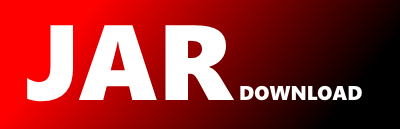
protoj.lang.internal.sample.ServerDemoSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal.sample;
import java.io.File;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.Java;
import org.apache.tools.ant.taskdefs.condition.Os;
import org.junit.Assert;
import protoj.lang.ProjectLayout;
import protoj.lang.ScriptSession;
import protoj.lang.StandardProject;
import protoj.lang.internal.ProtoProject;
import protoj.util.AntTarget;
import protoj.util.ArgRunnable;
import protoj.util.CommandTask;
/**
* Executes a selection of commands against the serverDemoProject and performs
* various asserts against their results.
*
* @author Ashley Williams
*
*/
public final class ServerDemoSession {
private static final String ARCHIVE = "archive";
private static final String STOP = "stop";
private static final String START = "start";
private static final String DIST = "dist";
private static final String COMPILE = "compile";
private static final String RETRIEVE = "retrieve";
private static final String CONFIG = "config";
private static final String EXPAND = "expand";
private StandardProject serverDemoProject;
private ProtoProject protoProject;
private StandardProject distProject;
/**
* After this constructor call the physical serverdemo serverDemoProject
* will have been created.
*/
public ServerDemoSession() {
this.protoProject = new ProtoProject();
this.serverDemoProject = protoProject.createServerDemoProjectDelegate();
}
/**
* The sample project gets executed right up until the dist command is
* called on it which is responsible for creating a copy in the form of an
* executable jar. That jar is executed and then we continue executing
* commands against that new project.
*/
public void execute() {
executeSampleProject();
distProject = createDistProject();
executeDistProject();
}
/**
* The distributed copy of the sample project just needs configuring and
* then it is ready for starting and stopping jboss.
*/
private void executeDistProject() {
final ScriptSession session = distProject.createScriptSession();
ArgRunnable listener = new ArgRunnable() {
public void run(ScriptSession arg) {
CommandTask exec = session.getCurrentExec();
String log = distProject.getLayout().loadLog();
Assert.assertTrue(log, exec.isSuccess());
}
};
session.addCommand("\"dirconf -name profile -interpolate\"", listener,
CONFIG);
session.addCommand("start", listener, START);
session.addCommand("stop", listener, STOP);
// TODO WORKAROUND FOR WINDOW: don't run jboss else serverdemo vm will
// block until it has finished, which is never
if (Os.isFamily(Os.FAMILY_WINDOWS)) {
System.out.println("check the start and stop command manually");
} else {
session.execute();
}
}
/**
* The sample project will act as an installer that extracts the jboss zip
* and configures it appropriately before creating the executable jar file.
*/
private void executeSampleProject() {
final ScriptSession session = serverDemoProject.createScriptSession();
ArgRunnable listener = new ArgRunnable() {
public void run(ScriptSession arg) {
CommandTask exec = session.getCurrentExec();
String log = serverDemoProject.getLayout().loadLog();
Assert.assertTrue(log, exec.isSuccess());
}
};
session.addCommand("\"dirconf -name profile -interpolate\"", listener,
CONFIG);
session.addCommand("retrieve", listener, RETRIEVE);
session.addCommand("compile", listener, COMPILE);
session.addCommand("expand", listener, EXPAND);
session.addCommand("\"dirconf -name profile -interpolate\"", listener,
CONFIG);
session.addCommand("archive", listener, ARCHIVE);
session.addCommand("dist", listener, DIST);
session.execute();
}
/**
* Executes the distribution created by the serverdemo project in order to
* create another copy of it at another location, under the sample project
* target directory.
*
* @return
*/
private StandardProject createDistProject() {
AntTarget target = new AntTarget("serverdemo");
ProjectLayout sampleLayout = serverDemoProject.getLayout();
target.initLogging(Project.MSG_INFO);
Java java = new Java();
target.addTask(java);
java.setTaskName("serverdemo-java");
java.setJar(new File(sampleLayout.getArchiveDir(),
"serverdemo-dist.jar"));
java.setFork(true);
java.setFailonerror(true);
java.setDir(sampleLayout.getTargetDir());
target.execute();
File rootDir = new File(sampleLayout.getTargetDir(), "serverdemo");
StandardProject distProject = new StandardProject(rootDir,
"serverdemo", null);
distProject.getLayout().relaxPermissions();
return distProject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy