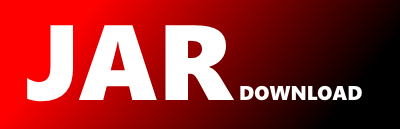
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tink Show documentation
Show all versions of tink Show documentation
Tink is a small cryptographic library that provides a safe, simple, agile and fast way to accomplish some common cryptographic tasks.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: proto/jwt_rsa_ssa_pkcs1.proto
// Protobuf Java Version: 4.28.2
package com.google.crypto.tink.proto;
/**
*
* key_type: type.googleapis.com/google.crypto.tink.JwtRsaSsaPkcs1PublicKey
*
*
* Protobuf type {@code google.crypto.tink.JwtRsaSsaPkcs1PublicKey}
*/
public final class JwtRsaSsaPkcs1PublicKey extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.JwtRsaSsaPkcs1PublicKey)
JwtRsaSsaPkcs1PublicKeyOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
JwtRsaSsaPkcs1PublicKey.class.getName());
}
// Use JwtRsaSsaPkcs1PublicKey.newBuilder() to construct.
private JwtRsaSsaPkcs1PublicKey(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private JwtRsaSsaPkcs1PublicKey() {
algorithm_ = 0;
n_ = com.google.protobuf.ByteString.EMPTY;
e_ = com.google.protobuf.ByteString.EMPTY;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.class, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.Builder.class);
}
public interface CustomKidOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid)
com.google.protobuf.MessageOrBuilder {
/**
* string value = 1;
* @return The value.
*/
java.lang.String getValue();
/**
* string value = 1;
* @return The bytes for value.
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
* Optional, custom kid header value to be used with "RAW" keys.
* "TINK" keys with this value set will be rejected.
*
*
* Protobuf type {@code google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid}
*/
public static final class CustomKid extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid)
CustomKidOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
CustomKid.class.getName());
}
// Use CustomKid.newBuilder() to construct.
private CustomKid(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CustomKid() {
value_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_CustomKid_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_CustomKid_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.class, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object value_ = "";
/**
* string value = 1;
* @return The value.
*/
@java.lang.Override
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
}
}
/**
* string value = 1;
* @return The bytes for value.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(value_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(value_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid other = (com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid) obj;
if (!getValue()
.equals(other.getValue())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Optional, custom kid header value to be used with "RAW" keys.
* "TINK" keys with this value set will be rejected.
*
*
* Protobuf type {@code google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid)
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKidOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_CustomKid_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_CustomKid_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.class, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder.class);
}
// Construct using com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
value_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_CustomKid_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid getDefaultInstanceForType() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid build() {
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid buildPartial() {
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid result = new com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.value_ = value_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid) {
return mergeFrom((com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid other) {
if (other == com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance()) return this;
if (!other.getValue().isEmpty()) {
value_ = other.value_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
value_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object value_ = "";
/**
* string value = 1;
* @return The value.
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string value = 1;
* @return The bytes for value.
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string value = 1;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string value = 1;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string value = 1;
* @param value The bytes for value to set.
* @return This builder for chaining.
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
value_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid)
private static final com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid();
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CustomKid parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int VERSION_FIELD_NUMBER = 1;
private int version_ = 0;
/**
* uint32 version = 1;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
public static final int ALGORITHM_FIELD_NUMBER = 2;
private int algorithm_ = 0;
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @return The enum numeric value on the wire for algorithm.
*/
@java.lang.Override public int getAlgorithmValue() {
return algorithm_;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @return The algorithm.
*/
@java.lang.Override public com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm getAlgorithm() {
com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm result = com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm.forNumber(algorithm_);
return result == null ? com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm.UNRECOGNIZED : result;
}
public static final int N_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString n_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Modulus.
* Unsigned big integer in big-endian representation.
*
*
* bytes n = 3;
* @return The n.
*/
@java.lang.Override
public com.google.protobuf.ByteString getN() {
return n_;
}
public static final int E_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString e_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Public exponent.
* Unsigned big integer in big-endian representation.
*
*
* bytes e = 4;
* @return The e.
*/
@java.lang.Override
public com.google.protobuf.ByteString getE() {
return e_;
}
public static final int CUSTOM_KID_FIELD_NUMBER = 5;
private com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid customKid_;
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
* @return Whether the customKid field is set.
*/
@java.lang.Override
public boolean hasCustomKid() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
* @return The customKid.
*/
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid getCustomKid() {
return customKid_ == null ? com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance() : customKid_;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKidOrBuilder getCustomKidOrBuilder() {
return customKid_ == null ? com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance() : customKid_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (version_ != 0) {
output.writeUInt32(1, version_);
}
if (algorithm_ != com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm.RS_UNKNOWN.getNumber()) {
output.writeEnum(2, algorithm_);
}
if (!n_.isEmpty()) {
output.writeBytes(3, n_);
}
if (!e_.isEmpty()) {
output.writeBytes(4, e_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(5, getCustomKid());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, version_);
}
if (algorithm_ != com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm.RS_UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, algorithm_);
}
if (!n_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, n_);
}
if (!e_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, e_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getCustomKid());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey)) {
return super.equals(obj);
}
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey other = (com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey) obj;
if (getVersion()
!= other.getVersion()) return false;
if (algorithm_ != other.algorithm_) return false;
if (!getN()
.equals(other.getN())) return false;
if (!getE()
.equals(other.getE())) return false;
if (hasCustomKid() != other.hasCustomKid()) return false;
if (hasCustomKid()) {
if (!getCustomKid()
.equals(other.getCustomKid())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
hash = (37 * hash) + ALGORITHM_FIELD_NUMBER;
hash = (53 * hash) + algorithm_;
hash = (37 * hash) + N_FIELD_NUMBER;
hash = (53 * hash) + getN().hashCode();
hash = (37 * hash) + E_FIELD_NUMBER;
hash = (53 * hash) + getE().hashCode();
if (hasCustomKid()) {
hash = (37 * hash) + CUSTOM_KID_FIELD_NUMBER;
hash = (53 * hash) + getCustomKid().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* key_type: type.googleapis.com/google.crypto.tink.JwtRsaSsaPkcs1PublicKey
*
*
* Protobuf type {@code google.crypto.tink.JwtRsaSsaPkcs1PublicKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:google.crypto.tink.JwtRsaSsaPkcs1PublicKey)
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.class, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.Builder.class);
}
// Construct using com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getCustomKidFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
version_ = 0;
algorithm_ = 0;
n_ = com.google.protobuf.ByteString.EMPTY;
e_ = com.google.protobuf.ByteString.EMPTY;
customKid_ = null;
if (customKidBuilder_ != null) {
customKidBuilder_.dispose();
customKidBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1.internal_static_google_crypto_tink_JwtRsaSsaPkcs1PublicKey_descriptor;
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey getDefaultInstanceForType() {
return com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.getDefaultInstance();
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey build() {
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey buildPartial() {
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey result = new com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.version_ = version_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.algorithm_ = algorithm_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.n_ = n_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.e_ = e_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000010) != 0)) {
result.customKid_ = customKidBuilder_ == null
? customKid_
: customKidBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey) {
return mergeFrom((com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey other) {
if (other == com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.getDefaultInstance()) return this;
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
if (other.algorithm_ != 0) {
setAlgorithmValue(other.getAlgorithmValue());
}
if (other.getN() != com.google.protobuf.ByteString.EMPTY) {
setN(other.getN());
}
if (other.getE() != com.google.protobuf.ByteString.EMPTY) {
setE(other.getE());
}
if (other.hasCustomKid()) {
mergeCustomKid(other.getCustomKid());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
version_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
algorithm_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
n_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
e_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getCustomKidFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int version_ ;
/**
* uint32 version = 1;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* uint32 version = 1;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
version_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* uint32 version = 1;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000001);
version_ = 0;
onChanged();
return this;
}
private int algorithm_ = 0;
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @return The enum numeric value on the wire for algorithm.
*/
@java.lang.Override public int getAlgorithmValue() {
return algorithm_;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @param value The enum numeric value on the wire for algorithm to set.
* @return This builder for chaining.
*/
public Builder setAlgorithmValue(int value) {
algorithm_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @return The algorithm.
*/
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm getAlgorithm() {
com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm result = com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm.forNumber(algorithm_);
return result == null ? com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm.UNRECOGNIZED : result;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @param value The algorithm to set.
* @return This builder for chaining.
*/
public Builder setAlgorithm(com.google.crypto.tink.proto.JwtRsaSsaPkcs1Algorithm value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
algorithm_ = value.getNumber();
onChanged();
return this;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1Algorithm algorithm = 2;
* @return This builder for chaining.
*/
public Builder clearAlgorithm() {
bitField0_ = (bitField0_ & ~0x00000002);
algorithm_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString n_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Modulus.
* Unsigned big integer in big-endian representation.
*
*
* bytes n = 3;
* @return The n.
*/
@java.lang.Override
public com.google.protobuf.ByteString getN() {
return n_;
}
/**
*
* Modulus.
* Unsigned big integer in big-endian representation.
*
*
* bytes n = 3;
* @param value The n to set.
* @return This builder for chaining.
*/
public Builder setN(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
n_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Modulus.
* Unsigned big integer in big-endian representation.
*
*
* bytes n = 3;
* @return This builder for chaining.
*/
public Builder clearN() {
bitField0_ = (bitField0_ & ~0x00000004);
n_ = getDefaultInstance().getN();
onChanged();
return this;
}
private com.google.protobuf.ByteString e_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Public exponent.
* Unsigned big integer in big-endian representation.
*
*
* bytes e = 4;
* @return The e.
*/
@java.lang.Override
public com.google.protobuf.ByteString getE() {
return e_;
}
/**
*
* Public exponent.
* Unsigned big integer in big-endian representation.
*
*
* bytes e = 4;
* @param value The e to set.
* @return This builder for chaining.
*/
public Builder setE(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
e_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Public exponent.
* Unsigned big integer in big-endian representation.
*
*
* bytes e = 4;
* @return This builder for chaining.
*/
public Builder clearE() {
bitField0_ = (bitField0_ & ~0x00000008);
e_ = getDefaultInstance().getE();
onChanged();
return this;
}
private com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid customKid_;
private com.google.protobuf.SingleFieldBuilder<
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKidOrBuilder> customKidBuilder_;
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
* @return Whether the customKid field is set.
*/
public boolean hasCustomKid() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
* @return The customKid.
*/
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid getCustomKid() {
if (customKidBuilder_ == null) {
return customKid_ == null ? com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance() : customKid_;
} else {
return customKidBuilder_.getMessage();
}
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
public Builder setCustomKid(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid value) {
if (customKidBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
customKid_ = value;
} else {
customKidBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
public Builder setCustomKid(
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder builderForValue) {
if (customKidBuilder_ == null) {
customKid_ = builderForValue.build();
} else {
customKidBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
public Builder mergeCustomKid(com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid value) {
if (customKidBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
customKid_ != null &&
customKid_ != com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance()) {
getCustomKidBuilder().mergeFrom(value);
} else {
customKid_ = value;
}
} else {
customKidBuilder_.mergeFrom(value);
}
if (customKid_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
public Builder clearCustomKid() {
bitField0_ = (bitField0_ & ~0x00000010);
customKid_ = null;
if (customKidBuilder_ != null) {
customKidBuilder_.dispose();
customKidBuilder_ = null;
}
onChanged();
return this;
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder getCustomKidBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getCustomKidFieldBuilder().getBuilder();
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKidOrBuilder getCustomKidOrBuilder() {
if (customKidBuilder_ != null) {
return customKidBuilder_.getMessageOrBuilder();
} else {
return customKid_ == null ?
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.getDefaultInstance() : customKid_;
}
}
/**
* .google.crypto.tink.JwtRsaSsaPkcs1PublicKey.CustomKid custom_kid = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKidOrBuilder>
getCustomKidFieldBuilder() {
if (customKidBuilder_ == null) {
customKidBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKid.Builder, com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey.CustomKidOrBuilder>(
getCustomKid(),
getParentForChildren(),
isClean());
customKid_ = null;
}
return customKidBuilder_;
}
// @@protoc_insertion_point(builder_scope:google.crypto.tink.JwtRsaSsaPkcs1PublicKey)
}
// @@protoc_insertion_point(class_scope:google.crypto.tink.JwtRsaSsaPkcs1PublicKey)
private static final com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey();
}
public static com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JwtRsaSsaPkcs1PublicKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.crypto.tink.proto.JwtRsaSsaPkcs1PublicKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy