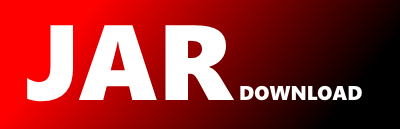
protoj.util.TarTask Maven / Gradle / Ivy
Show all versions of protoj-nodep Show documentation
/**
* Copyright 2009 Ashley Williams
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package protoj.util;
import java.io.File;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.Tar;
import org.apache.tools.ant.types.TarFileSet;
/**
* A convenience class for creating compressed tar files. Use the constructors
* to specify the minimal and most widely anticipated configuration and the
* initXXX
methods for the less common configuration options.
*
* Tar files are created in gnu format which means arbitrary path lengths are
* supported. Therefore they must be untarred with the gtar tool.
*
* @author Ashley Williams
*
*/
public final class TarTask {
private AntTarget target = new AntTarget("protoj-tar");
private Tar tar;
private File destFile;
private String tarName;
/**
* Creates a command that can generate tar files of the given name in the
* given directory. The generated file is compressed and given an extension
* of tar.gz. So the fully qualified path to the file is therefore
* destDir/tarName.tar.gz
*
* @param destDir
* where the tar file is to be created
* @param tarName
* the name of the tar file without the extension
*/
public TarTask(String destDir, String tarName) {
new File(destDir).mkdirs();
this.tarName = tarName;
tar = new Tar();
tar.setTaskName("tar");
target.addTask(tar);
destFile = new File(destDir, tarName + ".tar.gz");
tar.setDestFile(destFile);
Tar.TarLongFileMode mode = new Tar.TarLongFileMode();
mode.setValue("gnu");
tar.setLongfile(mode);
Tar.TarCompressionMethod method = new Tar.TarCompressionMethod();
method.setValue("gzip");
tar.setCompression(method);
}
/**
* Adds content to the tarfile with the specified properties. Consult the
* ant documentation for the meaning of the parameters.
*
* @param dir
* @param prefix
* @param fileMode
* @param dirMode
* @param userName
* @param group
* @param includes
* @param excludes
*/
public void addFileSet(File dir, String prefix, String fileMode,
String dirMode, String userName, String group, String includes,
String excludes) {
TarFileSet fileSet = new TarFileSet();
fileSet.setProject(tar.getProject());
fileSet.setDir(dir);
if (prefix != null) {
fileSet.setPrefix(prefix);
}
if (fileMode != null) {
fileSet.setFileMode(fileMode);
}
if (dirMode != null) {
fileSet.setDirMode(dirMode);
}
if (userName != null) {
fileSet.setUserName(userName);
}
if (group != null) {
fileSet.setGroup(group);
}
if (includes != null) {
fileSet.setIncludes(includes);
}
if (excludes != null) {
fileSet.setExcludes(excludes);
}
tar.add(fileSet);
}
/**
* Enables logging to the specified log file at Project.MSG_INFO level.
*/
public void initLogging() {
target.initLogging(Project.MSG_INFO);
}
public void execute() {
target.execute();
}
public String getTarName() {
return tarName;
}
public String getTarFilePath() {
return destFile.getName();
}
public File getDestFile() {
return destFile;
}
public Tar getTar() {
return tar;
}
}