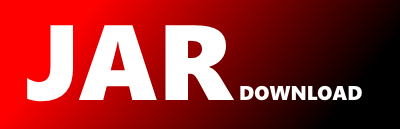
protoj.lang.internal.ProjectReporter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal;
import org.apache.log4j.Logger;
import protoj.lang.DispatchFeature;
import protoj.lang.StandardProject;
/**
* Responds to project events by logging appropriate messages.
*
* @author Ashley Williams
*
*/
public final class ProjectReporter {
private final StandardProject parent;
/**
* Construct with the parent aggregate of this instance.
*
* @param parent
*/
public ProjectReporter(StandardProject parent) {
this.parent = parent;
}
/**
* If we are in the shell script vm then we should report as normal. If not
* then we have been bootstrapped to an implementation vm and so to prevent
* doubled up messages, reporting needs to be disabled.
*
* @return
*/
public boolean isReportingEnabled() {
return true;
}
/**
* Logs that a command is about to be executed.
*
* @param command
*/
public void beginCommand(String command) {
if (isReportingEnabled()) {
getLogger().info("");
getLogger().info(getHighlightedMessage(command));
getLogger().info("");
}
}
/**
* Logs that the given command was unknown when an execution was attempted.
*
* @param command
*/
public void unknownCommand(String command) {
if (isReportingEnabled()) {
getLogger().error("unknown command: \"" + command + "\"");
}
}
/**
* Logs that the project command was successfully executed.
*/
public void projectSucceeded() {
if (isReportingEnabled()) {
getLogger().info("");
getLogger().info("BUILD SUCCESSFUL");
logElapsedTime();
getLogger().info("");
}
}
/**
* Logs that the project command was unsuccessfully executed, using the
* given exception argument.
*
* @param e
*/
public void projectFailed(Throwable e) {
getLogger().info("");
getLogger().info("BUILD FAILED", e);
logElapsedTime();
getLogger().info("");
}
/**
* Logs that the project command was unsuccessfully executed, using the
* given description argument. Additionally reports to stderr.
*
* @param description
*/
public void projectFailed(String description) {
getLogger().info("");
getLogger().info("BUILD FAILED");
getLogger().info(description);
logElapsedTime();
getLogger().info("");
String logPath = parent.getLayout().getLogFile().getAbsolutePath();
System.err.println("BUILD FAILED - see " + logPath
+ " for more information");
}
/**
* Appends a message describing the amount of time the build took.
*/
private void logElapsedTime() {
DispatchFeature feature = parent.getDispatchFeature();
StringBuilder message = new StringBuilder();
message.append("Total time: ");
long hours = feature.getElapsedHours();
if (hours == 0) {
// don't show hours
} else if (hours == 1) {
message.append("1 hour ");
} else {
message.append(hours);
message.append(" hours ");
}
long minutes = feature.getElapsedMinutes();
if (minutes == 0) {
// don't show minutes
} else if (minutes == 1) {
message.append("1 minute ");
} else {
message.append(minutes);
message.append(" minutes ");
}
long seconds = feature.getElapsedSeconds();
if (seconds == 0) {
message.append("0 seconds");
} else if (seconds == 1) {
message.append("1 second");
} else {
message.append(seconds);
message.append(" seconds");
}
getLogger().info(message);
}
/**
* Used to format each message in a standard way.
*
* @param message
* @return
*/
public String getHighlightedMessage(String message) {
return message + ":";
}
public Logger getLogger() {
return parent.getLogger();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy