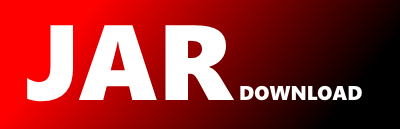
com.xerox.amazonws.typica.autoscale.jaxb.AutoScalingGroup Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.1-b02-fcs
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2010.10.06 at 09:30:17 PM CEST
//
package com.xerox.amazonws.typica.autoscale.jaxb;
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for AutoScalingGroup complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AutoScalingGroup">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AutoScalingGroupName" type="{http://autoscaling.amazonaws.com/doc/2009-05-15/}AutoScalingGroupName"/>
* <element name="LaunchConfigurationName" type="{http://autoscaling.amazonaws.com/doc/2009-05-15/}LaunchConfigurationName"/>
* <element name="MinSize" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="MaxSize" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="DesiredCapacity" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="Cooldown" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="AvailabilityZones" type="{http://autoscaling.amazonaws.com/doc/2009-05-15/}AvailabilityZones"/>
* <element name="LoadBalancerNames" type="{http://autoscaling.amazonaws.com/doc/2009-05-15/}LoadBalancerNames" minOccurs="0"/>
* <element name="Instances" type="{http://autoscaling.amazonaws.com/doc/2009-05-15/}Instances" minOccurs="0"/>
* <element name="CreatedTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AutoScalingGroup", propOrder = {
"autoScalingGroupName",
"launchConfigurationName",
"minSize",
"maxSize",
"desiredCapacity",
"cooldown",
"availabilityZones",
"loadBalancerNames",
"instances",
"createdTime"
})
public class AutoScalingGroup {
@XmlElement(name = "AutoScalingGroupName", required = true)
protected String autoScalingGroupName;
@XmlElement(name = "LaunchConfigurationName", required = true)
protected String launchConfigurationName;
@XmlElement(name = "MinSize", required = true)
protected BigInteger minSize;
@XmlElement(name = "MaxSize", required = true)
protected BigInteger maxSize;
@XmlElement(name = "DesiredCapacity", required = true)
protected BigInteger desiredCapacity;
@XmlElement(name = "Cooldown", required = true)
protected BigInteger cooldown;
@XmlElement(name = "AvailabilityZones", required = true)
protected AvailabilityZones availabilityZones;
@XmlElement(name = "LoadBalancerNames")
protected LoadBalancerNames loadBalancerNames;
@XmlElement(name = "Instances")
protected Instances instances;
@XmlElement(name = "CreatedTime", required = true)
protected XMLGregorianCalendar createdTime;
/**
* Gets the value of the autoScalingGroupName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAutoScalingGroupName() {
return autoScalingGroupName;
}
/**
* Sets the value of the autoScalingGroupName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAutoScalingGroupName(String value) {
this.autoScalingGroupName = value;
}
/**
* Gets the value of the launchConfigurationName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLaunchConfigurationName() {
return launchConfigurationName;
}
/**
* Sets the value of the launchConfigurationName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLaunchConfigurationName(String value) {
this.launchConfigurationName = value;
}
/**
* Gets the value of the minSize property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getMinSize() {
return minSize;
}
/**
* Sets the value of the minSize property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setMinSize(BigInteger value) {
this.minSize = value;
}
/**
* Gets the value of the maxSize property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getMaxSize() {
return maxSize;
}
/**
* Sets the value of the maxSize property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setMaxSize(BigInteger value) {
this.maxSize = value;
}
/**
* Gets the value of the desiredCapacity property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDesiredCapacity() {
return desiredCapacity;
}
/**
* Sets the value of the desiredCapacity property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDesiredCapacity(BigInteger value) {
this.desiredCapacity = value;
}
/**
* Gets the value of the cooldown property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getCooldown() {
return cooldown;
}
/**
* Sets the value of the cooldown property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setCooldown(BigInteger value) {
this.cooldown = value;
}
/**
* Gets the value of the availabilityZones property.
*
* @return
* possible object is
* {@link AvailabilityZones }
*
*/
public AvailabilityZones getAvailabilityZones() {
return availabilityZones;
}
/**
* Sets the value of the availabilityZones property.
*
* @param value
* allowed object is
* {@link AvailabilityZones }
*
*/
public void setAvailabilityZones(AvailabilityZones value) {
this.availabilityZones = value;
}
/**
* Gets the value of the loadBalancerNames property.
*
* @return
* possible object is
* {@link LoadBalancerNames }
*
*/
public LoadBalancerNames getLoadBalancerNames() {
return loadBalancerNames;
}
/**
* Sets the value of the loadBalancerNames property.
*
* @param value
* allowed object is
* {@link LoadBalancerNames }
*
*/
public void setLoadBalancerNames(LoadBalancerNames value) {
this.loadBalancerNames = value;
}
/**
* Gets the value of the instances property.
*
* @return
* possible object is
* {@link Instances }
*
*/
public Instances getInstances() {
return instances;
}
/**
* Sets the value of the instances property.
*
* @param value
* allowed object is
* {@link Instances }
*
*/
public void setInstances(Instances value) {
this.instances = value;
}
/**
* Gets the value of the createdTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getCreatedTime() {
return createdTime;
}
/**
* Sets the value of the createdTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setCreatedTime(XMLGregorianCalendar value) {
this.createdTime = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy