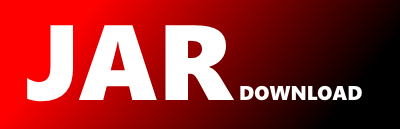
protoj.lang.internal.sample.AjcSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.sample;
import java.io.File;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.lang.StandardProject;
import protoj.lang.internal.ProtoProject;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Executes a selection of commands against the ajc project and performs various
* asserts against their results.
*
* @author Ashley Williams
*
*/
public final class AjcSession {
private static final String CONFIG = "config";
private static final String EMPTY = "empty";
private static final String CLEAN = "clean";
private static final String COMPILE = "compile";
private static final String RETRIEVE = "retrieve";
private static final String HELLO_AJC = "hello-ajc";
private static final String HELP = "help";
private StandardProject project;
/**
* After this constructor call the physical ajc project will have been
* created.
*/
public AjcSession() {
this.project = new ProtoProject().createSampleProject("ajc");
}
public void execute() {
ScriptSession session = project.createScriptSession();
ArgRunnableImplementation listener = new ArgRunnableImplementation();
session.addCommand("", listener, EMPTY);
session.addCommand("\"dirconf -name profile\"", listener, CONFIG);
session.addCommand("retrieve", listener, RETRIEVE);
session.addCommand("compile", listener, COMPILE);
session.addCommand("help", listener, HELP);
session.addCommand("hello-ajc", listener, HELLO_AJC);
session.addCommand("clean", listener, CLEAN);
session.execute();
}
private final class ArgRunnableImplementation implements
ArgRunnable {
public void run(ScriptSession session) {
ProjectLayout layout = project.getLayout();
CommandTask exec = session.getCurrentExec();
String stdout = exec.getStdout();
project.getLogger().debug(stdout);
String log = project.getLayout().loadLog();
File ajcProjectClass = new File(layout.getClassesDir(),
"org/ajc/system/AjcProject.class");
File ajcCoreClass = new File(layout.getClassesDir(),
"org/ajc/core/AjcCore.class");
File ivyFile = new File(layout.getClassesDir(), "ajc/ivy.xml");
File aopFile = new File(layout.getClassesDir(), "META-INF/aop.xml");
String tag = session.getCurrentTag();
if (tag.equals(RETRIEVE)) {
File libDir = layout.getLibDir();
File sources = new File(libDir, "junit-4.5-sources.jar");
File classes = new File(libDir, "junit-4.5.jar");
Assert.assertTrue(sources.exists());
Assert.assertTrue(classes.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(CONFIG)) {
File appProps = new File(layout.getConfDir(), "app.properties");
File projProps = new File(layout.getConfDir(), "ajc.properties");
Assert.assertTrue(appProps.exists());
Assert.assertTrue(projProps.exists());
} else if (tag.equals(EMPTY)) {
Assert.assertTrue(ajcCoreClass.exists());
Assert.assertTrue(ivyFile.exists());
// the ajc project class should not be compiled yet
Assert.assertFalse(ajcProjectClass.exists());
// the aop.xml file should be left out of the javac copy
Assert.assertFalse(aopFile.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(COMPILE)) {
Assert.assertTrue(ajcProjectClass.exists());
Assert.assertTrue(ajcCoreClass.exists());
Assert.assertTrue(ivyFile.exists());
Assert.assertTrue(aopFile.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(HELP)) {
Assert.assertTrue(stdout, stdout.contains("hello-ajc"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(HELLO_AJC)) {
Assert.assertTrue(stdout, stdout
.contains("hello from this sample project!"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(CLEAN)) {
Assert.assertTrue(stdout, stdout
.contains("load time weaving appears to work"));
Assert.assertTrue(log, exec.isSuccess());
}
layout.getLogFile().delete();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy