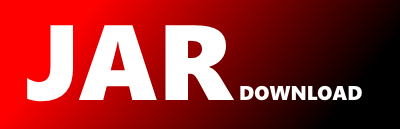
protoj.lang.internal.acme.AcmeSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.acme;
import java.io.File;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.lang.StandardProject;
import protoj.lang.internal.ProtoProject;
/**
* In a nutshell this represents the high level test that puts the acme project
* through its paces. It uses a {@link ScriptSession} to run a variety of
* commands and supplies matching listeners in order to verify correct
* functionality with assertions.
*
* @author Ashley Williams
*
*/
public final class AcmeSession {
/**
* See {@link #getProject()}.
*/
private StandardProject project;
/**
* After this constructor call the physical acme project will have been
* created.
*/
public AcmeSession() {
this.project = new ProtoProject().createSampleProject("acme");
}
/**
* Kicks off the command executions.
*/
public void execute() {
String acmeFile = getAcmeFile().getAbsolutePath();
ScriptSession session = project.createScriptSession();
session.addCommand("help", new AssertProjectHelp(this),
AssertProjectHelp.WARN);
session.addCommand("", new AssertCompile(this), AssertCompile.EMPTY);
session.addCommand("\"compile -i acme/util\"", new AssertCompile(this),
AssertCompile.UTIL);
session.addCommand("\"dirconf -name profile -interpolate\"",
new AssertDirConf(this), AssertDirConf.CONFIG);
session.addCommand("compile", new AssertCompile(this),
AssertCompile.ALL);
session.addCommand("fail", new AssertFail(this), null);
session.addCommand("retrieve", new AssertCompile(this),
AssertCompile.RETRIEVE);
session.addCommand("junit", new AssertJunit(this), null);
session.addCommand("test", new AssertJunit(this), null);
session.addCommand("help", new AssertProjectHelp(this),
AssertProjectHelp.OK);
session.addCommand("\"help compile\"", new AssertCommandHelp(this),
null);
session.addCommand("version", new AssertVersion(this), null);
session.addCommand("scp", new AssertScp(this),
AssertScp.MANDATORY_ARCHIVE_OPTION);
session.addCommand("\"scp -arc wibble\"", new AssertScp(this),
AssertScp.MANDATORY_DIR_OPTION);
session.addCommand("\"scp -arc wibble -dir a@http://b:c -pass b\"",
new AssertScp(this), AssertScp.BAD_ARCHIVE);
session.addCommand("\"scp -arc foo -dir a@http://b:c -pass p\"",
new AssertScp(this), AssertScp.MISSING_ARCHIVE);
session.addCommand("archive", new AssertArchive(this), null);
session.addCommand("\"publish -artifact foo\"",
new AssertPublish(this), AssertPublish.FOO);
session.addCommand("publish", new AssertPublish(this),
AssertPublish.ALL);
session.addCommand("verify-tar", new AssertVerifyTar(this),
AssertVerifyTar.NO_TAR);
session.addCommand("\"tar -nosrc\"", new AssertTar(this), null);
session.addCommand("verify-tar", new AssertVerifyTar(this),
AssertVerifyTar.NO_SRC);
session.addCommand("tar", new AssertTar(this), null);
session.addCommand("verify-tar", new AssertVerifyTar(this),
AssertVerifyTar.OK);
session.addCommand("\"scp -arc foo -dir a@http://b:c\"", new AssertScp(
this), AssertScp.OK);
session.addCommand("\"scp -arc foo -dir a@http://b:c -pass p\"",
new AssertScp(this), AssertScp.OK);
session.addCommand("\"scp -arc foo -dir a@http://b:c -key a\"",
new AssertScp(this), AssertScp.OK);
session.addCommand(
"\"scp -arc foo -dir a@http://b:c -key a -phrase a\"",
new AssertScp(this), AssertScp.OK);
session.addCommand(String.format("create-file \"opts -Dacme.file=%s\"",
acmeFile), new AssertJvmarg(this), null);
session
.addCommand(
"help \"opts -Dprotoj.debug.help -Dprotoj.debug.port.help=12345\"",
new AssertDebug(this), AssertDebug.HELP_PORT_12345);
session.addCommand("help \"opts -Dprotoj.debug.help\"",
new AssertDebug(this), AssertDebug.HELP_PORT_DEFAULT);
session.addCommand("help \"opts -Dprotoj.debug.version\"",
new AssertDebug(this), AssertDebug.HELP_WRONG_CMD);
session
.addCommand(
"junit \"opts -Dprotoj.junit.debug -Dprotoj.junit.debug.port=12345\"",
new AssertDebug(this), AssertDebug.JUNIT_PORT_12345);
session.addCommand("junit \"opts -Dprotoj.junit.debug\"",
new AssertDebug(this), AssertDebug.JUNIT_PORT_DEFAULT);
session.addCommand("message \"opts -Dprotoj.junit.debug\"",
new AssertDebug(this), AssertDebug.JUNIT_WRONG_CMD);
session
.addCommand(
"message \"opts -Dprotoj.main.debug -Dprotoj.main.debug.port=12345\"",
new AssertDebug(this), AssertDebug.MAIN_PORT_12345);
session.addCommand("message \"opts -Dprotoj.main.debug\"",
new AssertDebug(this), AssertDebug.MAIN_PORT_DEFAULT);
session.addCommand("\"dirconf -name profile -clean\"",
new AssertDirConf(this), AssertDirConf.UNDO);
session.addCommand("clean", new AssertClean(this), null);
session.execute();
}
/**
* Similar to the real acme project.
*
* @return
*/
public StandardProject getProject() {
return project;
}
/**
* Represents a file that should exist after the corresponding command has
* been executed.
*
* @return
*/
public File getAcmeFile() {
ProjectLayout acmeLayout = getProject().getLayout();
return new File(acmeLayout.getTargetDir(), "testvmarg.txt");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy