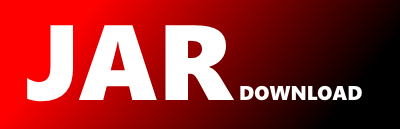
protoj.lang.internal.UserOverride.aj Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal;
import java.io.File;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
import protoj.lang.Command;
import protoj.lang.CommandStore;
import protoj.lang.CompileFeature;
import protoj.lang.DirconfFeature;
import protoj.lang.DispatchFeature;
import protoj.lang.JunitFeature;
import protoj.lang.PropertyInfo;
import protoj.lang.ProtoLogger;
import protoj.lang.ScpFeature;
import protoj.lang.ScpconfFeature;
import protoj.lang.StandardProject;
import protoj.lang.StandardProperties;
import protoj.lang.UploadGoogleCodeFeature;
import protoj.util.ArgRunnable;
import protoj.util.JavaTask;
import protoj.util.JunitTask;
import protoj.util.ScpTask;
/**
* All overrides from system properties are implemented here.
*
* @author Ashley Williams
*
*/
public final aspect UserOverride {
/**
* Checks for attempts to call the google upload functionality from a
* {@link UploadGoogleCodeFeature} instance. If the
* {@link StandardProperties#getGcskip()} system property is not present
* then the upload proceeds as normal.
*/
void around(UploadGoogleCodeFeature feature) : call(* net.bluecow.googlecode.ant.GoogleCodeUploadTask.execute())
&& cflow(this(feature)) {
StandardProject project = feature.getProject();
StandardProperties properties = project.getProperties();
PropertyInfo gcskipProperty = properties.getGcskip();
Boolean skipUpload = gcskipProperty.getBooleanValue();
if (skipUpload) {
StringBuilder builder = new StringBuilder();
builder.append(gcskipProperty.getKey());
builder.append(" property is set to true, so skipping the google");
builder.append(" code upload with the following properties:\n");
builder.append(feature.toString());
project.getLogger().info(builder.toString());
} else {
proceed(feature);
}
}
/**
* Checks for attempts to call the scp functionality from a
* {@link ScpFeature} instance. If the
* {@link StandardProperties#getScpskip()} system property is not present
* then the remote copy proceeds as normal.
*/
void around(ScpFeature feature) : call(* ScpTask.execute())
&& cflow(this(feature)) {
StandardProject project = feature.getProject();
PropertyInfo scpskipProperty = project.getProperties().getScpskip();
Boolean skipScp = scpskipProperty.getBooleanValue();
if (skipScp) {
StringBuilder builder = new StringBuilder();
builder.append(scpskipProperty.getKey());
builder.append(" property is set to true, so skipping the remote");
builder.append(" copy with the following properties:\n");
builder.append(feature.toString());
project.getLogger().info(builder.toString());
} else {
proceed(feature);
}
}
/**
* Shows or hides the status window when the dispatch feature has finished
* dispatching all the commands.
*
* @param project
*/
after(StandardProject project) returning : execution(StandardProject.new(..)) && this(project) {
PropertyInfo statusWindow = project.getProperties().getStatusWindow();
project.getDispatchFeature().setStatusWindow(
statusWindow.getBooleanValue());
}
/**
* Every time the junit feature starts the junit tests, we intervene in
* order to specify debug options.
*
* @param command
* @param project
*/
before(JunitFeature feature, JunitTask task) : call(* execute(..)) && this(feature) && target(task) {
StandardProject project = feature.getParent();
StandardProperties properties = project.getProperties();
// only check other properties if debugging is switched on
if (properties.getJunitDebug().getBooleanValue()) {
int port = properties.getJunitDebugPort().getIntegerValue();
boolean suspend = properties.getJunitDebugSuspend()
.getBooleanValue();
task.initDebug(port, suspend);
String message = "debug mode configured at port " + port
+ " for the junit vm";
project.getLogger().info(message);
}
}
/**
* Every time the dispatch feature bootstraps to the main vm, we intervene
* in order to specify debug options.
*
* @param feature
* the instance used to start the new vm
*/
before(final DispatchFeature feature) : execution(* startProjectMainVm(..)) && this(feature) {
feature.setStartVmConfig(new ArgRunnable() {
public void run(JavaTask vm) {
StandardProject project = feature.getParent();
StandardProperties properties = project.getProperties();
// only check other properties if debugging is switched on
if (properties.getMainDebugProperty().getBooleanValue()) {
int port = properties.getMainDebugPort().getIntegerValue();
boolean suspend = properties.getMainDebugSuspend()
.getBooleanValue();
vm.initDebug(port, suspend);
String message = "debug mode configured at port " + port
+ " for the main vm";
project.getLogger().info(message);
}
}
});
}
/**
* Every time a command is executed we intervene in order to specify debug
* options.
*
* @param command
* the command instance that the advice is being applied to
*/
before(final Command command) : execution(* startVm(..)) && this(command) {
command.setStartVmConfig(new ArgRunnable() {
public void run(JavaTask vm) {
String name = command.getName();
StandardProject project = command.getStore().getParent();
StandardProperties properties = project.getProperties();
// only check other properties if debugging is switched on
if (properties.getCommandDebug(name).getBooleanValue()) {
int port = properties.getCommandDebugPort(name)
.getIntegerValue();
boolean suspend = properties
.getCommandDebugSuspend(name)
.getBooleanValue();
vm.initDebug(port, suspend);
String message = "debug mode configured at port " + port
+ " for command: " + name;
project.getLogger().info(message);
}
}
});
}
/**
* When the project is created, the logging level and console appender
* configuration is modified depending on the system properties in the
* property store.
*
* @param project
*/
after(StandardProject project) returning : execution(StandardProject.new(..)) && this(project) {
StandardProperties properties = project.getProperties();
ProtoLogger logger = project.getProtoLogger();
boolean quiet = properties.getQuiet().getBooleanValue();
logger.setConsoleAppenderEnabled(!quiet);
String level = properties.getLevel().getValue();
logger.setLevel(Level.toLevel(level));
}
/**
* Configures the DispatchFeature for showing the status window based on the
* PropertyStore statusWindowProperty value.
*
* @param feature
*/
after(StandardProject project) returning : execution(StandardProject.new(..)) && this(project) {
PropertyInfo statusWindow = project.getProperties().getStatusWindow();
project.getDispatchFeature().setStatusWindow(
statusWindow.getBooleanValue());
}
/**
* Intercepts the place where command memory is configured and replaces with
* the config property override if configured.
*
* @param name
* @param memory
* @return
*/
Command around(CommandStore store, String name, String memory) :
execution(* protoj..CommandStore.addCommand(String, String, *))
&& args(name, memory, *)
&& this(store) {
String propmem = store.getParent().getProperties()
.getCommandMemory(name).getValue();
String override = propmem.length() > 0 ? propmem : memory;
Logger logger = store.getParent().getLogger();
logger.info("setting maxmemory for \"" + name + "\" to " + override);
return proceed(store, name, override);
}
/**
* Intercepts the place where main memory is configured and replaces with
* the system property override if configured.
*
* @param name
* @param memory
* @return
*/
void around(DispatchFeature feature, String memory) :
execution(* protoj..DispatchFeature.initBootstrap(*, String))
&& args(*, memory)
&& this(feature) {
String propmem = feature.getParent().getProperties().getMainMemory()
.getValue();
String override = propmem.length() > 0 ? propmem : memory;
Logger logger = feature.getParent().getLogger();
logger.info("setting main maxmemory to " + override);
proceed(feature, override);
}
/**
* Intercepts the place where cross compilation is configured and
* substitutes replacements from the system property overrides if specified.
*
* @param name
* @param memory
* @return
*/
void around(CompileFeature feature, String target, String extdirs,
String bootclasspath) :
execution(* init*CrossCompile(String, String, String))
&& this(feature)
&& args(target, extdirs, bootclasspath) {
StandardProperties props = feature.getParent().getProperties();
String userTarget = props.getCompileTarget().getValue();
String userExtdirs = props.getCompileExtdirs().getValue();
String userBootclasspath = props.getCompileBootclasspath().getValue();
String newTarget = userTarget.length() > 0 ? userTarget : target;
String newExtdirs = userExtdirs.length() > 0 ? userExtdirs : extdirs;
String newBootclasspath = userBootclasspath.length() > 0 ? userBootclasspath
: bootclasspath;
Logger logger = feature.getParent().getLogger();
logger.info("overriding compile target: " + newTarget);
logger.info("overriding compile extdirs: " + newExtdirs);
logger.info("overriding compile bootclasspath: " + newBootclasspath);
proceed(feature, newTarget, newExtdirs, newBootclasspath);
}
/**
* Intercepts the place where compiler source is specified and applies the
* system property override if specified.
*
* @param feature
* @param source
*/
void around(CompileFeature feature, String source) :
execution(* init*Source(String))
&& this(feature) && args(source) {
StandardProperties props = feature.getParent().getProperties();
String userSource = props.getCompileSource().getValue();
String newSource = userSource.length() > 0 ? userSource : source;
Logger logger = feature.getParent().getLogger();
logger.info("overriding compile source: " + newSource);
proceed(feature, source);
}
/**
* Intercepts the place where compiler maximum memory is specified and applies the
* system property override if specified.
*
* @param feature
* @param source
*/
void around(CompileFeature feature, String memory) :
execution(* init*Memory(String))
&& this(feature) && args(memory) {
StandardProperties props = feature.getParent().getProperties();
String userMemory = props.getCompileMemory().getValue();
String newMemory = userMemory.length() > 0 ? userMemory : memory;
Logger logger = feature.getParent().getLogger();
logger.info("overriding compile memory: " + newMemory);
proceed(feature, memory);
}
/**
* Intercepts the place where junit memory is configured and replaces with
* the config property override if configured.
*
* @param project
* @param name
* @param memory
* @return
*/
void around(StandardProject project, JunitFeature feature, String memory) :
execution(protoj..JunitFeature.new(StandardProject, String))
&& args(project, memory)
&& this(feature) {
String propmem = project.getProperties().getJunitMemory().getValue();
String override = propmem.length() > 0 ? propmem : memory;
Logger logger = project.getLogger();
logger.info("setting junit maxmemory to " + override);
proceed(project, feature, override);
}
/**
* Intercepts the place where the directory profile is set up and
* replaces the directory location with a property override if any.
*
* @param config
* @param project
* @param home
* @param name
*/
void around(DirconfFeature config, StandardProject project, File home) :
execution(new(StandardProject, File))
&& this(config)
&& args(project, home) {
StandardProperties props = project.getProperties();
String homeOverride = props.getProfileHome().getValue();
File newHome = homeOverride.length() > 0 ? new File(homeOverride) : home;
proceed(config, project, newHome);
}
/**
* Intercepts the place where the scp profile is set up and
* replaces the url with a property override if any.
*
* @param config
* @param project
* @param dir
* @param name
*/
void around(ScpconfFeature config, StandardProject project, String url) :
execution(new(StandardProject, String))
&& this(config)
&& args(project, url) {
StandardProperties props = project.getProperties();
String urlOverride = props.getProfileUrl().getValue();
String newUrl = urlOverride.length() > 0 ? urlOverride : url;
proceed(config, project, newUrl);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy