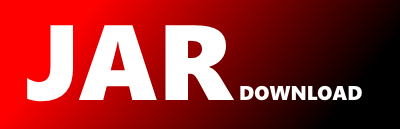
protoj.util.JavaTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.util;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.apache.tools.ant.taskdefs.Java;
import org.apache.tools.ant.types.Path;
import org.apache.tools.ant.types.Commandline.Argument;
/**
* A convenience class for creating a command that will start a virtual machine.
* Use the constructors to specify the minimal and most widely anticipated
* configuration and the initXXX
methods for the less common
* configuration options.
*
* @author Ashley Williams
*
*/
public final class JavaTask {
private String resultProperty = "protoj.result";
private String stdoutProperty = "protoj.stdout";
private String stderrProperty = "protoj.stderr";
private AntTarget target;
private Java javaTask;
private String mainClass;
private boolean fork = false;
private String maxMemory = "0";
private boolean spawn = false;
private List args = new ArrayList();
private List jvmargs = new ArrayList();
private String debugSuspend;
private int debugPort;
public JavaTask() {
target = new AntTarget("protoj-start-vm");
javaTask = new Java();
javaTask.setTaskName("javaTask");
target.addTask(javaTask);
}
/**
* Sets the main class on the underlying ant task.
*
* @param mainClass
*/
public void setMainClass(String mainClass) {
this.mainClass = mainClass;
this.javaTask.setClassname(mainClass);
}
/**
* Enables logging at Project.MSG_INFO level.
*/
public void initLogging(int level) {
target.initLogging(level);
}
/**
* Invoke this method if the virtual machine should fork.
*
* @param maxMemory
* specify the maximum memory in the format used by the
* javaTask
tool
* @param spawn
* whether or not the process should be spawned i.e. outlive the
* start-vm process
*/
public void initFork(String maxMemory, boolean spawn) {
this.fork = true;
this.maxMemory = maxMemory;
this.spawn = spawn;
javaTask.setFork(true);
javaTask.setMaxmemory(maxMemory);
javaTask.setSpawn(spawn);
if (!spawn) {
javaTask.setOutputproperty(stdoutProperty);
javaTask.setErrorProperty(stderrProperty);
javaTask.setResultProperty(resultProperty);
}
}
/**
* Invoke if any arguments to main are required.
*
* @param args
*/
public void initArgs(String... args) {
initArgs(Arrays.asList(args));
}
/**
* Invoke if any arguments to main are required.
*
* @param args
*/
public void initArgs(List args) {
this.args.addAll(args);
for (String value : args) {
Argument arg = javaTask.createArg();
arg.setValue(value);
}
}
/**
* Invoke if any virtual machine arguments are required.
*
* @param jvmargs
*/
public void initJvmargs(String... jvmargs) {
initJvmargs(Arrays.asList(jvmargs));
}
/**
* Invoke if any virtual machine arguments are required.
*
* @param jvmargs
*/
public void initJvmargs(List jvmargs) {
this.jvmargs.addAll(jvmargs);
for (String value : jvmargs) {
Argument jvmarg = javaTask.createJvmarg();
jvmarg.setValue(value);
}
}
/**
* Invoke if the classpath should be configured. Because invoking methods
* don't have the ability to create the concrete Path
instance,
* they instead supply a ClasspathConfig
instance containing
* instructions on how to configure the path.
*
* @param config
*/
public void initClasspath(ArgRunnable config) {
Path classpath = getJavaTask().createClasspath();
config.run(classpath);
}
/**
* Invoke if debugging is required.
*
* @param port
* the port that will accept a debugger connection
* @param suspend
* whether or not the virtual machine will suspend until a
* debugger connection is made
*/
public void initDebug(int port, boolean suspend) {
debugSuspend = suspend ? "y" : "n";
debugPort = port;
Argument jvmarg = javaTask.createJvmarg();
jvmarg.setLine("-Xdebug -Xrunjdwp:transport=dt_socket,address=" + port
+ ",server=y,suspend=" + debugSuspend);
}
/**
* Invoke if loadtime weaving is required.
*
* @param weaverJar
* the location of the aspectj weaver library
*/
public void initWeaving(File weaverJar) {
Argument jvmarg = javaTask.createJvmarg();
jvmarg.setValue("-javaagent:" + weaverJar.getAbsolutePath());
}
/**
* Invoke if local jmx connectivity is required.
*/
public void initLocalJmx() {
Argument jvmarg = javaTask.createJvmarg();
jvmarg.setValue("-Dcom.sun.management.jmxremote");
}
/**
* Invoke if remote jmx connectivity is required.
*
* @param port
* the port that the jmx agent will be listening for connections
* on
*/
public void initRemoteJmx(Integer port) {
Argument jvmarg = javaTask.createJvmarg();
jvmarg.setValue("-Dcom.sun.management.jmxremote.port=" + port);
}
public String getMainClass() {
return mainClass;
}
public String getStdout() {
return target.getProject().getProperty(stdoutProperty);
}
public String getStderr() {
return target.getProject().getProperty(stderrProperty);
}
public String getResult() {
return target.getProject().getProperty(resultProperty);
}
public Java getJavaTask() {
return javaTask;
}
public String getDebugSuspend() {
return debugSuspend;
}
public int getDebugPort() {
return debugPort;
}
public List getArgs() {
return args;
}
public List getJvmargs() {
return jvmargs;
}
public void execute() {
target.execute();
}
/**
* When forking a vm, all the output from the forked vm seems to get
* buffered. Not sure if this is down to javaTask or to the ant Java task.
* Either way when the forked vm has exited, the buffered output can be
* written using this method.
*/
public void writeOutput() {
String systemOut = getStdout();
if (systemOut != null && systemOut.length() > 0) {
System.out.println(systemOut);
}
String systemErr = getStderr();
if (systemErr != null && systemErr.length() > 0) {
System.err.println(systemErr);
}
}
/**
* It only makes sense to call this method from a forked but non-spawned vm.
* Remember that a spawned vm will outlive the calling process.
*
* @return
*/
public boolean isSuccess() {
return getResult().equals("0");
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("mainclass=");
builder.append(mainClass);
builder.append(" args={");
for (String arg : args) {
builder.append(arg);
builder.append(", ");
}
builder.append("} jvmargs={");
for (String jvmarg : jvmargs) {
builder.append(jvmarg);
builder.append(", ");
}
builder.append("} fork=");
builder.append(fork);
builder.append(" spawn=");
builder.append(spawn);
builder.append(" maxMemory=");
builder.append(maxMemory);
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy