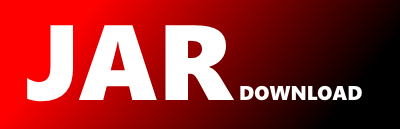
protoj.lang.internal.acme.AssertDebug Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.acme;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.util.ArgRunnable;
/**
* These assertions trigger when the various debug properties are specified and
* ensures that they perform as described in the project help.
*
* @author Ashley Williams
*
*/
final class AssertDebug implements ArgRunnable {
public static final String HELP_PORT_12345 = "help port 12345";
public static final String HELP_PORT_DEFAULT = "help port default";
public static final String HELP_WRONG_CMD = "help wrong command";
public static final String JUNIT_PORT_12345 = "junit port 12345";
public static final String JUNIT_PORT_DEFAULT = "junit port default";
public static final String JUNIT_WRONG_CMD = "junit wrong command";
public static final String MAIN_PORT_12345 = "main port 12345";
public static final String MAIN_PORT_DEFAULT = "main port default";
private final AcmeSession acmeSession;
/**
* This listener is attached to more than one debug command so we can keep
* track of which one with this testIndex. Changing the order of the
* commands in {@link AcmeSession} would break these assertion tests.
*/
private int testIndex = 0;
public AssertDebug(AcmeSession acmeSession) {
this.acmeSession = acmeSession;
}
public void run(ScriptSession projectSession) {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
String tag = projectSession.getCurrentTag();
String stdout = projectSession.getCurrentExec().getStdout();
String log = acmeSession.getProject().getLayout().loadLog();
if (tag.equals(HELP_PORT_12345)) {
// debug session detected at the 12345 port when specified
Assert
.assertTrue(
stdout,
stdout
.contains("Listening for transport dt_socket at address: 12345"));
Assert
.assertTrue(
log,
log
.contains("debug mode configured at port 12345 for command: help"));
} else if (tag.equals(HELP_PORT_DEFAULT)) {
// debug session detected at the default port when not specified
Assert
.assertTrue(
stdout,
stdout
.contains("Listening for transport dt_socket at address: 11111"));
Assert
.assertTrue(
log,
log
.contains("debug mode configured at port 11111 for command: help"));
} else if (tag.equals(HELP_WRONG_CMD)) {
// no debug session when not targeting the 'help command' vm
Assert
.assertFalse(
stdout,
stdout
.contains("Listening for transport dt_socket at address: "));
} else if (tag.equals(JUNIT_PORT_12345)) {
// debug session detected when targeting the junit test vm
Assert
.assertTrue(
stdout,
stdout
.contains("Listening for transport dt_socket at address: 12345"));
Assert
.assertTrue(
stdout,
stdout
.contains("debug mode configured at port 12345 for the junit vm"));
} else if (tag.equals(JUNIT_PORT_DEFAULT)) {
// debug session detected when targeting the junit test vm
Assert
.assertTrue(
stdout,
stdout
.contains("Listening for transport dt_socket at address: 11111"));
Assert
.assertTrue(
stdout,
stdout
.contains("debug mode configured at port 11111 for the junit vm"));
} else if (tag.equals(JUNIT_WRONG_CMD)) {
// a debug session is detected when targeting the project vm
// which is achieved by executing a command not known in the
// core vm - basically any newly added commands in the project vm
Assert
.assertFalse(
stdout,
stdout
.contains("Listening for transport dt_socket at address: "));
} else if (tag.equals(MAIN_PORT_12345)) {
// debug session detected when targeting the main vm
Assert
.assertTrue(
stdout,
stdout
.contains("Listening for transport dt_socket at address: 12345"));
Assert
.assertTrue(
stdout,
stdout
.contains("debug mode configured at port 12345 for the main vm"));
} else if (tag.equals(MAIN_PORT_DEFAULT)) {
// debug session detected when targeting the main vm
Assert
.assertTrue(
stdout,
stdout
.contains("Listening for transport dt_socket at address: 11111"));
Assert
.assertTrue(
stdout,
stdout
.contains("debug mode configured at port 11111 for the main vm"));
}
acmeLayout.getLogFile().delete();
testIndex++;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy