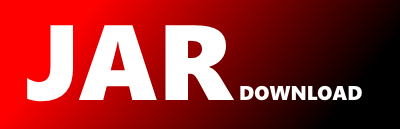
protoj.lang.internal.ProtoProject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoj-nodep Show documentation
Show all versions of protoj-nodep Show documentation
ProtoJ: the pure java build library with no dependencies
The newest version!
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.lang.internal;
import java.io.File;
import org.apache.commons.io.FileUtils;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.Java;
import protoj.lang.ArchiveFeature;
import protoj.lang.ClassesArchive;
import protoj.lang.JavadocArchive;
import protoj.lang.ProjectLayout;
import protoj.lang.ResourceFeature;
import protoj.lang.SourceArchive;
import protoj.lang.StandardProject;
import protoj.lang.ClassesArchive.ClassesEntry;
import protoj.lang.command.ArchiveCommand;
import protoj.lang.command.PublishCommand;
import protoj.lang.command.ReleaseCommand;
import protoj.lang.command.SiteCommand;
import protoj.util.AntTarget;
import protoj.util.ArgRunnable;
/**
* Represents the use-cases available to be carried out against the protoj
* delegate.
*
* @author Ashley Williams
*
*/
public final class ProtoProject {
/**
* See {@link ProtoProject}.
*
* @param args
*/
public static void main(String[] args) {
new ProtoProject(args).dispatchCommands();
}
/**
* See {@link #getDelegate()}.
*/
private StandardProject delegate;
/**
* See {@link #getReleaseCommand()}.
*/
private ReleaseCommand releaseCommand;
/**
* See {@link #getReleaseFeature()}.
*/
private ReleaseFeature releaseFeature;
/**
* Responsible for extracting the google wiki pages.
*/
private SiteCommand siteCommand;
/**
* Constructor that uses system properties protoj.rootDir and
* protoj.scriptName.
*/
public ProtoProject() {
init(new StandardProject(createVersionInfo()));
}
/**
* See the
* StandardProject.StandardProject(String[], String, String)
* constructor for a discussion of the arguments to main().
*
* @param args
*/
public ProtoProject(String[] args) {
init(new StandardProject(args, createVersionInfo()));
}
/**
* Create with the underlying project delegate.
*
* @param project
*/
public void init(StandardProject project) {
// objects that much functionality will be delegated to
delegate = project;
releaseFeature = new ReleaseFeature(this);
// change the name of the profile from default using property override
project.initDirConfig(getProjectHome());
project.getResourceFeature().getContext().put("protoProject", this);
project.getRetrieveFeature().initIvy("/protoj/ivy.xml");
initNoDependenciesArchive();
initDependenciesArchive();
// the tar feature is supported and will include the example directory
delegate.initJunit("32m");
delegate.initUploadGoogleCode("protoj");
delegate
.initPublish("scp://shell.sourceforge.net:/home/groups/p/pr/protojrepo/htdocs/mavensync");
delegate.getPublishFeature().initProvider("wagon-ssh", "1.0-beta-2");
PublishCommand publishCommand = delegate.getCommands().getPublish();
publishCommand.getDelegate().setMemory("32m");
ArchiveCommand archiveCommand = delegate.getCommands().getArchive();
archiveCommand.getDelegate().setMemory("32m");
releaseCommand = new ReleaseCommand(this, this.getClass().getName());
siteCommand = new SiteCommand(this);
}
/**
* Configures the artifacts for the no-dependencies archive.
*/
private void initNoDependenciesArchive() {
final String projectVersion = getVersion();
// the no-dependencies jar file merged with all jars in the lib dir
ClassesArchive classes = delegate.getArchiveFeature()
.getClassesArchive();
String jarName = getNoDepJarTag();
classes.addEntry(jarName, "MANIFEST", null, null);
classes.getEntry(jarName).initConfig(new ArgRunnable() {
public void run(ClassesArchive archive) {
archive.getCurrentAssembleTask().initManifest("ProtoJ-Version",
projectVersion);
archive.initExecutableJar(ProtoExecutableMain.class.getName());
}
});
classes.initExcludeArchives(jarName, "aspectjtools.jar",
"aspectjweaver.jar");
String pomName = "/protoj/" + jarName + "-publish.xml";
classes.initPublish(jarName, pomName, getGpgOptions());
// creates a source code jar file
SourceArchive source = delegate.getArchiveFeature().getSourceArchive();
source.addEntry(jarName, null, null, null);
source.getEntry(jarName).initConfig(new ArgRunnable() {
public void run(SourceArchive archive) {
archive.getCurrentAssembleTask().initManifest("ProtoJ-Version",
projectVersion);
}
});
// creates a javadoc jar file
JavadocArchive archive = delegate.getArchiveFeature()
.getJavadocArchive();
archive.addEntry(jarName, null, null, null, "16m");
archive.getEntry(jarName).initConfig(new ArgRunnable() {
public void run(JavadocArchive archive) {
archive.getCurrentAssembleTask().initManifest("ProtoJ-Version",
projectVersion);
}
});
}
/**
* Configures the artifacts for the dependencies archive.
*/
private void initDependenciesArchive() {
final String projectVersion = getVersion();
// merge with just those lib jars that can't be found at
// the maven central repository or with recent updates
ClassesArchive classes = delegate.getArchiveFeature()
.getClassesArchive();
String jarName = getJarTag();
classes.addEntry(jarName, "MANIFEST", null, null);
classes.getEntry(jarName).initConfig(new ArgRunnable() {
public void run(ClassesArchive archive) {
archive.getCurrentAssembleTask().initManifest("ProtoJ-Version",
projectVersion);
archive.initExecutableJar(ProtoExecutableMain.class.getName());
}
});
classes.initIncludeArchives(jarName, "ant-googlecode-0.0.1.jar",
"jsch-0.1.41.jar");
String pomName = "/protoj/" + jarName + "-publish.xml";
classes.initPublish(jarName, pomName, getGpgOptions());
// creates a source code jar file
SourceArchive source = delegate.getArchiveFeature().getSourceArchive();
source.addEntry(jarName, null, null, null);
source.getEntry(jarName).initConfig(new ArgRunnable() {
public void run(SourceArchive archive) {
archive.getCurrentAssembleTask().initManifest("ProtoJ-Version",
projectVersion);
}
});
// creates a javadoc jar file
JavadocArchive archive = delegate.getArchiveFeature()
.getJavadocArchive();
archive.addEntry(jarName, null, null, null, "16m");
archive.getEntry(jarName).initConfig(new ArgRunnable() {
public void run(JavadocArchive archive) {
archive.getCurrentAssembleTask().initManifest("ProtoJ-Version",
projectVersion);
}
});
}
private String getGpgOptions() {
return "--armor --local-user agwilliams1000 --output %2$s --detach-sign %1$s";
}
/**
* The downloaded jboss 5 and 6 zips are too big to include in the protoj
* jar file and so they are kept just under the source directory and copied
* when the serverdemo project is being created here.
*
* @return
*/
public StandardProject createServerDemoProjectDelegate() {
StandardProject demoDelegate = createSampleProject("serverdemo", true);
File thisSrcDir = delegate.getLayout().getSrcDir();
File demoSrcDir = demoDelegate.getLayout().getSrcDir();
File jboss5 = new File(thisSrcDir, "jboss-5.0.1.GA.zip");
FileUtils.copyFileToDirectory(jboss5, demoSrcDir);
File jboss6 = new File(thisSrcDir, "jboss-5.0.1.GA-jdk6.zip");
FileUtils.copyFileToDirectory(jboss6, demoSrcDir);
return demoDelegate;
}
/**
* Ensures the no dependencies jar file is present and executes it so that
* the requested sample project gets created. See
* {@link ProtoExecutableMain} for a list of the available sample projects.
*
* @param projectName
* the name of the sample project to create
* @param includeAjc
* for aspectj sample projects specify true to include the
* aspectj jars in the lib directory
*
* @return
*/
public StandardProject createSampleProject(String projectName,
boolean includeAjc) {
createNoDepArchive();
AntTarget target = new AntTarget("sample");
ProjectLayout layout = delegate.getLayout();
target.initLogging(Project.MSG_INFO);
Java java = new Java();
target.addTask(java);
java.setTaskName("sample-java");
java.setJar(getNoDepFile());
java.createArg().setValue("-sample");
java.createArg().setValue(projectName);
java.setFork(true);
java.setFailonerror(true);
java.setDir(layout.getTargetDir());
target.execute();
File rootDir = new File(layout.getTargetDir(), projectName);
StandardProject sampleProject = new StandardProject(rootDir,
projectName, null);
File protoLibDir = delegate.getLayout().getLibDir();
File sampleLibDir = sampleProject.getLayout().getLibDir();
if (includeAjc) {
File aspectjtools = new File(protoLibDir, "aspectjtools.jar");
FileUtils.copyFileToDirectory(aspectjtools, sampleLibDir);
File aspectjweaver = new File(protoLibDir, "aspectjweaver.jar");
FileUtils.copyFileToDirectory(aspectjweaver, sampleLibDir);
File aspectjrt = new File(protoLibDir, "aspectjrt.jar");
FileUtils.copyFileToDirectory(aspectjrt, sampleLibDir);
}
return sampleProject;
}
/**
* Dispatches any commands held by this project.
*/
public void dispatchCommands() {
delegate.getDispatchFeature().dispatchCommands();
}
/**
* The command used to invoke release functionality.
*
* @return
*/
public ReleaseCommand getReleaseCommand() {
return releaseCommand;
}
/**
* The command used to create documentation for the protoj googlecode
* website.
*
* @return
*/
public SiteCommand getSiteCommand() {
return siteCommand;
}
/**
* Delegate helper responsible for implementing the release procedure.
*
* @return
*/
public ReleaseFeature getReleaseFeature() {
return releaseFeature;
}
/**
* The main protoj domain object.
*
* @return
*/
public StandardProject getDelegate() {
return delegate;
}
/**
* The name of the project that includes the version number.
*
* @return
*/
public String getProjectName() {
return "protoj-" + getVersion();
}
/**
* The name of the protoj jar file that has been merged with all third party
* jar files. This doesn't include the version number or the extension.
*
* @return
*/
public String getNoDepJarTag() {
return "protoj-nodep";
}
/**
* The complete name of the no dependencies jar file.
*
* @return
*/
public String getNoDepJarName() {
return getNoDepJarTag() + "-" + getVersion() + ".jar";
}
/**
* The name of the protoj jar file that contains just a few third-party jar
* merges. They are restricted to those libraries that can't be found at the
* maven central repository. This doesn't include the version number or the
* extension.
*
* @return
*/
public String getJarTag() {
return "protoj";
}
/**
* The complete name of the maximum dependencies jar file.
*
* @return
*/
public String getJarName() {
return getJarTag() + "-" + getVersion() + ".jar";
}
/**
* The name of the generated tar file.
*
* @return
*/
public String getProjectArchiveName() {
return "protoj";
}
/**
* The group id to use when publish to the maven repository.
*
* @return
*/
public String getGroupId() {
return "com.google.code.protoj";
}
/**
* Nice to factor this into a method of its own.
*
* @return
*/
private String createVersionInfo() {
return "ProtoJ version " + getVersion();
}
/**
* The project version number.
*
* @return
*/
public String getVersion() {
return "1.9.0";
}
/**
* Creates the no dependencies archive in the archive directory. Used by the
* tests that need to create test projects.
*/
public void createNoDepArchive() {
ArchiveFeature feature = getDelegate().getArchiveFeature();
ClassesArchive classes = feature.getClassesArchive();
classes.createArchive(getNoDepJarTag());
}
public File getNoDepFile() {
ArchiveFeature feature = getDelegate().getArchiveFeature();
ClassesArchive classes = feature.getClassesArchive();
ClassesEntry entry = classes.getEntry(getNoDepJarTag());
return entry.getArchiveEntry().getArtifact();
}
/**
* Extracts the googlecode website files to the target directory.
*
* @return the directory where the files are extracted to
*/
public File extractSite() {
ResourceFeature feature = getDelegate().getResourceFeature();
File targetDir = getDelegate().getLayout().getTargetDir();
File siteDir = new File(targetDir, "google-site");
feature.filterResourceToDir("/protoj/site/project-summary.wiki",
siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/AlternativeProjects.wiki", siteDir);
feature.filterResourceToDir("/protoj/site/wiki/BasicConcepts.wiki",
siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/BuildingFromSource.wiki", siteDir);
feature.filterResourceToDir("/protoj/site/wiki/CommandSetup.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/Sidebar.wiki", siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseCompile.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseProfile.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseDebug.wiki",
siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/UseCaseDependencies.wiki", siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseDeploy.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseHelp.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseLog.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCasePackage.wiki",
siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/UseCaseUploadMavenRepo.wiki", siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/UseCaseUploadGoogleCode.wiki", siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseProfile.wiki",
siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/UseCasePackageRelationships.wiki", siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/UseCaseSpecifyProperties.wiki", siteDir);
feature.filterResourceToDir("/protoj/site/wiki/UseCaseTest.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/DemoJbossProject.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/DemoAlienProject.wiki",
siteDir);
feature.filterResourceToDir("/protoj/site/wiki/DemoBasicProject.wiki",
siteDir);
feature.filterResourceToDir(
"/protoj/site/wiki/DemoHelloWorldProject.wiki", siteDir);
return siteDir;
}
public File getProjectHome() {
String userHome = System.getProperty("user.home");
return new File(userHome, ".protoj");
}
/**
* ProtoJ artifacts are deployed to the maven central repository, this is
* the url.
*
* @return
*/
public String getCentralRepositoryUrl() {
return "http://repo1.maven.org/maven2/com/google/code/protoj/";
}
/**
* The browsable subversion url for the project. This is up to an including
* the root of just this release.
*
* @return
*/
public String getBrowsableUrl() {
return "http://code.google.com/p/protoj/source/browse/" + getVersion();
}
/**
* The googlecode download url for the project artifacts.
*
* @return
*/
public String getDownloadUr() {
return "http://protoj.googlecode.com/files";
}
/**
* The url for downloading the no dependencies jar from googlecode.
*
* @return
*/
public String getNoDepDownloadUrl() {
return getDownloadUr() + "/" + getNoDepJarName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy