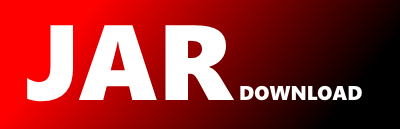
protoj.lang.internal.acme.AssertPublish Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
package protoj.lang.internal.acme;
import java.io.File;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Triggered when the publish command is specified. See the individual methods
* to determine what is being tested.
*
* @author Ashley Williams
*
*/
public final class AssertPublish implements ArgRunnable {
private final AcmeSession acmeSession;
public static final String ALL = "all";
public static final String FOO = "foo";
public AssertPublish(AcmeSession acmeSession) {
this.acmeSession = acmeSession;
}
public void run(ScriptSession projectSession) {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
File fooRepoDir = new File(acmeLayout.getTargetDir(),
"repo/org/acme/foo/1.0");
File fooPom = new File(fooRepoDir, "foo-1.0.pom");
File fooClasses = new File(fooRepoDir, "foo-1.0.jar");
File acmeRepoDir = new File(acmeLayout.getTargetDir(),
"repo/org/acme/acme/1.0");
File acmePom = new File(acmeRepoDir, "acme-1.0.pom");
File acmeClasses = new File(acmeRepoDir, "acme-1.0.jar");
File acmeSources = new File(acmeRepoDir, "acme-1.0-sources.jar");
File acmeJavadoc = new File(acmeRepoDir, "acme-1.0-javadoc.jar");
String tag = projectSession.getCurrentTag();
if (tag.equals(FOO)) {
// the publish command should only have generated foo artifacts
assertExists(fooPom, true);
assertExists(fooClasses, true);
assertArtifacts(acmePom, false);
assertArtifacts(acmeClasses, false);
assertArtifacts(acmeSources, false);
assertArtifacts(acmeJavadoc, false);
} else {
// the publish command should also have generated acme artifacts
assertArtifacts(acmePom, true);
assertArtifacts(acmeClasses, true);
assertArtifacts(acmeSources, true);
assertArtifacts(acmeJavadoc, true);
}
CommandTask exec = projectSession.getCurrentExec();
Assert.assertTrue(exec.getResult(), exec.isSuccess());
acmeLayout.getLogFile().delete();
}
/**
* Asserts that the given artifact and its associated signature, digest and
* checksum files all exist or don't exist depending on the value of the
* exists parameter.
*
* @param artifact
* @param exists
*/
private void assertArtifacts(File artifact, boolean exists) {
File repoDir = artifact.getParentFile();
String name = artifact.getName();
File artifactChecksum = new File(repoDir, name + ".md5");
File artifactDigest = new File(repoDir, name + ".sha1");
File artifactSig = new File(repoDir, name + ".asc");
File artifactSigChecksum = new File(repoDir, name + ".asc.md5");
File artifactSigDigest = new File(repoDir, name + ".asc.sha1");
assertExists(artifact, exists);
assertExists(artifactChecksum, exists);
assertExists(artifactDigest, exists);
assertExists(artifactSig, exists);
assertExists(artifactSigChecksum, exists);
assertExists(artifactSigDigest, exists);
}
/**
* Asserts the given file exists or doesn't exist depending on the value of
* the exists parameter.
*
* @param file
* @param exists
*/
private void assertExists(File file, boolean exists) {
if (exists) {
String message = "couldn't find " + file.getAbsolutePath();
Assert.assertTrue(message, file.exists());
} else {
String message = "shouldn't have found " + file.getAbsolutePath();
Assert.assertFalse(message, file.exists());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy