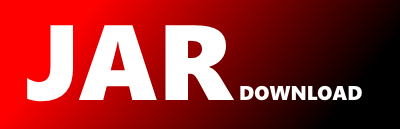
protoj.lang.internal.ReleaseFeature Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal;
import java.io.File;
import protoj.lang.ArchiveEntry;
import protoj.lang.ArchiveFeature;
import protoj.lang.ClassesArchive;
import protoj.lang.PropertyInfo;
import protoj.lang.PublishFeature;
import protoj.lang.StandardProject;
/**
* Responsible for kicking off a release of the ProtoJ project. The release
* culminates in a deployment of the java artifacts to the maven repository at
* source forge and to googlecode.
*
* The prerequisites for invoking the {@link #release(String, String, String)}
* method is that the protoj code is compiled and the wiki directory is checked
* out from source control as a sibling of the protoj project. Also the docs/api
* directory must be deleted from source control as the javadocs will be
* recreated.
*
* @author Ashley Williams
*
*/
public final class ReleaseFeature {
private final ProtoProject project;
public ReleaseFeature(ProtoProject project) {
this.project = project;
}
/**
* Carries out the steps to release protoj starting from just the compiled
* code.
*
* @param gcUserName
* the username for uploading to the googlecode project
* @param gcPassword
* the password for uploading to the googlecode project
* @param sfUserName
* the username for uploading to the sourceforge maven repository
*
*/
public void release(String gcUserName, String gcPassword, String sfUserName) {
showSummary();
createDocs();
// project.getDelegate().getJunitFeature().junit();
project.createAllArchives();
uploadToMaven(sfUserName);
uploadToGoogleCode(gcUserName, gcPassword);
}
/**
* Creates the javadocs and site docs but only in the java 5 build.
*/
private void createDocs() {
boolean isJava5 = !project.getDelegate().isJdk6();
if (isJava5) {
project.extractJavadocs();
project.extractSite();
}
}
/**
* Publishes the archives to the sourceforge maven repository. This is just
* the minimal jar.
*
* @param sfUserName
*/
public void uploadToMaven(String sfUserName) {
PublishFeature publishFeature = project.getDelegate()
.getPublishFeature();
publishFeature.deployAll(sfUserName, null, null, null);
}
/**
* Uploads the executable jar to the google code website.
*
* @param gcUserName
* @param gcPassword
*/
public void uploadToGoogleCode(String gcUserName, String gcPassword) {
StandardProject delegate = project.getDelegate();
ArchiveFeature archive = delegate.getArchiveFeature();
ClassesArchive classes = archive.getClassesArchive();
ArchiveEntry entry = classes.getEntry(
project.getVersionedExeJarTag()).getArchiveEntry();
String googleName = String.format("%s-%s.jar", entry.getName(), project
.getVersion());
File artifact = entry.getArtifact();
delegate.getUploadGoogleCodeFeature().uploadArtifact(artifact,
googleName, "Type-Archive, OpSys-All, Featured",
"executable jar file, execute with --help", gcUserName,
gcPassword);
}
/**
* The release takes a long time so it's worth knowing whether or not an
* upload to google code will take place at the end of it.
*/
private void showSummary() {
StandardProject delegate = project.getDelegate();
PropertyInfo gcskip = delegate.getProperties().getGcskip();
StringBuilder builder = new StringBuilder();
if (gcskip.getBooleanValue() == true) {
builder
.append("ProtoJ won't be uploaded to google code during this release.");
} else {
builder
.append("ProtoJ will be uploaded to google code during this release.");
}
builder.append(" See the help for ");
builder.append(gcskip.getKey());
builder.append(" for more information.");
delegate.getLogger().info(builder.toString());
}
}