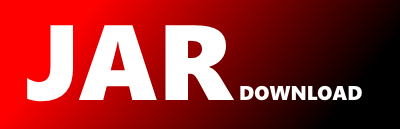
protoj.lang.internal.acme.AssertCompile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.acme;
import java.io.File;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.util.ArgRunnable;
/**
* See the conditional logic for the different types of tag name.
*
* @author Ashley Williams
*
*/
final class AssertCompile implements ArgRunnable {
public static final String EMPTY = "empty";
public static final String ALL = "all";
public static final String UTIL = "util";
public static final String RETRIEVE = "retrieve";
private final AcmeSession acmeSession;
public AssertCompile(AcmeSession acmeSession) {
this.acmeSession = acmeSession;
}
public void run(ScriptSession projectSession) {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
String log = acmeSession.getProject().getLayout().loadLog();
String tag = projectSession.getCurrentTag();
File acmeProjectClass = new File(acmeLayout.getClassesDir(),
"acme/AcmeProject.class");
File acmeUtilClass = new File(acmeLayout.getClassesDir(),
"acme/util/AcmeUtil.class");
File langSourcesJar = new File(acmeLayout.getLibDir(),
"commons-lang-2.4-sources.jar");
File httpClassesJar = new File(acmeLayout.getLibDir(),
"httpclient-4.0-beta2.jar");
File resource = new File(acmeLayout.getClassesDir(), "test.txt");
if (tag.equals(EMPTY)) {
// checks that before the compiler is executed, just the
// core classes are already compiled
Assert.assertFalse("shouldn't find "
+ acmeUtilClass.getAbsolutePath(), acmeUtilClass.exists());
Assert.assertFalse("shouldn't find "
+ acmeProjectClass.getAbsolutePath(), acmeProjectClass
.exists());
Assert
.assertFalse("shouldn't find "
+ httpClassesJar.getAbsolutePath(), httpClassesJar
.exists());
Assert.assertFalse("shouldn't find "
+ langSourcesJar.getAbsolutePath(), langSourcesJar
.exists());
} else if (tag.equals(RETRIEVE)) {
// checks that when the ivy command is executed, the http source jar
// should be downloaded since it was added to the dependencies
// store, but not commons-lang since it was taken away
Assert
.assertTrue("couldn't find "
+ httpClassesJar.getAbsolutePath(), httpClassesJar
.exists());
Assert.assertFalse("shouldn't find "
+ langSourcesJar.getAbsolutePath(), langSourcesJar
.exists());
} else if (tag.equals(UTIL)) {
// checks that when the util includes pattern is specified
// then just that package should be compiled
Assert.assertTrue("couldn't find "
+ acmeUtilClass.getAbsolutePath(), acmeUtilClass.exists());
Assert.assertFalse("shouldn't find "
+ acmeProjectClass.getAbsolutePath(), acmeProjectClass
.exists());
// the compiler settings should be as they were in the AcmeCore as
// the config hasn't been executed yet containing the overrides
Assert.assertTrue(log, log
.contains("overriding compile memory: 16m"));
Assert.assertTrue(log, log
.contains("overriding compile source: 1.5"));
} else if (tag.equals(ALL)) {
// checks that when no includes pattern is provided,
// all the classes should be compiled and also that
// the resources are copied into the classes directory
Assert.assertTrue("couldn't find "
+ acmeUtilClass.getAbsolutePath(), acmeUtilClass.exists());
Assert.assertTrue("couldn't find "
+ acmeProjectClass.getAbsolutePath(), acmeProjectClass
.exists());
Assert.assertTrue("couldn't find " + resource.getAbsolutePath(),
resource.exists());
Assert.assertTrue(log, log.contains("compile"));
Assert.assertTrue(log, log.contains("Task \"ajc\" started"));
Assert.assertTrue(log, log.contains("Task \"ajc\" finished"));
// the compiler settings should be as they were in the acme
// properties file since the config command has now been executed
Assert.assertTrue(log, log
.contains("overriding compile memory: 18m"));
Assert
.assertTrue(log, log
.contains("overriding compile source: 5"));
}
Assert.assertTrue(projectSession.getCurrentExec().isSuccess());
acmeLayout.getLogFile().delete();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy