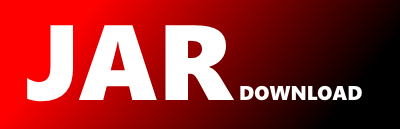
protoj.lang.internal.SampleProjectFeature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
package protoj.lang.internal;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.util.Collection;
import org.apache.commons.io.IOUtils;
import protoj.lang.DependencyInfo;
import protoj.lang.DependencyStore;
import protoj.lang.ProjectLayout;
import protoj.lang.StandardProject;
/**
* Responsible for creating a sample project on the filing system.
*
* @author Ashley Williams
*
*/
public final class SampleProjectFeature {
/**
* A reference to the sample project layout.
*/
private ProjectLayout layout;
/**
* The owning parent.
*/
private final ProtoProject parent;
/**
* Create an instance with the owning parent.
*
* @param parent
*/
public SampleProjectFeature(ProtoProject parent) {
this.parent = parent;
}
/**
* Creates the basic project under the specified parentDir. This is a
* pass-thru method to {@link #createProject(File, String, String...)} .
*
* @param parentDir
*/
public void createBasicProject(File parentDir) {
createProject(parentDir, "basic", "bin/basic.sh", "bin/basic.bat",
"src/resources/basic/ivy.xml",
"conf/profile/conf/basic.properties", "conf/dev.properties",
"README.txt", "NOTICE.txt",
"src/java/org/basic/core/BasicCore.java",
"src/java/org/basic/system/BasicProject.java");
}
/**
* Creates the ajc project with the specified rootDir. This is a pass-thru
* method to {@link #createProject(File, String, String...)}.
*
* @param parentDir
*/
public void createAjcProject(File parentDir) {
createProject(parentDir, "ajc", "bin/ajc.sh", "bin/ajc.bat",
"src/resources/ajc/ivy.xml", "src/resources/META-INF/aop.xml",
"conf/profile/conf/ajc.properties", "conf/dev.properties",
"README.txt", "NOTICE.txt",
"src/java/org/ajc/core/AjcCore.java",
"src/java/org/ajc/system/AjcProject.java",
"src/java/org/ajc/system/AjcTrace.aj",
"src/java/org/ajc/system/SoftException.aj");
}
/**
* Creates the acme project with the specified rootDir. This is a pass-thru
* method to {@link #createProject(File, String, String...)}.
*
* @param parentDir
*/
public void createAcmeProject(File parentDir) {
createProject(parentDir, "acme", "all-private/red.txt", "bin/acme.sh",
"bin/acme.bat", "conf/profile/conf/acme.properties",
"conf/profile/conf/configa.properties",
"conf/profile/conf/configb.properties",
"conf/profile/docs/sample.properties",
"conf/profile/docs/sample.txt", "conf/all.project.properties",
"log/acme.log", "part-private/amber.txt",
"part-private/green.txt", "src/java/acme/core/AcmeCore.java",
"src/java/acme/util/AcmeUtil.java",
"src/java/acme/AcmeProject.java",
"src/java/acme/AssemblyTrace.aj",
"src/java/acme/FakeSignature.aj",
"src/java/acme/SampleTest.java",
"src/java/acme/SoftException.aj",
"src/manifest/foo-manifest.MF",
"src/resources/acme/acme-publish.xml",
"src/resources/acme/foo-publish.xml",
"src/resources/acme/ivy.xml", "src/resources/META-INF/aop.xml",
"src/resources/test.txt", "README.txt", "NOTICE.txt");
}
/**
* Creates the hello world project with the specified rootDir. This is a
* pass-thru method to {@link #createProject(File, String, String...)} .
*
* @param parentDir
*/
public void createHelloWorldProject(File parentDir) {
createProject(parentDir, "helloworld", "bin/helloworld.sh",
"bin/helloworld.bat", "conf/helloworld.properties",
"conf/profile/conf/helloworld.properties",
"src/java/helloworld/HelloWorld.java", "README.txt",
"NOTICE.txt");
}
/**
* Creates the alien project with the specified rootDir. This is a pass-thru
* method to {@link #createProject(File, String, String...)} .
*
* @param parentDir
*/
public void createAlienProject(File parentDir) {
createProject(parentDir, "alien", "bin/alien.sh", "bin/alien.bat",
"conf/alien.test.properties",
"conf/profile/conf/alien.properties",
"conf/profile/conf/alien.test.properties",
"conf/profile/docs/topsecret.txt",
"conf/profile/conf/alien.properties",
"src/java/alien/core/AlienCore.java",
"src/java/alien/test/AlienTest.java",
"src/java/alien/AlienProject.java",
"src/resources/alien/ivy.xml", "README.txt", "NOTICE.txt");
}
/**
* Creates the serverdemo project with the specified rootDir. This is a
* pass-thru method to {@link #createProject(File, String, String...)} .
*
* @param parentDir
*/
public void createServerDemoProject(File parentDir) {
createProject(
parentDir,
"serverdemo",
"bin/serverdemo.sh",
"bin/serverdemo.bat",
"conf/profile/conf/jboss.opts.properties",
"conf/profile/conf/jboss.bindings.properties",
"conf/profile/conf/serverdemo.properties",
"conf/profile/jboss-5.0.1.GA/server/default/conf/bootstrap/bindings.xml",
"src/java/org/serverdemo/core/ServerDemoCore.java",
"src/java/org/serverdemo/system/ServerDemoProject.java",
"src/java/org/serverdemo/system/JbossWatcher.aj",
"src/java/org/serverdemo/system/SoftException.aj",
"src/resources/META-INF/aop.xml",
"src/resources/org/serverdemo/ivy.xml",
"README.txt",
"src/resources/org/serverdemo/jboss/server/default/conf/jboss-service.xml",
"src/resources/org/serverdemo/jboss/server/default/deploy/properties-service.xml",
"NOTICE.txt");
}
/**
* Creates a project under the specified parent with the specified
* projectName and resource file. The specified jarFile will be used to copy
* into the lib directory and will typically be either the minimal protoj
* jar file or the executable jar file.
*
* @param parentDir
* the directory where the project is to be extracted to
* @param projectName
* the name of the project under the root directory and the name
* of the resources parent containing the files to be extracted
* @param relResourceNames
* the list of resources to be copied
*/
private void createProject(File parentDir, String projectName,
String... relResourceNames) {
File rootDir = new File(parentDir, projectName);
StandardProject project = new StandardProject(rootDir, projectName,
null);
this.layout = project.getLayout();
// creates the directory structure layout
layout.createPhysicalLayout();
// copy the resources common to all sample projects
copyResource("common", "conf/profile/conf/app.properties");
DependencyStore store = parent.getDelegate().getDependencyStore();
Collection infos = store.getDependencies();
for (DependencyInfo info : infos) {
String jarName = info.getJarName();
copyResource("common", "lib/" + jarName);
}
// extracts all the resources to their equivalent locations on the
// filing system
for (String relResourceName : relResourceNames) {
copyResource(layout.getRootName(), relResourceName);
}
// ensure the project can be executed
layout.relaxPermissions();
System.out.println("created project at "
+ layout.getRootDir().getCanonicalPath());
System.out.println("please check the README.txt file");
}
/**
* Copies the file under the /protoj resource path to the project root
* directory on the filing system at the matching relative location.
*
* @param resParent
* the resource path parent of the resource to be copied
* @param name
* the name of the resource relative to the resource dir
*/
private void copyResource(String resParent, String resName) {
String resourceName = "/protoj-exe/" + resParent + "/" + resName;
InputStream in = getClass().getResourceAsStream(resourceName);
if (in == null) {
throw new RuntimeException("couldn't find resource " + resourceName);
}
File dest = new File(layout.getRootDir(), resName);
dest.getParentFile().mkdirs();
dest.createNewFile();
try {
FileOutputStream out = new FileOutputStream(dest);
try {
IOUtils.copy(in, out);
} finally {
IOUtils.closeQuietly(out);
}
} finally {
IOUtils.closeQuietly(in);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy