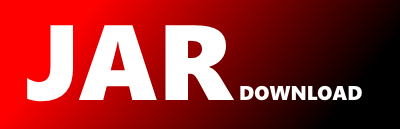
net.spy.memcached.protocol.ascii.DeleteOperationImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spymemcached Show documentation
Show all versions of spymemcached Show documentation
A client library for memcached.
// Copyright (c) 2006 Dustin Sallings
package net.spy.memcached.protocol.ascii;
import java.nio.ByteBuffer;
import java.util.Collection;
import java.util.Collections;
import net.spy.memcached.KeyUtil;
import net.spy.memcached.ops.DeleteOperation;
import net.spy.memcached.ops.OperationCallback;
import net.spy.memcached.ops.OperationState;
import net.spy.memcached.ops.OperationStatus;
/**
* Operation to delete an item from the cache.
*/
final class DeleteOperationImpl extends OperationImpl
implements DeleteOperation {
private static final int OVERHEAD=32;
private static final OperationStatus DELETED=
new OperationStatus(true, "DELETED");
private static final OperationStatus NOT_FOUND=
new OperationStatus(false, "NOT_FOUND");
private final String key;
public DeleteOperationImpl(String k, OperationCallback cb) {
super(cb);
key=k;
}
@Override
public void handleLine(String line) {
getLogger().debug("Delete of %s returned %s", key, line);
getCallback().receivedStatus(matchStatus(line, DELETED, NOT_FOUND));
transitionState(OperationState.COMPLETE);
}
@Override
public void initialize() {
ByteBuffer b=ByteBuffer.allocate(
KeyUtil.getKeyBytes(key).length + OVERHEAD);
setArguments(b, "delete", key);
b.flip();
setBuffer(b);
}
public Collection getKeys() {
return Collections.singleton(key);
}
@Override
public String toString() {
return "Cmd: delete Key: " + key;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy