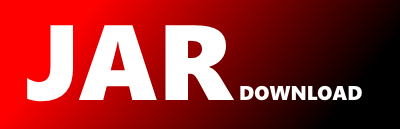
protoj.util.JunitTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-jdk6 Show documentation
Show all versions of protoj-jdk6 Show documentation
ProtoJ: the pure java build library with maximum dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.util;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.optional.junit.BatchTest;
import org.apache.tools.ant.taskdefs.optional.junit.FormatterElement;
import org.apache.tools.ant.taskdefs.optional.junit.JUnitTask;
import org.apache.tools.ant.taskdefs.optional.junit.FormatterElement.TypeAttribute;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.Path;
import org.apache.tools.ant.types.Commandline.Argument;
/**
* A convenience class for running junit tests. Use the constructors to specify
* the minimal and most widely anticipated configuration and the
* initXXX
methods for the less common configuration options.
*
* @author Ashley Williams
*
*/
public final class JunitTask {
public static final String ERROR = "error";
private AntTarget target;
private JUnitTask junit;
private List jvmargs = new ArrayList();
private String debugSuspend;
private int debugPort;
/**
* Accepts the information required for running junit tests.
*
* @param junitReportsDir
* the directory where the reports should be created
* @param javaDir
* the directory to search for junit tests
* @param classpathConfig
* @param maxMemory
* the maximum memory to be given to junit
* @param includes
* which tests to include, can be null
* @param excludes
* which tests to exclude, can be null
*/
public JunitTask(File junitReportsDir, File javaDir,
ArgRunnable classpathConfig, String maxMemory,
String includes, String excludes) {
target = new AntTarget("protoj-junit");
junitReportsDir.mkdirs();
try {
junit = new JUnitTask();
} catch (Exception e) {
throw new RuntimeException(e);
}
target.addTask(junit);
junit.setTaskName("junit-test");
junit.setMaxmemory(maxMemory);
junit.setHaltonerror(true);
FormatterElement fe = new FormatterElement();
TypeAttribute type = new TypeAttribute();
type.setValue("plain");
fe.setType(type);
fe.setUseFile(true);
junit.addFormatter(fe);
Path classpath = junit.createClasspath();
classpathConfig.run(classpath);
BatchTest batchTest = junit.createBatchTest();
batchTest.setFork(true);
batchTest.setTodir(junitReportsDir);
FileSet testFilter = new FileSet();
testFilter.setDir(javaDir);
if (includes != null) {
testFilter.setIncludes(includes);
}
if (excludes != null) {
testFilter.setExcludes(excludes);
}
batchTest.addFileSet(testFilter);
}
/**
* Invoke if debugging is required.
*
* @param port
* the port that will accept a debugger connection
* @param suspend
* whether or not the virtual machine will suspend until a
* debugger connection is made
*/
public void initDebug(int port, boolean suspend) {
debugSuspend = suspend ? "y" : "n";
debugPort = port;
Argument jvmarg = junit.createJvmarg();
jvmarg.setLine("-Xdebug -Xrunjdwp:transport=dt_socket,address="
+ debugPort + ",server=y,suspend=" + debugSuspend);
}
/**
* Invoke if any virtual machine arguments are required.
*
* @param jvmargs
*/
public void initJvmargs(String... jvmargs) {
initJvmargs(Arrays.asList(jvmargs));
}
/**
* Invoke if any virtual machine arguments are required.
*
* @param jvmargs
*/
public void initJvmargs(List jvmargs) {
this.jvmargs.addAll(jvmargs);
for (String value : jvmargs) {
Argument jvmarg = junit.createJvmarg();
jvmarg.setValue(value);
}
}
public void captureError() {
junit.setErrorProperty(ERROR);
}
public String getError() {
return target.getProject().getProperty(ERROR);
}
public void initLogging() {
target.initLogging(Project.MSG_INFO);
}
public void execute() {
target.execute();
}
public JUnitTask getJunit() {
return junit;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy