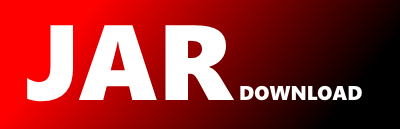
protoj.lang.internal.sample.HelloWorldSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.sample;
import java.io.File;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.lang.StandardProject;
import protoj.lang.internal.ProtoProject;
import protoj.lang.internal.VerifyArchive;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Executes a selection of commands against the HelloWorld project and performs
* various asserts against their results.
*
* @author Ashley Williams
*
*/
public final class HelloWorldSession {
private static final String CONFIG = "config";
private static final String CLEAN = "clean";
private static final String HELLO_WORLD = "hello world";
private static final String VERIFY_TAR = "verify-tar";
private static final String TAR = "tar";
private static final String TEST = "test";
private static final String ARCHIVE = "archive";
private static final String VERSION = "version";
private static final String COMMAND_HELP = "command-help";
private static final String PROJECT_HELP = "project-help";
private StandardProject project;
/**
* been created.
*/
public HelloWorldSession() {
this.project = new ProtoProject().createSampleProject("helloworld");
}
public void execute() {
ScriptSession session = project.createScriptSession();
ArgRunnableImplementation listener = new ArgRunnableImplementation();
session.addCommand("\"dirconf -name profile\"", listener, CONFIG);
session.addCommand("help", listener, PROJECT_HELP);
session.addCommand("\"help test\"", listener, COMMAND_HELP);
session.addCommand("version", listener, VERSION);
session.addCommand("archive", listener, ARCHIVE);
session.addCommand("test", listener, TEST);
session.addCommand("tar", listener, TAR);
session.addCommand("verify-tar", listener, VERIFY_TAR);
session.addCommand("hello-world", listener, HELLO_WORLD);
session.addCommand("clean", listener, CLEAN);
session.execute();
}
private final class ArgRunnableImplementation implements
ArgRunnable {
public void run(ScriptSession session) {
ProjectLayout layout = project.getLayout();
CommandTask exec = session.getCurrentExec();
String stdout = exec.getStdout();
project.getLogger().debug(stdout);
String log = project.getLayout().loadLog();
String tag = session.getCurrentTag();
if (tag.equals(CLEAN)) {
Assert.assertFalse(layout.getTargetDir().exists());
Assert.assertFalse(layout.getClassesDir().exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(HELLO_WORLD)) {
Assert.assertTrue(stdout, stdout.contains("Hello World!"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(CONFIG)) {
File appProps = new File(layout.getConfDir(), "app.properties");
File projProps = new File(layout.getConfDir(),
"helloworld.properties");
Assert.assertTrue(appProps.exists());
Assert.assertTrue(projProps.exists());
} else if (tag.equals(VERIFY_TAR)) {
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(TAR)) {
File tarFile = new File(layout.getArchiveDir(),
"hello-world.tar.gz");
Assert.assertTrue(tarFile.exists());
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(TEST)) {
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(ARCHIVE)) {
File classesJar = new File(layout.getArchiveDir(),
"hello-world-9.9.jar");
verifyContents(classesJar, "helloworld/HelloWorld.class");
File javadocJar = new File(layout.getArchiveDir(),
"hello-world-9.9-javadoc.jar");
verifyContents(javadocJar, "index.html",
"helloworld/HelloWorld.html");
File sourcesJar = new File(layout.getArchiveDir(),
"hello-world-9.9-sources.jar");
verifyContents(sourcesJar, "helloworld/HelloWorld.java");
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(VERSION)) {
Assert.assertTrue(stdout, stdout.contains("9.9"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(COMMAND_HELP)) {
Assert.assertTrue(stdout, stdout
.contains("Executes the junit tests"));
Assert.assertTrue(log, exec.isSuccess());
} else if (tag.equals(PROJECT_HELP)) {
Assert
.assertTrue(stdout, stdout
.contains("Available commands"));
Assert.assertTrue(log, exec.isSuccess());
}
layout.getLogFile().delete();
}
/**
* Asserts that the given jar file exists and contains resources as
* listed by the names parameter.
*
* @param jar
* @param names
*/
private void verifyContents(File jar, String... names) {
VerifyArchive verifyArchive = new VerifyArchive(jar);
verifyArchive.initIncludedResources(names);
verifyArchive.execute();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy