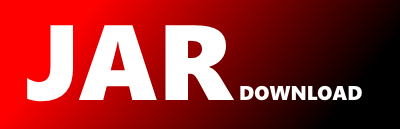
protoj.util.CommandTask Maven / Gradle / Ivy
Show all versions of protoj-jdk6 Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.util;
import java.io.File;
import org.apache.tools.ant.taskdefs.ExecTask;
import org.apache.tools.ant.types.Commandline.Argument;
/**
* A convenience class for executing a system command. Use the constructors to
* specify the minimal and most widely anticipated configuration and the
* initXXX
methods for the less common configuration options.
*
* As far as possible this class is used to hide the differences in syntax
* between different operating systems.
*
* @author Ashley Williams
*
*/
public final class CommandTask {
private String resultProperty = "protoj.result";
private String stdoutProperty = "protoj.stdout";
private String stderrProperty = "protoj.stderr";
private AntTarget target;
private ExecTask execTask;
/**
* Accepts the details of the system command that should be executed. This
* constructor also takes care of ensuring that on windows, the executable
* is in fact cmd.exe
.
*
* If the executable argument ends with a '.' character then this task will
* assume it is a shell script rather than an executable and will add the
* correct bat or sh extension depending on the current operating system.
*
* A special startArgs parameter is provided so that additional windows
* "start" command arguments may be provided. This is a special dos command
* that starts the remainder of the command in a new dos window. This does
* not effect the unix string at all.
*
* @param dir
* the directory in which the command should be executed
* @param executable
* the command to execute without any command line arguments, for
* example "ls" or "run.sh" or "run."
* @param argLine
* a space-delimited list of command-line arguments.
* @param startArgs
* any arguments to the windows "start" command or null if the
* start command isn't required. Specify empty quotes if a new
* dos windows is required but no options are needed.
*/
public CommandTask(File dir, String executable, String argLine,
String startArgs) {
this.target = new AntTarget("protoj-execTask");
execTask = new ExecTask();
target.addTask(execTask);
execTask.setDir(dir);
Argument arg = execTask.createArg();
if (isWindows()) {
String winExecutable = getExecutable(executable, "bat");
// append the correct dos start command and args
String startCommand;
if (startArgs == null) {
startCommand = "";
} else if (startArgs.length() == 0) {
startCommand = "start";
} else {
startCommand = "start " + startArgs;
}
String line = String.format("/c %s %s %s", startCommand,
winExecutable, argLine);
arg.setLine(line);
execTask.setExecutable("cmd.exe");
} else {
String nixExecutable = getExecutable(executable, "sh");
arg.setLine(argLine);
execTask.setExecutable(nixExecutable);
}
}
/**
* Enables logging to the specified log file at Project.MSG_INFO level.
*/
public void initLogging(int level) {
target.initLogging(level);
}
/**
* If the executable ends with a "." then the extension is appended and
* returned, else it is returned unchanged.
*
* @param executable
* @param extension
* @return
*/
private String getExecutable(String executable, String extension) {
String winExe;
if (executable.endsWith(".")) {
winExe = executable + extension;
} else {
winExe = executable;
}
return winExe;
}
/**
* Specify true so that the process will outlive this java process, false
* otherwise.
*
* @param spawn
*/
public void initSpawn(boolean spawn) {
execTask.setSpawn(spawn);
if (!spawn) {
execTask.setOutputproperty(stdoutProperty);
execTask.setErrorProperty(stderrProperty);
execTask.setResultProperty(resultProperty);
}
}
/**
* Helper for {@link #CommandTask} for determining if the current OS is
* windows.
*
* @return
*/
public boolean isWindows() {
String osName = System.getProperty("os.name").toUpperCase();
return osName.indexOf("WINDOWS") >= 0;
}
/**
* The underlying ant implementation object.
*
* @return
*/
public ExecTask getExecTask() {
return execTask;
}
public void execute() {
target.execute();
}
public String getStdout() {
return target.getProject().getProperty(stdoutProperty);
}
public String getStderr() {
return target.getProject().getProperty(stderrProperty);
}
public String getResult() {
return target.getProject().getProperty(resultProperty);
}
/**
* All the output from the executed command seems to get buffered.command
* has exited, the buffered output can be written using this method.
*/
public void writeOutput() {
String systemOut = getStdout();
if (systemOut != null && systemOut.length() > 0) {
System.out.println(systemOut);
}
String systemErr = getStderr();
if (systemErr != null && systemErr.length() > 0) {
System.err.println(systemErr);
}
}
/**
* It only makes sense to call this method from a forked but non-spawned vm.
* Remember that a spawned vm will outlive the calling process.
*
* @return
*/
public boolean isSuccess() {
return getResult().equals("0");
}
}