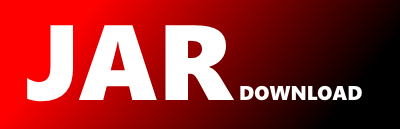
protoj.lang.internal.sample.AlienSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.sample;
import java.io.File;
import org.apache.commons.configuration.PropertiesConfiguration;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.lang.StandardProject;
import protoj.lang.internal.ProtoProject;
import protoj.lang.internal.VerifyArchive;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Executes a selection of commands against the Alien project and performs
* various asserts against their results.
*
* @author Ashley Williams
*
*/
public final class AlienSession {
private static final String RETRIEVE = "retrieve";
private static final String EMPTY = "empty";
private static final String UNDO = "undo";
private static final String COMPILE = "compile";
private static final String CONFIG = "config";
private static final String CLEAN = "clean";
private static final String TEST = "test";
private static final String FIND_ALIEN = "find alien";
private static final String ARCHIVE = "archive";
private static final String NO_JAR = "no jar";
private static final String VERSION = "version";
private static final String COMMAND_HELP = "command-help";
private static final String PROJECT_HELP = "project-help";
private StandardProject project;
/**
* After this constructor call the physical alien project will have been
* created.
*/
public AlienSession() {
this.project = new ProtoProject().createSampleProject("alien");
}
public void execute() {
ScriptSession session = project.createScriptSession();
ArgRunnableImplementation listener = new ArgRunnableImplementation();
session.addCommand("", listener, EMPTY);
session.addCommand("\"dirconf -name profile\"", listener, CONFIG);
session.addCommand("compile", listener, COMPILE);
session.addCommand("help", listener, PROJECT_HELP);
session.addCommand("\"help test\"", listener, COMMAND_HELP);
session.addCommand("version", listener, VERSION);
session.addCommand("retrieve", listener, RETRIEVE);
session.addCommand("archive", listener, ARCHIVE);
session
.addCommand("\"find-alien -gx milky-way\"", listener,
FIND_ALIEN);
session.addCommand("test", listener, TEST);
session.addCommand("\"dirconf -name profile -clean\"", listener, UNDO);
session.addCommand("clean", listener, CLEAN);
session.execute();
}
private final class ArgRunnableImplementation implements
ArgRunnable {
public void run(ScriptSession session) {
ProjectLayout layout = project.getLayout();
CommandTask exec = session.getCurrentExec();
String stdout = exec.getStdout();
project.getLogger().debug(stdout);
String log = project.getLayout().loadLog();
File alienProjectClass = new File(layout.getClassesDir(),
"alien/AlienProject.class");
File testReport = new File(layout.getJunitReportsDir(),
"TEST-alien.test.AlienTest.txt");
String tag = session.getCurrentTag();
if (tag.equals(CLEAN)) {
// generated resources are deleted
Assert.assertFalse(layout.getTargetDir().exists());
Assert.assertFalse(layout.getClassesDir().exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(EMPTY)) {
// at least one non-core class hasn't been created
// since compile command hasn't yet been executed
Assert.assertFalse(alienProjectClass.exists());
} else if (tag.equals(UNDO)) {
// check doc file got deleted
File topSecretFile = new File(layout.getDocsDir(),
"topsecret.txt");
Assert.assertTrue(!topSecretFile.exists());
// check properties file got deleted
File alien = new File(layout.getConfDir(),
"alien.test.properties");
Assert.assertTrue(!alien.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(TEST)) {
// the test report has been created
Assert.assertTrue(testReport.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(RETRIEVE)) {
// the junit jars have been downloaded into the lib directory
File libDir = layout.getLibDir();
File sourcesJar = new File(libDir, "junit-4.5-sources.jar");
File classesJar = new File(libDir, "junit-4.5.jar");
Assert.assertTrue(sourcesJar.exists());
Assert.assertTrue(classesJar.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(FIND_ALIEN)) {
// the test report has been created
Assert.assertTrue(stdout, stdout
.contains("No life found in the milky-way"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(ARCHIVE)) {
File classesJar = new File(layout.getArchiveDir(), "alien.jar");
verifyContents(classesJar, "alien/core/AlienCore.class",
"alien/AlienProject.class");
File javadocJar = new File(layout.getArchiveDir(),
"alien-javadoc.jar");
verifyContents(javadocJar, "index.html",
"alien/core/AlienCore.html", "alien/AlienProject.html");
File sourcesJar = new File(layout.getArchiveDir(),
"alien-sources.jar");
verifyContents(sourcesJar, "alien/core/AlienCore.java",
"alien/AlienProject.java");
// the jar file has been created
Assert.assertTrue(classesJar.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(NO_JAR)) {
// google upload should fail if there is no jar
Assert.assertTrue(log, log.contains("no jar file"));
Assert.assertFalse(log, exec.isSuccess());
} else if (tag.equals(CONFIG)) {
File appProps = new File(layout.getConfDir(), "app.properties");
File projProps = new File(layout.getConfDir(),
"alien.properties");
Assert.assertTrue(appProps.exists());
Assert.assertTrue(projProps.exists());
// check profile text file got copied ok
File topSecretFile = new File(layout.getDocsDir(),
"topsecret.txt");
Assert.assertTrue(topSecretFile.exists());
// check properties file merge worked
File alien = new File(layout.getConfDir(),
"alien.test.properties");
Assert.assertTrue(alien.exists());
PropertiesConfiguration config = new PropertiesConfiguration(
alien);
Assert.assertTrue(config.containsKey("galaxy"));
Assert.assertEquals(config.getString("galaxy"), "milky-way");
Assert.assertTrue(config.containsKey("planet"));
Assert.assertEquals(config.getString("planet"), "venus");
Assert.assertTrue(config.containsKey("side"));
Assert.assertEquals(config.getString("side"), "dark");
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(COMPILE)) {
// at least one non-core class has been created
Assert.assertTrue(alienProjectClass.exists());
} else if (tag.equals(VERSION)) {
// the version string is as expected
Assert.assertTrue(stdout, stdout.contains("1.0"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(COMMAND_HELP)) {
// the project help is as expected
Assert.assertTrue(stdout, stdout
.contains("Executes the junit tests"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(PROJECT_HELP)) {
Assert.assertTrue(stdout, stdout.contains("find-alien"));
Assert.assertTrue(log, exec.isSuccess());
}
layout.getLogFile().delete();
}
}
/**
* Asserts that the given jar file exists and contains resources as listed
* by the names parameter.
*
* @param jar
* @param names
*/
private void verifyContents(File jar, String... names) {
VerifyArchive verifyArchive = new VerifyArchive(jar);
verifyArchive.initIncludedResources(names);
verifyArchive.execute();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy