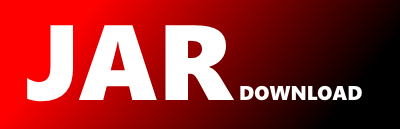
com.google.crypto.tink.hybrid.PredefinedHybridParameters Maven / Gradle / Ivy
Show all versions of tink Show documentation
// Copyright 2023 Google Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
////////////////////////////////////////////////////////////////////////////////
package com.google.crypto.tink.hybrid;
import static com.google.crypto.tink.internal.TinkBugException.exceptionIsBug;
import com.google.crypto.tink.aead.AesCtrHmacAeadParameters;
import com.google.crypto.tink.aead.AesGcmParameters;
/**
* Pre-generated {@link Parameter} objects for {@link com.google.crypto.tink.HybridEncrypt} and
* {@link com.google.crypto.tink.HybridDecrypt} keys.
*
* Note: if you want to keep dependencies small, consider inlining the constants here.
*/
public final class PredefinedHybridParameters {
/**
* A {@link KeyTemplate} that generates new instances of {@link
* com.google.crypto.tink.proto.EciesAeadHkdfPrivateKey} with the following parameters:
*
*
* - KEM: ECDH over NIST P-256
*
- DEM: AES128-GCM
*
- KDF: HKDF-HMAC-SHA256 with an empty salt
*
*
* Unlike other key templates that use AES-GCM, the instances of {@link HybridDecrypt}
* generated by this key template has no limitation on Android KitKat (API level 19). They might
* not work in older versions though.
*/
public static final EciesParameters ECIES_P256_HKDF_HMAC_SHA256_AES128_GCM =
exceptionIsBug(
() ->
EciesParameters.builder()
.setCurveType(EciesParameters.CurveType.NIST_P256)
.setHashType(EciesParameters.HashType.SHA256)
.setNistCurvePointFormat(EciesParameters.PointFormat.UNCOMPRESSED)
.setVariant(EciesParameters.Variant.TINK)
.setDemParameters(
AesGcmParameters.builder()
.setIvSizeBytes(12)
.setKeySizeBytes(16)
.setTagSizeBytes(16)
.setVariant(AesGcmParameters.Variant.NO_PREFIX)
.build())
.build());
/**
* A {@link KeyTemplate} that generates new instances of {@link
* com.google.crypto.tink.proto.EciesAeadHkdfPrivateKey} with the following parameters:
*
*
* - KEM: ECDH over NIST P-256
*
- DEM: AES128-GCM
*
- KDF: HKDF-HMAC-SHA256 with an empty salt
*
- EC Point Format: Compressed
*
- OutputPrefixType: RAW
*
*
* Unlike other key templates that use AES-GCM, the instances of {@link HybridDecrypt}
* generated by this key template has no limitation on Android KitKat (API level 19). They might
* not work in older versions though.
*/
public static final EciesParameters
ECIES_P256_HKDF_HMAC_SHA256_AES128_GCM_COMPRESSED_WITHOUT_PREFIX =
exceptionIsBug(
() ->
EciesParameters.builder()
.setCurveType(EciesParameters.CurveType.NIST_P256)
.setHashType(EciesParameters.HashType.SHA256)
.setNistCurvePointFormat(EciesParameters.PointFormat.COMPRESSED)
.setVariant(EciesParameters.Variant.NO_PREFIX)
.setDemParameters(
AesGcmParameters.builder()
.setIvSizeBytes(12)
.setKeySizeBytes(16)
.setTagSizeBytes(16)
.setVariant(AesGcmParameters.Variant.NO_PREFIX)
.build())
.build());
/**
* A {@link KeyTemplate} that generates new instances of {@link
* com.google.crypto.tink.proto.EciesAeadHkdfPrivateKey} with the following parameters:
*
*
* - KEM: ECDH over NIST P-256
*
- DEM: AES128-CTR-HMAC-SHA256 with the following parameters
*
* - AES key size: 16 bytes
*
- AES CTR IV size: 16 bytes
*
- HMAC key size: 32 bytes
*
- HMAC tag size: 16 bytes
*
* - KDF: HKDF-HMAC-SHA256 with an empty salt
*
*/
public static final EciesParameters ECIES_P256_HKDF_HMAC_SHA256_AES128_CTR_HMAC_SHA256 =
exceptionIsBug(
() ->
EciesParameters.builder()
.setCurveType(EciesParameters.CurveType.NIST_P256)
.setHashType(EciesParameters.HashType.SHA256)
.setNistCurvePointFormat(EciesParameters.PointFormat.UNCOMPRESSED)
.setVariant(EciesParameters.Variant.TINK)
.setDemParameters(
AesCtrHmacAeadParameters.builder()
.setAesKeySizeBytes(16)
.setHmacKeySizeBytes(32)
.setTagSizeBytes(16)
.setIvSizeBytes(16)
.setHashType(AesCtrHmacAeadParameters.HashType.SHA256)
.setVariant(AesCtrHmacAeadParameters.Variant.NO_PREFIX)
.build())
.build());
private PredefinedHybridParameters() {}
}