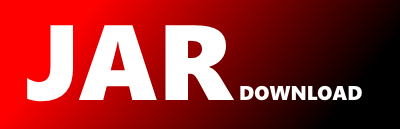
protoj.lang.command.ScpCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.command;
import java.io.File;
import joptsimple.OptionParser;
import joptsimple.OptionSet;
import joptsimple.OptionSpec;
import protoj.lang.Command;
import protoj.lang.CommandStore;
import protoj.lang.ScpFeature;
import protoj.lang.StandardProject;
/**
* Command driven access to {@link ScpFeature}.
*
* @author Ashley Williams
*
*/
public final class ScpCommand {
private final class Body implements Runnable {
public void run() {
Command delegate = getDelegate();
OptionSet options = delegate.getOptions();
// archive option is mandatory
if (!options.has(archiveOption)) {
delegate
.throwBadOptionsException("please specify the name of an archive");
}
// dir option is mandatory
if (!options.has(dirOption)) {
delegate
.throwBadOptionsException("please specify a dir option");
}
String archive = options.valueOf(archiveOption);
String dir = options.valueOf(dirOption);
if (options.has(keyFileOption)) {
// key based - empty phrase assumed when option missing
String phrase;
if (options.has(phraseOption)) {
phrase = options.valueOf(phraseOption);
} else {
phrase = "";
}
File keyFile = options.valueOf(keyFileOption);
project.getScpFeature().copyClasses(archive, dir, phrase,
keyFile);
} else {
// password based - empty password assumed when option missing
String password;
if (options.has(passwordOption)) {
password = options.valueOf(passwordOption);
} else {
password = "";
}
project.getScpFeature().copyClasses(archive, dir, password);
}
}
}
private final StandardProject project;
private Command delegate;
private OptionSpec keyFileOption;
private OptionSpec dirOption;
private OptionSpec passwordOption;
private OptionSpec phraseOption;
private OptionSpec archiveOption;
public ScpCommand(StandardProject project) {
this.project = project;
CommandStore store = project.getCommandStore();
delegate = store.addCommand("scp", "16m", new Body());
delegate.initHelpResource("/protoj-common/language/english/scp.txt");
OptionParser parser = delegate.getParser();
archiveOption = parser.accepts(getArchiveOption()).withRequiredArg();
dirOption = parser.accepts(getDirOption()).withRequiredArg();
passwordOption = parser.accepts(getPasswordOption()).withOptionalArg();
phraseOption = parser.accepts(getPhraseOption()).withOptionalArg();
keyFileOption = parser.accepts(getKeyfileOption()).withRequiredArg()
.ofType(File.class);
}
public String getKeyfileOption() {
return "keyfile";
}
public String getPhraseOption() {
return "phrase";
}
public String getPasswordOption() {
return "password";
}
public String getDirOption() {
return "dir";
}
public String getArchiveOption() {
return "archive";
}
public Command getDelegate() {
return delegate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy