
* @author Ashley Williams
*
*/
public final class UploadGoogleCodeFeature {
private GoogleCodeUploadTask upload;
/**
* See {@link #getProject()}.
*/
private final StandardProject project;
/**
* Configures this feature for uploading either the project tar or the
* project jar depending on the value of the uploadTar
* argument. See the google code website for further explanation of the
* remaining parameters.
*
* @param project
* the owning project of this feature
* @param googleProjectName
* the name of the google project
*/
public UploadGoogleCodeFeature(StandardProject project,
String googleProjectName) {
this.project = project;
AntTarget target = new AntTarget("upload");
upload = new GoogleCodeUploadTask();
upload.setTaskName("do-upload");
target.initLogging(Project.MSG_INFO);
target.addTask(upload);
upload.setProjectName(googleProjectName);
}
/**
* Convenience method that delegates to
* {@link #uploadArtifact(File, String, String, String, String, String)}
* with the project tar file created via the {@link ProjectArchive} class.
*
* @param googleName
* @param labels
* @param summary
* @param userName
* @param password
*/
public void uploadTar(String googleName, String labels, String summary,
String userName, String password) {
String tarPath = project.getArchiveFeature().getProjectArchive()
.getArchivePath();
File tarFile = new File(tarPath);
uploadArtifact(tarFile, googleName, labels, summary, userName, password);
}
/**
* Convenience method that delegates to
* {@link #uploadArtifact(File, String, String, String, String, String)}
* with the artifact relative to the project root. Useful for uploading jar
* files for example.
*
* @param relativeName
* @param googleName
* @param labels
* @param summary
* @param userName
* @param password
*/
public void uploadRelativeArtifact(String relativeName, String googleName,
String labels, String summary, String userName, String password) {
File artifact = new File(project.getLayout().getRootDir(), relativeName);
uploadArtifact(artifact, googleName, labels, summary, userName,
password);
}
/**
* Uploads the given artifact to the google code project website using the
* specified information. The googleName parameter is the name of the file
* as it will appear on the google code website.
*
* @param googleName
* @param labels
* @param summary
* @param userName
* @param password
*/
public void uploadArtifact(File artifact, String googleName, String labels,
String summary, String userName, String password) {
if (!artifact.exists()) {
throw new InformationException(
("no artifact: can't find file to upload to google " + artifact
.getAbsolutePath()));
}
upload.setUserName(userName);
upload.setPassword(password);
upload.setFileName(artifact.getAbsolutePath());
upload.setTargetFileName(googleName);
upload.setLabels(labels);
upload.setSummary(summary);
upload.execute();
}
/**
* The parent project of this feature.
*
* @return
*/
public StandardProject getProject() {
return project;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("userName: ");
builder.append(upload.getUserName());
builder.append("\nprojectName: ");
builder.append(upload.getProjectName());
builder.append("\nfileName: ");
builder.append(upload.getFileName());
builder.append("\ntargetFileName: ");
builder.append(upload.getTargetFileName());
builder.append("\nsummary: ");
builder.append(upload.getSummary());
builder.append("\nlabels: ");
builder.append(upload.getLabels());
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy