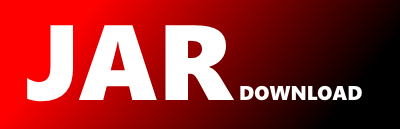
protoj.lang.command.StandardCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.command;
import protoj.lang.CommandStore;
import protoj.lang.StandardProject;
/**
* These are all the commands that are added to the {@link CommandStore} on
* behalf of the {@link StandardProject}.
*
* @author Ashley Williams
*
*/
public final class StandardCommands {
/**
* See {@link #getCompile()}.
*/
private CompileCommand compile;
/**
* See {@link #getJavac()}.
*/
private JavacCommand javac;
/**
* See {@link #getAjc()}.
*/
private AjcCommand ajc;
/**
* See {@link #getRetrieve()}.
*/
private RetrieveCommand retrieve;
/**
* See {@link #getClean()}.
*/
private CleanCommand clean;
/**
* See {@link #getPublish()}.
*/
private PublishCommand publish;
/**
* See {@link #getHelp()}.
*/
private HelpCommand help;
/**
* See {@link #getVersion()}.
*/
private VersionCommand version;
/**
* See {@link #getJunit()}.
*/
private JunitCommand junit;
/**
* See {@link #getArchive()}.
*/
private ArchiveCommand archive;
/**
* See {@link #getTar()}.
*/
private TarCommand tar;
/**
* See {@link #getVerifyTar()}.
*/
private VerifyTarCommand verifyTar;
/**
* See {@link #getScp()}.
*/
private ScpCommand scp;
/**
* See {@link #getDirconf()}.
*/
private DirconfCommand dirconf;
/**
* See {@link #getScpconf()}.
*/
private ScpconfCommand scpconf;
/**
* Create an instance with the owning parent project.
*
* @param parent
*/
public StandardCommands(StandardProject parent) {
this.compile = new CompileCommand(parent);
this.javac = new JavacCommand(parent);
this.ajc = new AjcCommand(parent);
this.retrieve = new RetrieveCommand(parent);
this.help = new HelpCommand(parent);
this.clean = new CleanCommand(parent);
this.publish = new PublishCommand(parent);
this.version = new VersionCommand(parent);
this.junit = new JunitCommand(parent);
this.archive = new ArchiveCommand(parent);
this.tar = new TarCommand(parent);
this.verifyTar = new VerifyTarCommand(parent);
this.scp = new ScpCommand(parent);
this.dirconf = new DirconfCommand(parent);
this.scpconf = new ScpconfCommand(parent);
}
/**
* Use this command to copy project artifacts with the scp protocol.
*
* @return
*/
public ScpCommand getScp() {
return scp;
}
/**
* Use this command to apply profiles to the project from a mounted
* directory.
*
* @return
*/
public DirconfCommand getDirconf() {
return dirconf;
}
/**
* Use this command to apply profiles to the project from a remote url.
*
* @return
*/
public ScpconfCommand getScpconf() {
return scpconf;
}
/**
* Use this command to report parent information such as version strings and
* help text to stdout.
*
* @return
*/
public HelpCommand getHelp() {
return help;
}
/**
* Use this command to report version information to stdout.
*
* @return
*/
public VersionCommand getVersion() {
return version;
}
/**
* Use this command to clean the generated content, not including the log
* file.
*
* @return
*/
public CleanCommand getClean() {
return clean;
}
/**
* Use this command to create one or more jar files from the parent
* resources and compiled classes.
*
* @return
*/
public ArchiveCommand getArchive() {
return archive;
}
/**
* Use this command to exercise all junit tests under the source directory.
*
* @return
*/
public JunitCommand getJunit() {
return junit;
}
/**
* Use this command to make a compressed tar of the parent.
*
* @return
*/
public TarCommand getTar() {
return tar;
}
/**
* Use this command to verify the correctness of a created parent tar.
*
* @return
*/
public VerifyTarCommand getVerifyTar() {
return verifyTar;
}
/**
* Use this command to access the functionality for downloading
* dependencies.
*
* @return
*/
public RetrieveCommand getRetrieve() {
return retrieve;
}
/**
* Use this command to perform in-code compilation.
*
* @return
*/
public CompileCommand getCompile() {
return compile;
}
/**
* Use this command to invoke javac usually from a script to compile just
* the bootstrap portion of a project.
*
* @return
*/
public JavacCommand getJavac() {
return javac;
}
/**
* Use this command to invoke ajc usually from a script to compile just the
* bootstrap portion of a project.
*
* @return
*/
public AjcCommand getAjc() {
return ajc;
}
/**
* Use this command to publish parent artifacts.
*
* @return
*/
public PublishCommand getPublish() {
return publish;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy