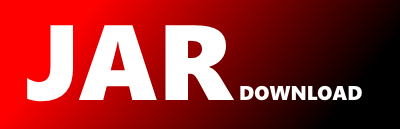
protoj.lang.internal.acme.AssertTar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.acme;
import java.io.File;
import org.apache.commons.io.FileUtils;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.GUnzip;
import org.apache.tools.ant.taskdefs.Untar;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.util.AntTarget;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* These assertions trigger when the tar command is specified and ensures that
* the correct information is sent to stdout and not the log file. We ensure
* that the includes and excludes settings are respected and also that the
* -nosrc argument results in the source directory being excluded.
*
* @author Ashley Williams
*
*/
final class AssertTar implements ArgRunnable {
private final AcmeSession acmeSession;
public AssertTar(AcmeSession acmeSession) {
this.acmeSession = acmeSession;
}
public void run(ScriptSession projectSession) {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
String log = acmeSession.getProject().getLayout().loadLog();
File acmeTargetDir = acmeLayout.getTargetDir();
File extractDir = new File(acmeTargetDir, "assert-tar");
File acmeDir = new File(extractDir, "acme-1.0");
File logDir = new File(acmeDir, "log");
File binDir = new File(acmeDir, "bin");
File confDir = new File(acmeDir, "conf");
File propertiesFile = new File(confDir, "all.project.properties");
File docsDir = new File(acmeDir, "docs");
File protoLogFile = new File(logDir, "protoj.log");
File acmeLogFile = new File(logDir, "acme.log");
File libDir = new File(acmeDir, "lib");
File javadocJar = new File(libDir, "junit-4.4-javadoc.jar");
File sourcesJar = new File(libDir, "junit-4.4-sources.jar");
File aspectJar = new File(libDir, "aspectjrt.jar");
File targetDir = new File(acmeDir, "target");
File classesDir = new File(acmeDir, "classes");
File srcDir = new File(acmeDir, "src");
File privateDir = new File(acmeDir, "private");
File redFile = new File(privateDir, "red.txt");
File partPrivateDir = new File(acmeDir, "part-private");
File amberFile = new File(partPrivateDir, "amber.txt");
File greenFile = new File(partPrivateDir, "green.txt");
Assert.assertTrue(log, log.contains("tar"));
CommandTask exec = projectSession.getCurrentExec();
Assert.assertTrue(exec.getResult(), exec.isSuccess());
extractTarFiles(projectSession, extractDir);
Assert.assertTrue(acmeDir.exists());
Assert.assertTrue(binDir.exists());
Assert.assertTrue(confDir.exists());
Assert.assertFalse(propertiesFile.exists());
Assert.assertTrue(docsDir.exists());
Assert.assertTrue(libDir.exists());
Assert.assertFalse(javadocJar.exists());
Assert.assertFalse(sourcesJar.exists());
Assert.assertTrue(logDir.exists());
Assert.assertTrue(acmeLogFile.exists());
Assert.assertFalse(protoLogFile.exists());
Assert.assertTrue(aspectJar.exists());
Assert.assertFalse(targetDir.exists());
Assert.assertFalse(classesDir.exists());
if (isNosrcSpecified(projectSession)) {
Assert.assertFalse(srcDir.exists());
} else {
Assert.assertTrue(srcDir.exists());
}
Assert.assertFalse(privateDir.exists());
Assert.assertFalse(redFile.exists());
Assert.assertTrue(partPrivateDir.exists());
Assert.assertFalse(greenFile.exists());
Assert.assertTrue(amberFile.exists());
FileUtils.deleteDirectory(extractDir);
acmeLayout.getLogFile().delete();
}
/**
* Extracts the given tar file to the target directory.
*
* @param projectSession
* @param extractDir
*
* @param gzFile
* @param tarFile
*/
private void extractTarFiles(ScriptSession projectSession, File extractDir) {
ProjectLayout acmeLayout = acmeSession.getProject().getLayout();
extractDir.mkdirs();
File acmeArchiveDir = acmeLayout.getArchiveDir();
AntTarget target = new AntTarget("protoj-acme-gunzip");
File gzFile = new File(acmeArchiveDir, "acme.tar.gz");
File tarFile = new File(acmeArchiveDir, "acme.tar");
target.initLogging(Project.MSG_INFO);
GUnzip gunzip = new GUnzip();
gunzip.setTaskName("gunzip");
target.addTask(gunzip);
gunzip.setSrc(gzFile);
Untar untar = new Untar();
untar.setTaskName("untar");
untar.setSrc(tarFile);
target.addTask(untar);
untar.setDest(extractDir);
target.execute();
}
private boolean isNosrcSpecified(ScriptSession projectSession) {
String currentCommand = projectSession.getCurrentCommand();
return currentCommand.contains("-nosrc");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy