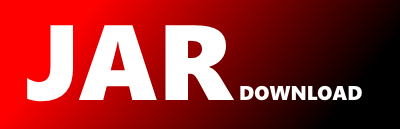
protoj.lang.internal.sample.snippet.UseCaseUploadMavenRepo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
package protoj.lang.internal.sample.snippet;
import protoj.lang.ArchiveFeature;
import protoj.lang.ClassesArchive;
import protoj.lang.JavadocArchive;
import protoj.lang.SourceArchive;
import protoj.lang.StandardProject;
import protoj.lang.command.PublishCommand;
/**
* A code fragment used to demonstrate uploading artifacts to a repository.
*
* @author Ashley Williams
*
*/
public final class UseCaseUploadMavenRepo {
/**
* Adds configuration to the specified project for creating the classes,
* source and javadocs acme archives and uploading them to a maven
* repository.
*
* @param project
*/
public void addConfig(StandardProject project) {
// 1. We want to call methods from this class from inside our pom file.
//
// Any text file in the resources directory can contain velocity markup
// so that hard-coded values such as version numbers can be avoided.
// For example the embedded ${snippet.version} text would get replaced
// with the value of UseCaseUploadMavenRepo.getVersion(). By default the
// StandardProject bean is always available without
// calling this method and is called "project".
project.getResourceFeature().getContext().put("snippet", this);
// 2. We need to inform ProtoJ that we want to publish our artifacts.
//
// The publish feature just needs to know the url of the repository and
// the protocol to use. Additionally we will be using the publish
// command at the command line which will delegate to gpg. In turn gpg
// will prompt for the keyfile password.
String url = "scp://my.projects.net:/path/to/mavenrepo";
project.initPublish(url);
project.getPublishFeature().initProvider("wagon-ssh", "1.0-beta-2");
PublishCommand command = project.getCommands().getPublish();
command.getDelegate().setMemory("32m");
// 3. Next we get on with the business of setting up our archives.
//
// As well as the standard classes archives we would would also like to
// create the javadoc and source code archives. We will be using the out
// of the box archive command at the command line to create the
// archives.
ArchiveFeature archive = project.getArchiveFeature();
ClassesArchive classes = archive.getClassesArchive();
classes.addEntry("acme", null, null, null);
SourceArchive source = archive.getSourceArchive();
source.addEntry("acme", null, null, null);
JavadocArchive javadoc = archive.getJavadocArchive();
javadoc.addEntry("acme", null, null, null, "16m");
// 4. Finally we need to tell the classes archive it is to be published.
//
// The archive feature just needs to know the maven classpath resource
// file that contains the artifact details as well as the gnu
// privacy guard command for signing the artifacts. You can use whatever
// text you like as long as you leave the placeholders for the artifact
// path and signature path. The source code and javadoc archives will
// automatically attach themselves to the upload because they share the
// "acme" name.
String pomName = "/org/acme/acme-publish.xml";
String gpgOptions = "--armor --local-user bob --output %2$s --detach-sign %1$s";
classes.initPublish("acme", pomName, gpgOptions);
}
/**
* The maven file references this property to avoid hard-coding the project
* group id.
*
* @return
*/
public String getGroupId() {
return "org.acme";
}
/**
* The maven file references this property to avoid hard-coding the project
* version number.
*
* @return
*/
public String getVersion() {
return "1.0";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy