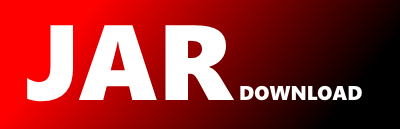
protoj.lang.internal.acme.AssertArchive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.acme;
import java.io.File;
import java.util.jar.Attributes;
import java.util.jar.Manifest;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.lang.internal.VerifyArchive;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Triggered when the assemble command is specified. See the individual methods
* to determine what is being tested.
*
* @author Ashley Williams
*
*/
final class AssertArchive implements ArgRunnable {
private final AcmeSession acmeSession;
public AssertArchive(AcmeSession acmeSession) {
this.acmeSession = acmeSession;
}
public void run(ScriptSession projectSession) {
assertAcmeClassesJar();
assertFooClassesJar();
assertFooSourcesJar();
assertFooJavadocJar();
assertLogContents();
assertAdvice(projectSession);
CommandTask exec = projectSession.getCurrentExec();
Assert.assertTrue(exec.getResult(), exec.isSuccess());
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
acmeLayout.getLogFile().delete();
}
/**
* Asserts that advice was applied during load time weaving.
*
* @param projectSession
*/
private void assertAdvice(ScriptSession projectSession) {
String stdout = projectSession.getCurrentExec().getStdout();
String expected = "before advice triggered at StandardProject.assemble execution join point";
Assert.assertTrue(stdout, stdout.contains(expected));
}
/**
* Asserts that the build sent the expected text to the log file.
*/
private void assertLogContents() {
String log = acmeSession.getProject().getLayout().loadLog();
Assert.assertTrue(log, log.contains("archive"));
Assert.assertTrue(log, log.contains("Task \"archive\" started"));
Assert.assertTrue(log, log.contains("Building jar:"));
Assert.assertTrue(log, log.contains("acme-1.0.jar"));
Assert.assertTrue(log, log.contains("foo.jar"));
Assert.assertTrue(log, log.contains("Task \"archive\" finished"));
}
/**
* Asserts the contents of the foo classes jar is as expected.
*/
private void assertFooClassesJar() {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
File jar = new File(acmeLayout.getArchiveDir(), "foo.jar");
VerifyArchive verify = new VerifyArchive(jar);
verify.initIncludedResources("test.txt");
verify.initExcludedResources("acme/AcmeProject.class",
"acme/core/AcmeCore.class");
verify.execute();
Manifest manifest = verify.getManifest();
// this attribute was hard-coded in file
Assert
.assertTrue(manifest.getMainAttributes().values().contains(
"foo"));
// this attribute was added programmatically
Assert
.assertTrue(manifest.getMainAttributes().values().contains(
"bar"));
}
/**
* Asserts the contents of the acme classes jar is as expected.
*/
private void assertAcmeClassesJar() {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
// acme has been marked as a classpath jar, so it should be in lib/
File libJar = new File(acmeLayout.getLibDir(), "acme-1.0.jar");
Assert.assertTrue(libJar.exists());
File jar = new File(acmeLayout.getArchiveDir(), "acme-1.0.jar");
VerifyArchive verify = new VerifyArchive(jar);
verify.initIncludedResources("acme/AcmeProject.class",
"acme/core/AcmeCore.class", "test.txt", "org/junit/Test.class");
verify.execute();
Attributes mainAttributes = verify.getManifest().getMainAttributes();
Assert.assertFalse(mainAttributes.values().contains("foo"));
Assert.assertTrue(mainAttributes
.containsKey(Attributes.Name.CLASS_PATH));
String classpathValue = mainAttributes
.getValue(Attributes.Name.CLASS_PATH);
Assert.assertTrue(classpathValue, classpathValue
.contains("aspectjrt.jar"));
Assert.assertFalse(
"junit jar merged so shouldn't be in manifest classpath",
classpathValue.contains("junit-4.4.jar"));
Assert.assertTrue(mainAttributes
.containsKey(Attributes.Name.MAIN_CLASS));
String mainClassValue = mainAttributes
.getValue(Attributes.Name.MAIN_CLASS);
Assert.assertEquals(mainClassValue, "acme.AcmeProject");
}
/**
* Asserts the contents of the foo sources jar is as expected.
*/
private void assertFooSourcesJar() {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
File jar = new File(acmeLayout.getArchiveDir(),
"foosources-sources.jar");
VerifyArchive verify = new VerifyArchive(jar);
verify.initIncludedResources("acme/AcmeProject.java",
"org/junit/Test.java");
verify.execute();
}
/**
* Asserts the contents of the foo javadoc jar is as expected.
*/
private void assertFooJavadocJar() {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
File jar = new File(acmeLayout.getArchiveDir(),
"foojavadoc-javadoc.jar");
VerifyArchive verify = new VerifyArchive(jar);
verify.initIncludedResources("acme/AcmeProject.html");
verify.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy